POJ2195 Going Home【KM最小匹配】
题目链接:http://poj.org/problem?id=2195
Going Home
Time Limit: 1000MS | Memory Limit: 65536K | |
Total Submissions:27287 | Accepted: 13601 |
Description
On a grid map there are n little men and n houses. In each unit time, every little man can move one unit step, either horizontally, or vertically, to an adjacent point. For each little man, you need to pay a $1 travel fee for every step he moves, until he enters a house. The task is complicated with the restriction that each house can accommodate only one little man.
Your task is to compute the minimum amount of money you need to pay in order to send these n little men into those n different houses. The input is a map of the scenario, a '.' means an empty space, an 'H' represents a house on that point, and am 'm' indicates there is a little man on that point.
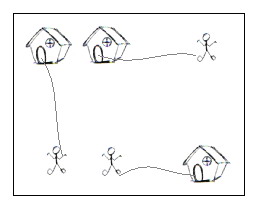
You can think of each point on the grid map as a quite large square, so it can hold n little men at the same time; also, it is okay if a little man steps on a grid with a house without entering that house.
Your task is to compute the minimum amount of money you need to pay in order to send these n little men into those n different houses. The input is a map of the scenario, a '.' means an empty space, an 'H' represents a house on that point, and am 'm' indicates there is a little man on that point.
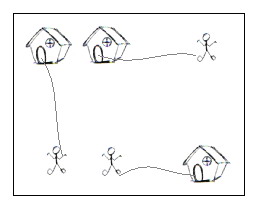
You can think of each point on the grid map as a quite large square, so it can hold n little men at the same time; also, it is okay if a little man steps on a grid with a house without entering that house.
Input
There are one or more test cases in the input. Each case starts with a line giving two integers N and M, where N is the number of rows of the map, and M is the number of columns. The rest of the input will be N lines describing the map. You may assume both N and M are between 2 and 100, inclusive. There will be the same number of 'H's and 'm's on the map; and there will be at most 100 houses. Input will terminate with 0 0 for N and M.
Output
For each test case, output one line with the single integer, which is the minimum amount, in dollars, you need to pay.
题意:在图上有相同数量的人和房子,人走一步的代价为1,求每个人都进入房子后代价和最小为多少。
思路:
1.利用KM算法求最小匹配,将人作为二分图的x部的点,房子作为y部的点,边权为走一步的代价*哈曼顿距离。需要注意的是,KM算法是求最大匹配的,求最小匹配需要将边权取负值,初始化lx[]数组时需要取 -inf,最后返回答案也要返回相反值。
2.用最小费用最大流的做法在这里 https://www.cnblogs.com/yuanweidao/p/11254863.html
代码如下:

1 #include<stdio.h> 2 #include<string.h> 3 #include<math.h> 4 #include<algorithm> 5 #define mem(a, b) memset(a, b, sizeof(a)) 6 const int inf = 0x3f3f3f3f; 7 using namespace std; 8 9 int n, m; 10 char map[110][110]; 11 int lx[110], ly[110], match[110], visx[110], visy[110], weight[110][110], slack[110]; 12 13 struct Node 14 { 15 int x, y; 16 }xx[110], yy[110]; 17 int cnt1, cnt2; 18 19 int find(int x) 20 { 21 visx[x] = 1; 22 for(int j = 1; j <= cnt2; j ++) 23 { 24 if(!visy[j]) 25 { 26 int t = lx[x] + ly[j] - weight[x][j]; 27 if(t == 0) 28 { 29 visy[j] = 1; 30 if(match[j] == -1 || find(match[j])) 31 { 32 match[j] = x; 33 return 1; 34 } 35 } 36 else if(slack[j] > t) 37 slack[j] = t; 38 } 39 } 40 return 0; 41 } 42 43 int KM() 44 { 45 mem(lx, -inf); //最小权 lx初始化为 -inf 46 mem(ly, 0), mem(match, -1); 47 for(int i = 1; i <= cnt1; i ++) 48 for(int j = 1; j <= cnt2; j ++) 49 lx[i] = max(lx[i], weight[i][j]); 50 for(int i = 1; i <= cnt1; i ++) 51 { 52 for(int j = 1; j <= cnt2; j ++) 53 slack[j] = inf; 54 while(1) 55 { 56 mem(visx, 0), mem(visy, 0); 57 if(find(i)) 58 break; 59 int d = inf; 60 for(int j = 1; j <= cnt2; j ++) 61 if(!visy[j] && d > slack[j]) 62 d = slack[j]; 63 for(int j = 1; j <= cnt2; j ++) 64 { 65 if(!visy[j]) 66 slack[j] -= d; 67 else 68 ly[j] += d; 69 } 70 for(int j = 1; j <= cnt1; j ++) 71 if(visx[j]) 72 lx[j] -= d; 73 } 74 } 75 int ans = 0; 76 for(int j = 1; j <= cnt2; j ++) 77 if(match[j] != -1) 78 ans += weight[match[j]][j]; 79 return -ans;//返回负值 80 } 81 82 int main() 83 { 84 while(scanf("%d%d", &n, &m) != EOF) 85 { 86 if(n == 0 && m == 0) 87 break; 88 getchar(); 89 cnt1 = 0, cnt2 = 0; 90 for(int i = 1; i <= n; i ++) 91 scanf("%s", map[i] + 1); 92 for(int i = 1; i <= n; i ++) 93 for(int j = 1; j <= m; j ++) 94 { 95 if(map[i][j] == 'm')//存人的点 96 xx[++ cnt1].x = i, xx[cnt1].y = j; 97 else if(map[i][j] == 'H')//存房子的点 98 yy[++ cnt2].x = i, yy[cnt2].y = j; 99 } 100 for(int i = 1; i <= cnt1; i ++) //KM求最小匹配 边权赋为 负值 101 for(int j = 1; j <= cnt2; j ++) 102 weight[i][j] = -(abs(xx[i].x - yy[j].x) + abs(xx[i].y - yy[j].y)); 103 int ans = KM(); 104 printf("%d\n", ans); 105 } 106 return 0; 107 }