20202322 2021-2022-1 《数据结构与面向对象程序设计》实验三 实验报告
# 20202322 2021-2022-1 《数据结构与面向对象程序设计》实验二报告
课程:《程序设计与数据结构》
班级: 2023
姓名: 袁艺匀
学号:20202322
实验教师:王志强老师
实验日期:2021年9月30日
必修/选修: 必修
## 1.实验内容
下载安装并使用IDEA,完成下面实验(https://www.cnblogs.com/rocedu/p/6371315.html)。
1. 初步掌握单元测试和TDD
2. 理解并掌握面向对象三要素:封装、继承、多态
3. 初步掌握UML建模
4. 完成蓝墨云上 (1)-(5)实验。
## 2. 实验过程及结果
(1)初步掌握单元测试和TDD
a.单元测试
代码:
abstract class Data {
abstract public void DisplayValue();
}
class Integer extends Data {
int value;
Integer() {
value=100;
}
public void DisplayValue(){
System.out.println (value);
}
}
class Float extends Data {
float value;
Float() {
value=1.23456F;
}
public void DisplayValue(){
System.out.println (value);
}
}
abstract class Factory {
abstract public Data CreateDataObject();
}
class IntFactory extends Factory {
public Data CreateDataObject(){
return new Integer();
}
}
class FloatFactory extends Factory {
public Data CreateDataObject(){
return new Float();
}
}
class Document {
Data pd;
Document(Factory pf){
pd = pf.CreateDataObject();
}
public void DisplayData(){
pd.DisplayValue();
}
}
public class MyDoc {
static Document d,f;
public static void main(String[] args) {
d = new Document(new IntFactory());
d.DisplayData();
f = new Document(new FloatFactory());
f.DisplayData();
}
}
b.TDD
public class StringBufferDemo {
public static void main(String[] args) {
StringBuffer buffer = new StringBuffer(20);
buffer.append('S');
buffer.append("tringBuffer");
System.out.println(buffer.charAt(1));
System.out.println(buffer.capacity());
System.out.println(buffer.indexOf("tring12345"));
System.out.println("buffer = " + buffer.toString());
System.out.println(buffer.length());
}
}
import org.junit.Test;
import junit.framework.TestCase;
public class StringBufferDemoTest extends TestCase {
