《图形学》实验二:分形树
实验平台:
VC++6.0,OpenGL
实验内容:
先按某一方向画一条直线,然后在此线段上找到一系列节点,在每一节点处向左右偏转60各画一条分枝。节点位置和节点处所画分枝的长度的比值各按0.618分割。
编程要点:
1.递归调用
grow(x,y,length,fai)
2.结束条件:最短树枝
3.树枝数<10
实验结果:
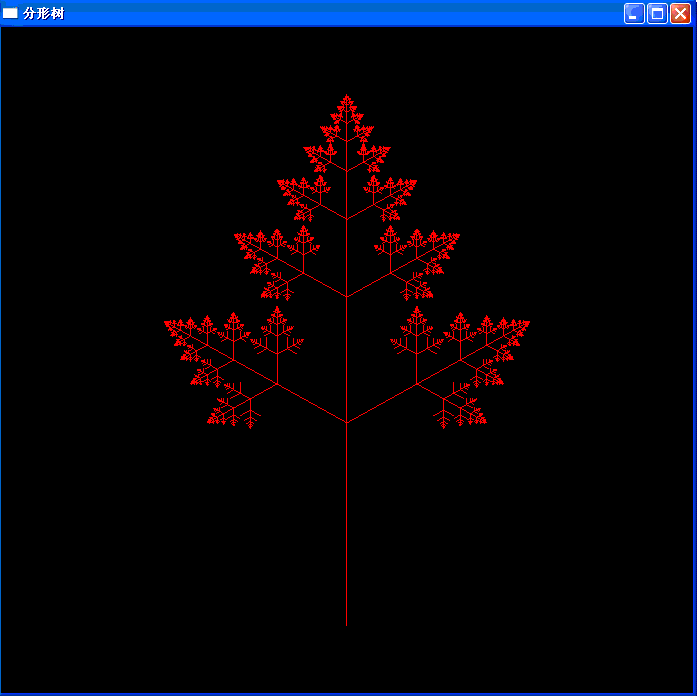
代码:
1 #include <windows.h>
2 #include <gl/gl.h>
3 #include <stdio.h>
4
5 #define PI acos(-1.0)
6
7 //存储n边形的n个点坐标
8 float px[1000] = {0};
9 float py[1000] = {0};
10 int n; //n边形
11
12 void GetAllPoint(int n) //得到n边形n个点的坐标
13 {
14 float x,y;
15 float r = 0.8; //半径
16 float si = 2*PI/n; //360°/n
17 float angle = si/2; //开始度数
18 int pnum = 0;
19 while(angle<=2*PI){
20 x = r*cos(angle);
21 y = r*sin(angle);
22 px[pnum] = x;
23 py[pnum++] = y;
24 angle = angle+si;
25 }
26 }
27
28 void ShowPic1(int n) //画出n边形的外边框
29 {
30 int i;
31 float x,y;
32 for(i=0;i<n;i++){
33 x = px[i];
34 y = py[i];
35 glVertex2f(x,y);
36 }
37 }
38
39 void ShowPic2(int n) //画出n边形的中间连线
40 {
41 int i,j;
42 float x0,y0,x,y;
43 for(i=0;i<n;i++){
44 x0 = px[i];
45 y0 = py[i];
46 for(j=0;j<n;j++){
47 if(i==j)
48 continue;
49 glVertex2f(x0,y0);
50 x = px[j];
51 y = py[j];
52 glVertex2f(x,y);
53 }
54 }
55 }
56
57 LRESULT CALLBACK WindowProc(HWND, UINT, WPARAM, LPARAM);
58 void EnableOpenGL(HWND hwnd, HDC*, HGLRC*);
59 void DisableOpenGL(HWND, HDC, HGLRC);
60
61 int WINAPI WinMain(HINSTANCE hInstance,
62 HINSTANCE hPrevInstance,
63 LPSTR lpCmdLine,
64 int nCmdShow)
65 {
66 WNDCLASSEX wcex;
67 HWND hwnd;
68 HDC hDC;
69 HGLRC hRC;
70 MSG msg;
71 BOOL bQuit = FALSE;
72 float theta = 0.0f;
73
74 printf("请输入n (n边形):\n");
75 scanf("%d",&n);
76
77 GetAllPoint(n); //获取多边形全部的点
78
79 /* register window class */
80 wcex.cbSize = sizeof(WNDCLASSEX);
81 wcex.style = CS_OWNDC;
82 wcex.lpfnWndProc = WindowProc;
83 wcex.cbClsExtra = 0;
84 wcex.cbWndExtra = 0;
85 wcex.hInstance = hInstance;
86 wcex.hIcon = LoadIcon(NULL, IDI_APPLICATION);
87 wcex.hCursor = LoadCursor(NULL, IDC_ARROW);
88 wcex.hbrBackground = (HBRUSH)GetStockObject(BLACK_BRUSH);
89 wcex.lpszMenuName = NULL;
90 wcex.lpszClassName = "GLSample";
91 wcex.hIconSm = LoadIcon(NULL, IDI_APPLICATION);;
92
93
94 if (!RegisterClassEx(&wcex))
95 return 0;
96
97 /* create main window */
98 hwnd = CreateWindowEx(0,
99 "GLSample",
100 "钻石图案",
101 WS_OVERLAPPEDWINDOW,
102 CW_USEDEFAULT,
103 CW_USEDEFAULT,
104 700,
105 700,
106 NULL,
107 NULL,
108 hInstance,
109 NULL);
110
111 ShowWindow(hwnd, nCmdShow);
112
113 /* enable OpenGL for the window */
114 EnableOpenGL(hwnd, &hDC, &hRC);
