第一节:MongoDB简介、基本环境搭建、基于.Net实操
一. 简介
1. 说明
MongoDB是一个基于分布式文件存储 的数据库。由C++语言编写。旨在为WEB应用提供可扩展的高性能数据存储解决方案。
MongoDB是一个介于关系数据库和非关系数据库之间的产品,是非关系数据库当中功能最丰富,最像关系数据库的。它支持的数据结构非常松散,是类似json的bson格式,因此可以存储比较复杂的数据类型。Mongo最大的特点是它支持的查询语言非常强大,其语法有点类似于面向对象的查询语言,几乎可以实现类似关系数据库单表查询的绝大部分功能,而且还支持对数据建立索引。
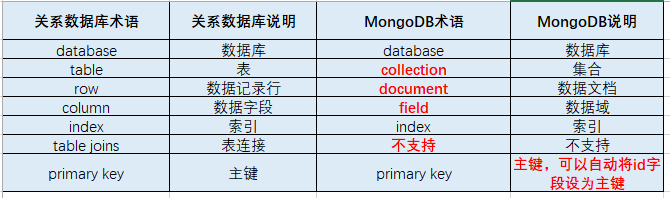
2. 相关地址
.Net 驱动程序集【MongoDB.Driver】:https://github.com/mongodb/mongo-csharp-driver
二. 环境准备
1. 安装MongoDB
【这里以windows环境下 5.0.6为例 】
下载地址:https://www.mongodb.com/try/download/community
安装后的目录如下:
会自动安装成windows服务:
配置文件:D:\Program Files\MongoDB\Server\5.0\bin\mongod.cfg (这里保持默认配置即可)
主要包括三部分:数据存储路径、日志路径、连接地址
查看代码
# mongod.conf
# for documentation of all options, see:
# http://docs.mongodb.org/manual/reference/configuration-options/
# Where and how to store data.
storage:
dbPath: D:\Program Files\MongoDB\Server\5.0\data
journal:
enabled: true
# engine:
# wiredTiger:
# where to write logging data.
systemLog:
destination: file
logAppend: true
path: D:\Program Files\MongoDB\Server\5.0\log\mongod.log
# network interfaces
net:
port: 27017
bindIp: 127.0.0.1
#processManagement:
#security:
#operationProfiling:
#replication:
#sharding:
## Enterprise-Only Options:
#auditLog:
#snmp:
2. 安装客户端 MongoDB-Compass
【这里以版本1.2.6为例】
默认连接:不使用账号密码
3. 启动MongoDB
(1). 服务的形式启动:
【net start mongodb】
【net stop mongodb】
(2). 指令的形式启动:
cmd中运行 【mongod.exe –config mongod.cfg】
或者用绝对路径 【mongod.exe –config “D:\Program Files\MongoDB\Server\5.0\bin”】
4. 启动客户端程序 MongoDB-Compass
(1). 双击
(2). 连接即可
默认连接字符串为:mongodb://localhost:27017/?readPreference=primary&appname=MongoDB%20Compass&ssl=false
或者使用可视化界面进行输入连接:
三. 实操
1. 相关程序集
【MongoDB.Driver 2.17.1】
相关实体:加上如下特性,id值自动生成。
public class ShipInfo
{
/// <summary>
/// 自动生成id
/// </summary>
[BsonId]
[BsonRepresentation(BsonType.ObjectId)]
public string id { set; get; }
public string shipCode { set; get; }
public string shipName { set; get; }
public decimal shipPrice { set; get; }
public DateTime addTime { set; get; }
public int delflag { set; get; }
}
2. 建立连接
对于db和collection,有的话直接连接,没有则创建,但必须进行相应的数据操作的时候才会创建。
public class HomeController : ControllerBase
{
private readonly IMongoCollection<ShipInfo> shipService;
public HomeController()
{
// 1. 建立MongoDB连接
var client = new MongoClient("mongodb://localhost:27017");
// 2. 获取数据库ShipDB 【若没有,则自动创建,插入数据的时候才生效】
var database = client.GetDatabase("ShipDB");
// 3. 获取表 ShipInfo【若没有,则自动创建,插入数据的时候才生效】
shipService = database.GetCollection<ShipInfo>("ShipInfo");
}
}
3. 增加
单条:InsertOne
/// <summary>
/// 插入-单条
/// </summary>
[HttpPost]
public string InsertOne()
{
ShipInfo shipInfo = new()
{
shipName = "001",
shipCode = "test1",
shipPrice = 1000,
addTime = DateTime.Now,
delflag = 0
};
shipService.InsertOne(shipInfo);
return "okok";
}
多条:InsertMany
/// <summary>
/// 插入-多条
/// </summary>
[HttpPost]
public string InsertMany()
{
List<ShipInfo> shipList = new List<ShipInfo>();
for (int i = 1; i <= 5; i++)
{
ShipInfo shipInfo = new();
shipInfo.shipName = "ypf-" + i;
shipInfo.shipCode = "000-" + i;
shipInfo.shipPrice = 1000;