StringBuffer a = new StringBuffer("StringBuffer");
StringBuffer b = new StringBuffer("StringBufferStringBuffer");
StringBuffer c = new StringBuffer("StringBufferStringBufferStringBuffer");
@Test
public void testcharAt() throws Exception{
assertEquals('S',a.charAt(0));
assertEquals('i',b.charAt(15));
assertEquals('g',c.charAt(29));
}
@Test
public void testcapacity() throws Exception{
assertEquals(28,a.capacity());
assertEquals(40,b.capacity());
assertEquals(52,c.capacity());
}
@Test
public void testlength() throws Exception{
assertEquals(12,a.length());
assertEquals(24,b.length());
assertEquals(36,c.length());
}
@Test
public void testindexOf() throws Exception{
assertEquals(0,a.indexOf("Str"));
assertEquals(5,a.indexOf("gBu"));
assertEquals(4,a.indexOf("ng"));
}
@Test
public void testtoString() throws Exception{
assertEquals("Stringbuffer",a.toString());
assertEquals("StringBufferStringBuffer",b.toString());
assertEquals("StringBufferStringBufferStringBuffer",c.toString());
}
}
(2)理解和掌握封装、继承、多态
a.封装:封装,即隐藏对象的属性和实现细节,仅对外公开接口,控制在程序中属性的读和修改的访问级别;将抽象得到的数据和行为(或功能)相结合,形成一个有机的整体,也就是将数据与操作数据的源代码进行有机的结合,形成“类”,其中数据和函数都是类的成员。
public class Complex {
double RealPart;
double ImagePart;
void setRealPart(double r){
RealPart=r;
}
void setImagePart(double i){
ImagePart=i;
}
double getRealPart(Complex a){
return a.RealPart;
}
double getImagePart(Complex a){
return a.ImagePart;
}
public Complex(double r,double i){
RealPart=r;
ImagePart=i;
}
public String toString(){
String result = new String();
if (ImagePart>0)
result = RealPart+"+"+ImagePart+"i";
if (ImagePart==0)
result = RealPart+"";
if (ImagePart<0)
result = RealPart+"-"+ImagePart*(-1)+"i";
return result;
}
public Complex ComplexAdd(Complex a){
return new Complex(RealPart+a.RealPart,ImagePart+a.ImagePart);
}
