添加记录:
1.save方法
stu=Student(name="李四",age=18,sex=0,birthday="2021-11-23")
stu.save()
print(stu.name)
print(stu.age)
print(stu.sex)
print(stu.birthday)
2.create方法(常用)
stu=Student.objects.create(name="王五",age=68,sex=2,birthday="1994-11-13")
print(stu.name)
print(stu.age)
print(stu.sex)
print(stu.birthday)
示例:
student/models.py文件
from django.db import models
# Create your models here.
class Student(models.Model):
sex_choices = (
(0, "女"),
(1, "男"),
(2, "保密")
)
# id=models.AutoField(primary_key=True)
name = models.CharField(max_length=32, unique=True, verbose_name="姓名")
age = models.SmallIntegerField(verbose_name="年龄",default=18)
sex = models.SmallIntegerField(choices=sex_choices)
birthday = models.DateField()
class Meta:
db_table = "cc_student"
ORM/urls.py文件
"""ORM URL Configuration
from django.contrib import admin
from django.urls import path,include
import student
urlpatterns = [
path('admin/', admin.site.urls),
path('student/', include("student.urls")),
]
student/urls.py文件
from django.contrib import admin
from django.urls import path
from .views import student_add
urlpatterns = [
path('add/', student_add),
]
student/views.py文件
from django.shortcuts import render,HttpResponse
from .models import Student
# Create your views here.
"""
-- auto-generated definition
create table cc_student
(
id bigint auto_increment
primary key,
name varchar(32) not null,
age smallint not null,
sex smallint not null,
birthday date not null,
constraint name
unique (name)
);
"""
def student_add(request):
### 方法一
# stu=Student(name="李四",age=18,sex=0,birthday="2021-11-23")
# stu.save()
# print(stu.name)
# print(stu.age)
# print(stu.sex)
# print(stu.birthday)
### 方法二
stu=Student.objects.create(name="王五",age=68,sex=2,birthday="1994-11-13")
print(stu.name)
print(stu.age)
print(stu.sex)
print(stu.birthday)
return HttpResponse("添加成功")
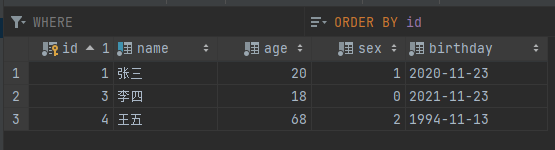
基础查询:
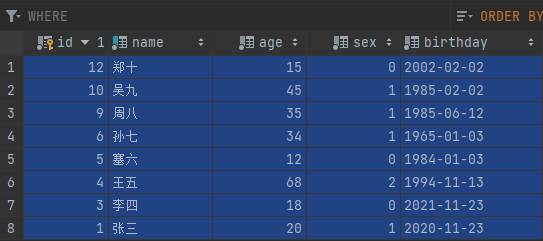
student/views.py文件
from django.shortcuts import render,HttpResponse
from .models import Student
def student_select(request):
# all():查询所有对象,返回queryset对象,查询集,也称查询结果集、QuerySet,表示从数据库中获取的对象集合。
stu = Student.objects.all()
stu1=Student.objects.all()[0]
print(stu)
print(stu1.name)
print(stu1.birthday)
# filter()筛选条件相匹配的对象,返回queryset对象
stu2=Student.objects.filter(sex=0)
print(stu2)
# get()返回与所给筛选条件相匹配的对象,返回结果有且只有一个, 如果符合筛选条件的对象超过一个或者没有都会抛出错误
stu3=Student.objects.get(sex=2)
print(stu3.name)
# first()、last() 分别为查询集的第一条记录和最后一条记录
stu4=Student.objects.first()
print(stu4.name)
stu5 = Student.objects.last()
print(stu5.name)
# exclude():筛选条件不匹配的对象,返回queryset对象。
stu6=Student.objects.exclude(name="塞六")
print(stu6)
# order_by():对查询结果排序
# order_by("字段") # 按指定字段正序显示,相当于 asc 从小到大
# order_by("-字段") # 按字段倒序排列,相当于 desc 从大到小
# order_by("第一排序","第二排序",...)
stu7=Student.objects.order_by('-age')
print(stu7)
# count():查询集中对象的个数
stu8=Student.objects.filter(sex=1).count()
print(stu8)
# values()/values_list()
#value()把结果集中的模型对象转换成字典,并可以设置转换的字段列表,达到减少内存损耗,提高性能
#values_list(): 把结果集中的模型对象转换成列表,并可以设置转换的字段列表(元祖),达到减少内存损耗,提高性能
stu9=Student.objects.values("name","age")
print(stu9)
# distinct():从返回结果中剔除重复纪录。返回queryset。
stu10=Student.objects.values('sex').distinct()
print(stu10)
# exists():判断查询集中是否有数据,如果有则返回True,没有则返回False
print(Student.objects.exists())
return HttpResponse("*******************查询成功*******************")