Spring AOP 记录日志
去年的时候由于业务量快速上升,导致我们提供的很多接口或者第三方的接口都出现性能问题,请求处理时间都特别长,为了能够快速检查出哪些接口或者业务方法响应时间特别长,PM让我们记录下每个接口或方法的处理时长。就一句MMP,要在每个方法之前之后都加上当前时间然后在相减?特么的想想都要死人了,这特么没法工作了。但是在程序员的思维里是:重复的东西我们要让程序去做,我们负责实现这个程序。
好了,废话不说。
框架:Maven+Spring
一、通过XML配置实现AOP 记录方法执行时间
1、引入jar
<!-- AOP JAR --> <dependency> <groupId>org.aspectj</groupId> <artifactId>aspectjweaver</artifactId> <version>1.8.9</version> </dependency> <!-- Spring JAR --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-aop</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-context</artifactId> <version>${spring.version}</version> </dependency> <!-- 测试使用的JAR --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-test</artifactId> <version>${spring.version}</version> <scope>provided</scope> </dependency> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>4.12</version> <!-- 表示开发的时候引入,发布的时候不会加载此包 --> <scope>test</scope> </dependency>
2、编写通知类
package com.ssm.interf;
import org.aopalliance.intercept.MethodInterceptor; import org.aopalliance.intercept.MethodInvocation; import org.springframework.util.StopWatch; import org.springframework.util.StringUtils; public class MethodTimeAdvice implements MethodInterceptor { @Override public Object invoke(MethodInvocation invocation) throws Throwable { StopWatch clock = new StopWatch(); clock.start(); // 计时开始 Object result = invocation.proceed(); clock.stop(); // 计时结束 // 方法参数类型,转换成简单类型 Class[] params = invocation.getMethod().getParameterTypes(); String[] simpleParams = new String[params.length]; for (int i = 0; i < params.length; i++) { simpleParams[i] = params[i].getSimpleName(); } System.out.println("Takes:" + clock.getTotalTimeMillis() + " ms [" + invocation.getThis().getClass().getName() + "." + invocation.getMethod().getName() + "(" + StringUtils.arrayToDelimitedString(simpleParams, ",") + ")]"); return result; } }
StopWatch 是Spring 提供的一个计时类。
3、spring的xml配置
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:aop="http://www.springframework.org/schema/aop" xmlns:tx="http://www.springframework.org/schema/tx" xmlns:context="http://www.springframework.org/schema/context" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-4.0.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-4.0.xsd http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-4.0.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.0.xsd"> <context:component-scan base-package="com.ssm" /> <!-- 日志时间打印 --> <aop:config> <aop:pointcut id="runTimePc" expression="execution(* com.ssm.service.impl.*.*(..))" /> <!-- Spring 2.0 可以用 AspectJ 的语法定义 Pointcut,这里拦截 service 包中的所有方法 --> <aop:advisor advice-ref="methodTimeAdvice" pointcut-ref="runTimePc" /> <!--<aop:advisor id="methodTimeLog" advice-ref="methodTimeAdvice" pointcut="execution(* com.tmg.perfomance.service.impl.*.*(..))"/> --> </aop:config> <!-- 默认使用JDK动态代理,对于Schema风格配置切面使用如下方式来指定使用CGLIB代理。 <aop:config proxy-target-class="true"></aop:config> --> <bean id="methodTimeAdvice" class="com.ssm.interf.MethodTimeAdvice" /> </beans>
expression="execution(* com.ssm.service.impl.*.*(..))" 代表切入点,意思是com.ssm.service.impl包下的所有方法都会被切入 时间记录
4、测试的类
package com.ssm.service.impl; import org.springframework.stereotype.Service; import com.ssm.service.AopLogService; @Service("aopLogService") public class AopLogServiceImpl implements AopLogService { public void operation1() { System.out.println("运行方法:operation1"); try { Thread.sleep(1000); } catch (InterruptedException e) { // TODO Auto-generated catch block e.printStackTrace(); } } public void operation2() { System.out.println("运行方法:operation2"); try { Thread.sleep(2000); } catch (InterruptedException e) { // TODO Auto-generated catch block e.printStackTrace(); } } public void operation3() { System.out.println("运行方法:operation3"); try { Thread.sleep(3000); } catch (InterruptedException e) { // TODO Auto-generated catch block e.printStackTrace(); } } }
5、junit 运行测试
package org.logRumTime; import org.BaseTest; import org.junit.Test; import org.springframework.beans.factory.annotation.Autowired; import com.ssm.service.AopLogService; public class RumTimeTest extends BaseTest { @Autowired private AopLogService aopLogService; @Test public void testOperation() { aopLogService.operation1(); aopLogService.operation2(); aopLogService.operation3(); } public AopLogService getAopLogService() { return aopLogService; } public void setAopLogService(AopLogService aopLogService) { this.aopLogService = aopLogService; } }
BaseTest 类代码
package org; import org.junit.runner.RunWith; import org.springframework.test.context.ContextConfiguration; import org.springframework.test.context.junit4.SpringJUnit4ClassRunner; @RunWith(SpringJUnit4ClassRunner.class)//使用junit4进行测试 @ContextConfiguration(locations= {"classpath:app-context-core.xml"}) public class BaseTest { }
运行结果:
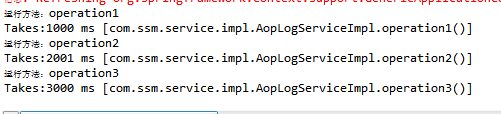