shipInfo.addTime = DateTime.Now.AddDays(i);
shipInfo.delflag = 0;
shipList.Add(shipInfo);
}
shipService.InsertMany(shipList);
return "ok";
}
如下图:
4. 删除
单条:DeleteOne
/// <summary>
/// 删除-单条
/// </summary>
[HttpPost]
public string DeleteOne(string id)
{
DeleteResult result = shipService.DeleteOne<ShipInfo>(u => u.id == id);
return "ok--" + result.DeletedCount;
}
多条:DeleteMany
/// <summary>
/// 删除-多条条
/// </summary>
[HttpPost]
public string DeleteMany(string id)
{
DeleteResult result = shipService.DeleteMany<ShipInfo>(u => u.id == id);
return "ok--" + result.DeletedCount;
}
5. 修改
单条: UpdateOne
/// <summary>
/// 更新-单条 (根据id值更新)
/// </summary>
/// <param name="id">编号</param>
/// <param name="shipName">船名</param>
/// <param name="shipCode">船编码</param>
/// <returns></returns>
[HttpGet]
public string UpdateOne(string id, string shipName, string shipCode)
{
var myUpdate = Builders<ShipInfo>.Update
.Set("shipName", shipName)
.Set("shipCode", shipCode);
UpdateResult result = shipService.UpdateOne(u => u.id == id, myUpdate);
return "ok--" + result.ModifiedCount;
}
多条: UpdateMany
/// <summary>
/// 批量更新-多条 (根据shipName值更新)
/// </summary>
/// <param name="shipName">船名</param>
/// <param name="shipCode">船编码</param>
[HttpGet]
public string UpdateMany(string shipName, string shipCode)
{
var myUpdate = Builders<ShipInfo>.Update
.Set("shipCode", shipCode);
UpdateResult result = shipService.UpdateMany(u => u.shipName == shipName, myUpdate);
return "ok--" + result.ModifiedCount;
}
6. 查询
查询:Find
/// <summary>
/// 条件查询
/// (根据船名查询信息)
/// </summary>
/// <param name="shipName">船名</param>
/// <returns></returns>
[HttpPost]
public List<ShipInfo> GetByShipName(string shipName)
{
return shipService.Find(u => u.shipName == shipName).ToList();
}
分页:Skip+Limit
/// <summary>
/// 分页查询
/// </summary>
/// <param name="pageIndex">当前页码</param>
/// <param name="pageSize">每页数量</param>
/// <returns></returns>
[HttpGet]
public List<ShipInfo> GetPageList(int pageIndex = 1, int pageSize = 1)
{
return shipService.Find(u => true).Skip((pageIndex - 1) * pageSize).Limit(pageSize).ToList();
}
排序:SortBy、SortByDescending
/// <summary>
/// 排序(根据时间字段)
/// </summary>
/// <param name="isAsc">是否升序</param>
/// <returns></returns>
[HttpPost]
public List<ShipInfo> GetListBySort(bool isAsc = true)
{
if (isAsc)
{
return shipService.Find(u => true).SortBy(u => u.addTime).ToList();
}
else
{
return shipService.Find(u => true).SortByDescending(u => u.addTime).ToList();
}
}
7. 创建索引
索引:CreateOne+CreateIndexModel
/// <summary>
/// 创建索引
/// </summary>
/// <param name="indexName">索引名称</param>
/// <returns></returns>
[HttpPost]
public string CreateOne(string indexName)
{
var indexKeysDefinition = Builders<ShipInfo>.IndexKeys.Ascending(indexName);
shipService.Indexes.CreateOne(new CreateIndexModel<ShipInfo>(indexKeysDefinition));
return "ok";
}
如下图:
!
- 作 者 : Yaopengfei(姚鹏飞)
- 博客地址 : http://www.cnblogs.com/yaopengfei/
- 声 明1 : 如有错误,欢迎讨论,请勿谩骂^_^。
- 声 明2 : 原创博客请在转载时保留原文链接或在文章开头加上本人博客地址,否则保留追究法律责任的权利。