题目:利用多线程与有名管道技术,实现两个进程之间发送即时消息,实现聊天功能
思路:关键在于建立两个有名管道,利用多线程技术,进程A中线程1向管道A写数据,进程B中线程2从管道A读数据,进程A线程2从管道B中读数据,进程B中线程1往管道B中写数据。
//利用多线程与有名管道技术,实现两个进程之间发送即时消息,实现聊天功能
//进程A
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <errno.h>
#include <pthread.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
//从管道1中读数据
void * Threadread(void * arg)
{
//open the fifo
int fd = 0;
//open the fifo in read and write mode--以读写方式打开管道
fd = open("/home/test/1/fifo1", O_RDONLY);
if (fd == -1)
{
printf("open the fifo failed ! error message :%s\n", strerror(errno));
return NULL;
}
char buf[100] = { 0 };
//从管道中读数据
while (read(fd, buf, sizeof(buf)) > 0)
{
printf("%s", buf);
memset(buf, 0, sizeof(buf));
}
close(fd);
return NULL;
}
//往管道2中写数据
void * ThreadWrite(void * arg)
{
//open the fifo
int fd = 0;
//open the fifo in read and write mode--以读写方式打开管道
fd = open("/home/test/1/fifo2", O_WRONLY);
if (fd == -1)
{
printf("open the fifo failed ! error message :%s\n", strerror(errno));
return NULL;
}
char buf[100] = { 0 };
while (1)
{
//往标准输入上读数据
read(STDIN_FILENO, buf, sizeof(buf));
//在管道中写数据
write(fd, buf, strlen(buf));
memset(buf, 0, sizeof(buf));
}
close(fd);
return NULL;
}
int main(int arg, char * args[])
{
//两线程共用一个文件描述符
pthread_t thr1, thr2;
//创建读数据线程
if (pthread_create(&thr1, NULL, Threadread, NULL) != 0)
{
printf("create thread is failed ! error mesage :%s\n", strerror(errno));
return -1;
}
//创建写数据线程
if (pthread_create(&thr2, NULL, ThreadWrite, NULL) != 0)
{
printf("create thread is failed ! error mesage :%s\n", strerror(errno));
return -1;
}
//等待两线程都返回 关闭文件描述符
pthread_join(thr1, NULL);
pthread_join(thr2, NULL);
return 0;
}
.SUFFIXES:.c .o
CC=gcc
SRCS1=tec01.c
SRCS2=tec02.c
OBJS1=$(SRCS1:.c=.o)
OBJS2=$(SRCS2:.c=.o)
EXEC1=a
EXEC2=b
start:$(OBJS1) $(OBJS2)
$(CC) -lpthread -o $(EXEC1) $(OBJS1)
$(CC) -lpthread -o $(EXEC2) $(OBJS2)
@echo "^_^-----OK------^_^"
.c.o:
$(CC) -Wall -g -o $@ -c $<
clean:
rm -f $(OBJS1)
rm -f $(OBJS2)
rm -f $(EXEC1)
rm -f $(EXEC2)
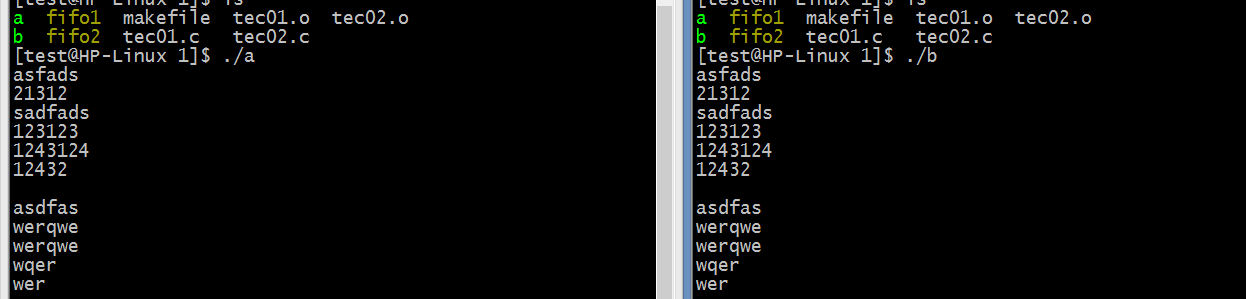