public Complex ComplexSub(Complex a){
return new Complex(RealPart-a.RealPart,ImagePart-a.ImagePart);
}
public Complex ComplexMulti(Complex a){
return new Complex(RealPart*a.RealPart-ImagePart*a.ImagePart,RealPart*a.ImagePart+ImagePart*a.RealPart);
}
public Complex ComplexDiv(Complex a){
double d = Math.sqrt(a.RealPart * a.RealPart) + Math.sqrt(a.ImagePart * a.ImagePart);
return new Complex((RealPart*a.RealPart+ImagePart*a.ImagePart)/d,(ImagePart*a.RealPart-RealPart*a.ImagePart)/d);
}
public static void main(String[] a) {
Complex b = new Complex(2, 6);
Complex c = new Complex(1, -3);
System.out.println("("+b + ")+(" + c + ")=" + b.ComplexAdd(c));
System.out.println("("+b + ")-(" + c + ")=" + b.ComplexSub(c));
System.out.println("("+b + ")*(" + c + ")=" + b.ComplexMulti(c));
System.out.println("("+b + ")/(" + c + ")=" + b.ComplexDiv(c));
}
}
b.继承:是从现有类派生新类的过程。
abstract class Data {
abstract public void DisplayValue();
}
class Integer extends Data {
int value;
Integer() {
value=100;
}
public void DisplayValue(){
System.out.println (value);
}
}
class Float extends Data {
float value;
Float() {
value=1.23456F;
}
public void DisplayValue(){
System.out.println (value);
}
}
abstract class Factory {
abstract public Data CreateDataObject();
}
class IntFactory extends Factory {
public Data CreateDataObject(){
return new Integer();
}
}
class FloatFactory extends Factory {
public Data CreateDataObject(){
return new Float();
}
}
class Document {
Data pd;
Document(Factory pf){
pd = pf.CreateDataObject();
}
public void DisplayData(){
pd.DisplayValue();
}
}
public class MyDoc {
static Document d,f;
public static void main(String[] args) {
d = new Document(new IntFactory());
d.DisplayData();
f = new Document(new FloatFactory());
f.DisplayData();
}
}
