路由控制器:
from django.urls import path # 字符串路由
from django.urls import re_path # 正则路由,会把url地址看成一个正则模式与客户端的请求url地址进行正则匹配
# path和re_path 使用参数一致.仅仅在url参数和接收参数时写法不一样
基本使用:
from django.contrib import admin
from django.urls import path,re_path
from app01 import views
urlpatterns = [
path('admin/', admin.site.urls),
path(r'^articles/2003/$', views.special_case_2003),
re_path(r'^articles/([0-9]{4})/$', views.year_archive),
re_path(r'^articles/([0-9]{4})/([0-9]{2})/$', views.month_archive),
## 有名分组,根据名称进行分组,后面方便传参
re_path(r'^articles/(?P<year>[0-9]{4})/(?P<month>[0-9]{2})/$', views.month_archive2),
]
路由分发:
- 使用路由分发(include),让每个app目录都单独拥有自己的 urls
全局urls配置
from django.contrib import admin
from django.urls import path,include
urlpatterns = [
path('admin/', admin.site.urls),
path('app01/',include("app01.urls"))
]
app01.urls配置
from django.urls import path
from app01 import views
urlpatterns = [
path('',views.index)
]
views.index配置
from django.shortcuts import render
def index(request):
return render(request,"index.html")
index.html配置
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<h3>hello index.........</h3>
</body>
</html>
执行结果:
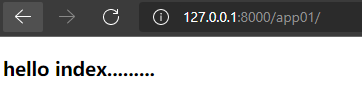
路由转发器:
- 内置的url转换器并不能满足我们的需求,因此django给我们提供了一个接口可以让我们自己定义自己的url转换器
全局urls配置
from django.contrib import admin
from django.urls import path,register_converter,include
from app01.views import index
class Mobile(object):
regex="1[3-9]\d{9}"
def to_python(self,values):
return values
register_converter(Mobile, "mo")
urlpatterns = [
path("index/<mo:m>",index)
]
app01.views函数index配置
from django.shortcuts import HttpResponse
def index(request,m):
print(":::",type(m))
return HttpResponse(f"hi,{m}用户")
执行结果:

反向解析:
app07/urls.py配置文件
from django.urls import path
from app07.views import order,index
urlpatterns = [
path('index/', index, name="ind"),
path('order/', order, name="ord")
]
模板反向解析:
app07/views.py配置文件
from django.shortcuts import render
def order(request):
return render(request,"order.html")
def index(request):
return render(request,"index.html")
templates/order.html配置文件
{% extends 'basis.html' %}
{% block content %}
{#保留原有数据#}
{{ block.super }}
<h3>修改原有数据</h3>
{% endblock %}
templates/basis.html配置文件
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
{% block content %}
<h1>1111111111111111111</h1>
{% endblock %}
{% block mes %}
<h1>22222222222222222</h1>
{% endblock %}
<p><a href="{% url 'ind' %}">跳转到主页</a></p>
<style>
.advertise{
width: 200px;
height: 100px;
background-color: orange;
position: fixed;
bottom: 10px;
right: 10px;
}
</style>
<div class="advertise">广告</div>
</body>
视图反向解析:
app07/views.py配置文件
from django.shortcuts import render,redirect
from django.urls import reverse
def order(request):
print("反向解析地址:",reverse("ord"))
return redirect(reverse("ind"))
def index(request):
return render(request,"index.html")
templates/index.html配置文件
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<H2>首页页面</H2>
</body>
</html>