115
116 /* program main loop */
117 while (!bQuit)
118 {
119 /* check for messages */
120 if (PeekMessage(&msg, NULL, 0, 0, PM_REMOVE))
121 {
122 /* handle or dispatch messages */
123 if (msg.message == WM_QUIT)
124 {
125 bQuit = TRUE;
126 }
127 else
128 {
129 TranslateMessage(&msg);
130 DispatchMessage(&msg);
131 }
132 }
133 else
134 {
135 /* OpenGL animation code goes here */
136
137 glClearColor(0.0f, 0.0f, 0.0f, 0.0f);
138 glClear(GL_COLOR_BUFFER_BIT);
139
140 //glPushMatrix();
141 //glRotatef(theta, 0.0f, 0.0f, 1.0f);
142
143 glBegin(GL_LINE_STRIP);
144 glColor3f(1.0f, 0.0f, 0.0f); //设置画笔颜色
145 ShowPic1(n); //输出n边形的外边框
146 glEnd();
147
148 //printf("%d\n",pnum);
149
150 glBegin(GL_LINES);
151 ShowPic2(n); //画出n边形的中间连线
152 glEnd();
153
154 glPopMatrix();
155
156 SwapBuffers(hDC);
157
158 theta += 1.0f;
159 Sleep (1);
160 }
161 }
162
163 /* shutdown OpenGL */
164 DisableOpenGL(hwnd, hDC, hRC);
165
166 /* destroy the window explicitly */
167 DestroyWindow(hwnd);
168
169 return msg.wParam;
170 }
171
172 LRESULT CALLBACK WindowProc(HWND hwnd, UINT uMsg, WPARAM wParam, LPARAM lParam)
173 {
174 switch (uMsg)
175 {
176 case WM_CLOSE:
177 PostQuitMessage(0);
178 break;
179
180 case WM_DESTROY:
181 return 0;
182
183 case WM_KEYDOWN:
184 {
185 switch (wParam)
186 {
187 case VK_ESCAPE:
188 PostQuitMessage(0);
189 break;
190 }
191 }
192 break;
193
194 default:
195 return DefWindowProc(hwnd, uMsg, wParam, lParam);
196 }
197
198 return 0;
199 }
200
201 void EnableOpenGL(HWND hwnd, HDC* hDC, HGLRC* hRC)
202 {
203 PIXELFORMATDESCRIPTOR pfd;
204
205 int iFormat;
206
207 /* get the device context (DC) */
208 *hDC = GetDC(hwnd);
209
210 /* set the pixel format for the DC */
211 ZeroMemory(&pfd, sizeof(pfd));
212
213 pfd.nSize = sizeof(pfd);
214 pfd.nVersion = 1;
215 pfd.dwFlags = PFD_DRAW_TO_WINDOW |
216 PFD_SUPPORT_OPENGL | PFD_DOUBLEBUFFER;
217 pfd.iPixelType = PFD_TYPE_RGBA;
218 pfd.cColorBits = 24;
219 pfd.cDepthBits = 16;
220 pfd.iLayerType = PFD_MAIN_PLANE;
221
222 iFormat = ChoosePixelFormat(*hDC, &pfd);
223
224 SetPixelFormat(*hDC, iFormat, &pfd);
225
226 /* create and enable the render context (RC) */
227 *hRC = wglCreateContext(*hDC);
228
229 wglMakeCurrent(*hDC, *hRC);
230 }
231
232 void DisableOpenGL (HWND hwnd, HDC hDC, HGLRC hRC)
233 {
234 wglMakeCurrent(NULL, NULL);
235 wglDeleteContext(hRC);
236 ReleaseDC(hwnd, hDC);
237 }
Freecode : www.cnblogs.com/yym2013