c.多态:多种形态,使用父类的对象指向子类的对象,调用子类的方法。
//多态
animal2023 = dog2023;
animal2023.shout();
animal2023.feed("bone or shit");
animal2023=daju2023;
animal2023.shout();
animal2023.feed("麻辣香锅");
//daju2023=(Cat2023) dog2023; 子类间不能进行操作
(3)初步掌握UML建模
(4)完成蓝墨云上 (1)-(5)实验。
a.参考 http://www.cnblogs.com/rocedu/p/6371315.html#SECUNITTEST 参考http://www.cnblogs.com/rocedu/p/6736847.html 提交最后三个测试用例都通过的截图,截图上要有画图加水印,输入自己的学号。
b.参考 积极主动敲代码,使用JUnit学习Java (http://www.cnblogs.com/rocedu/p/4837092.html)
参考http://www.cnblogs.com/rocedu/p/6736847.html
以 TDD的方式研究学习StringBuffer,提交你的单元测试用例和测试通过的截图,截图要加上学号水印。
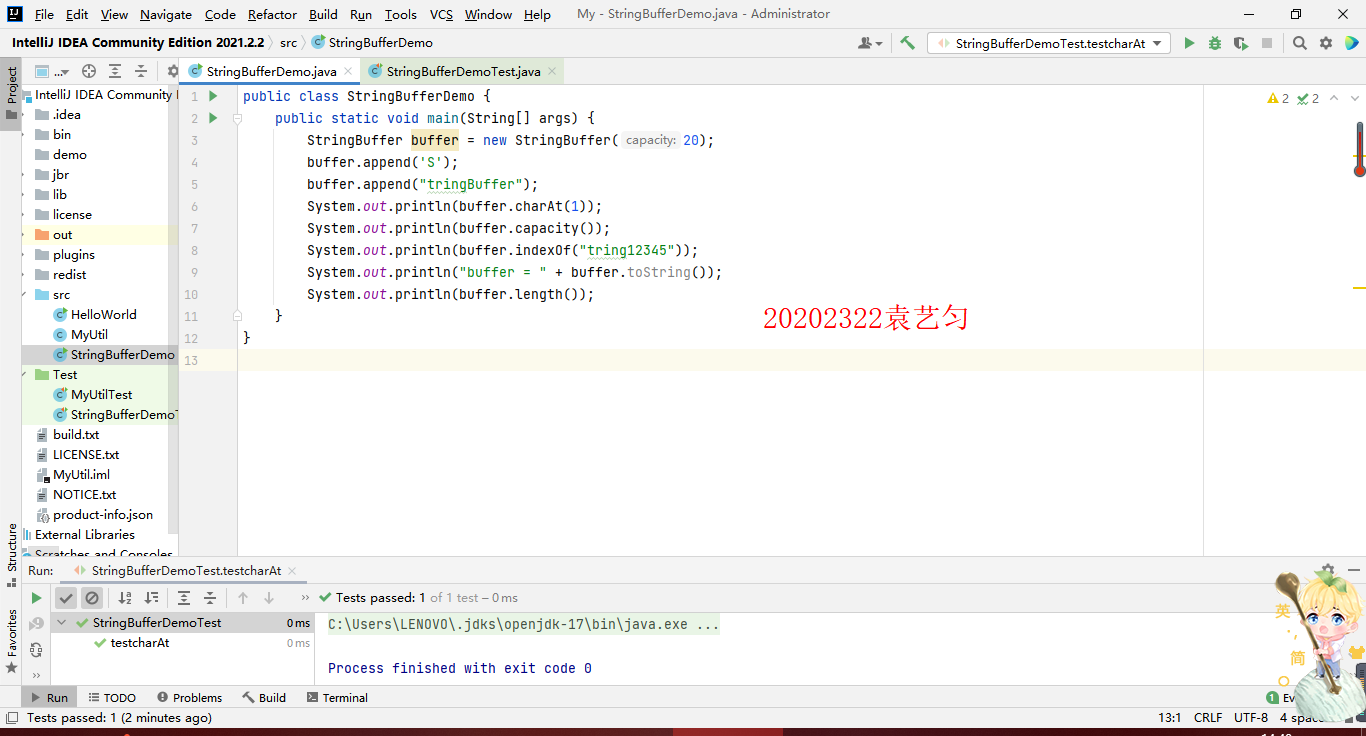
c.实验三 Java面向对象程序设计(http://www.cnblogs.com/rocedu/p/4472842.html)
参考http://www.cnblogs.com/rocedu/p/6736847.html
对设计模式示例进行扩充,体会OCP原则和DIP原则的应用,初步理解设计模式
用自己的学号%6进行取余运算,根据结果进行代码扩充:
0:让系统支持Byte类,并在MyDoc类中添加测试代码表明添加正确,提交测试代码和运行结的截图,加上学号水印
1:让系统支持Short类,并在MyDoc类中添加测试代码表明添加正确,提交测试代码和运行结的截图,加上学号水印
2:让系统支持Boolean类,并在MyDoc类中添加测试代码表明添加正确,提交测试代码和运行结的截图,加上学号水印
3:让系统支持Long类,并在MyDoc类中添加测试代码表明添加正确,提交测试代码和运行结的截图,加上学号水印
4:让系统支持Float类,并在MyDoc类中添加测试代码表明添加正确,提交测试代码和运行结的截图,加上学号水印
5:让系统支持Double类,并在MyDoc类中添加测试代码表明添加正确,提交测试代码和运行结的截图,加上学号
d.任务:以TDD的方式开发一个复数类Complex,要求如下:
// 定义属性并生成getter,setter
double RealPart;
double ImagePart;
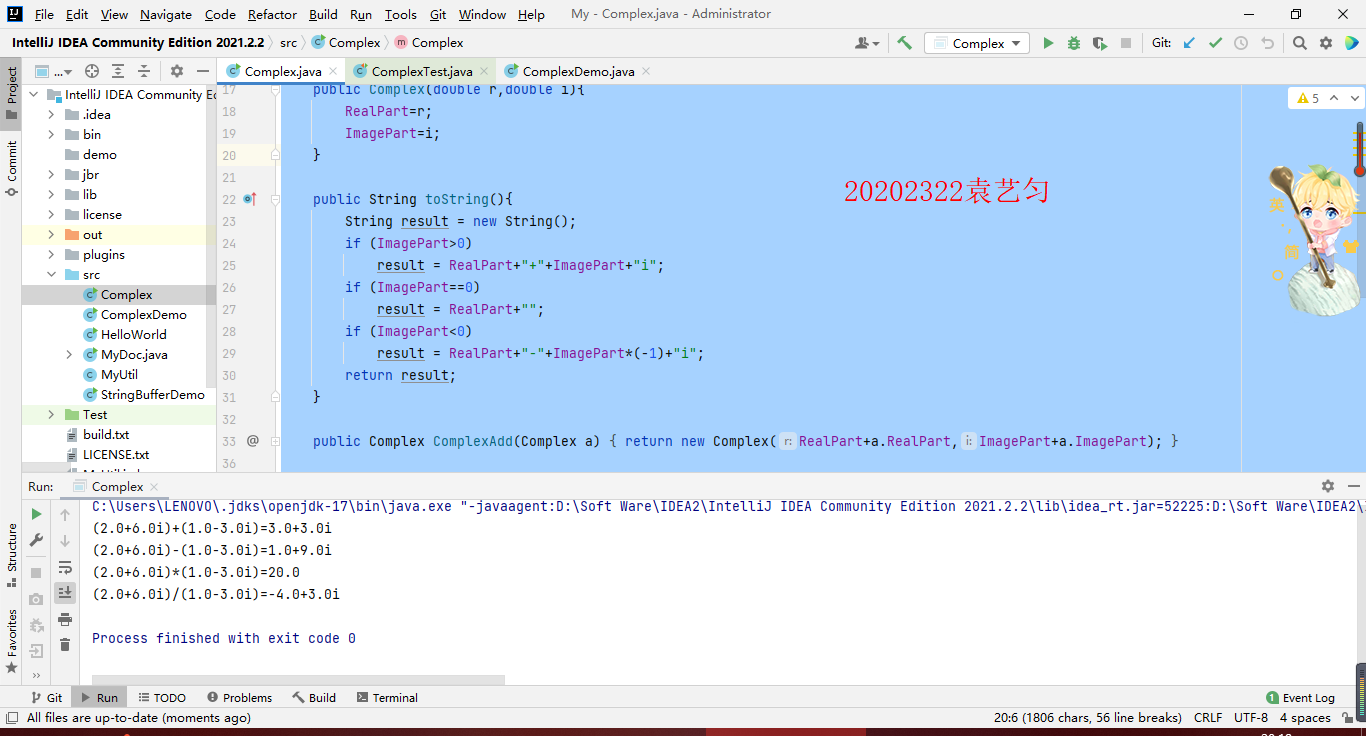
e.对实验二中的代码进行建模,发类图的截图,加上学号水印。
类图中只少两个类。
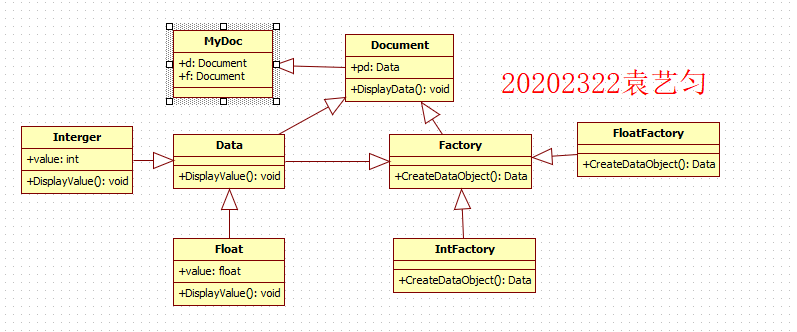
## 3. 实验过程中遇到的问题和解决过程
-问题1 在此处少了一个后括号,后面还有一张也是相同地方没有后括号。
-问题2 第九行有无toString在表达上都是不影响的
-问题3 找不到模块
解决:在运行时选择Edit进行编辑
1.从上方工具栏里点击Run
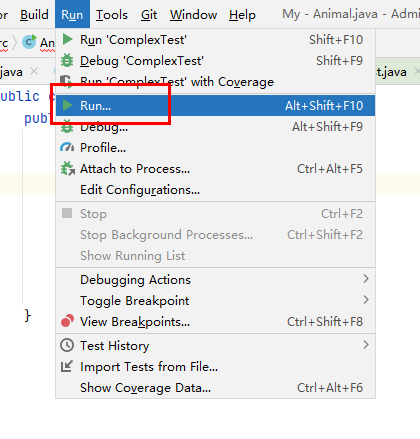
2.点击Edit
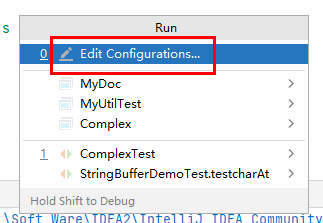
## 参考资料
- [《Java程序设计与数据结构教程(第二版)》](https://book.douban.com/subject/26851579/)
- [《Java程序设计与数据结构教程(第二版)》学习指导](http://www.cnblogs.com/rocedu/p/5182332.html)
- 本地项目上传到码云(gitee)远程仓库(图文详解) - 星空流年 - 博客园 (cnblogs.com)