|
|
这个作业属于哪个课程 |
C语言课程设计与游戏开发实践教程 |
这个作业要求在哪里 |
2019春季第十四周作业 |
我的课程目标 |
学会函数与之的结构利用 |
这个作业在哪个具体方面帮助我实现目标 |
写出一个简单的飞机游戏 |
参考文献 |
无 |
一、2019春第二次课程设计实验报告
一、实验项目名称:
飞机空战游戏
二、 实验项目功能描述:
进一步改进飞机游戏为空战游戏,并实现多台敌机、发射散弹等效果。新增了积分系统,不同的分数段有不同的效果(敌机速度、子弹速度.)。
三、 项目模块结构介绍:
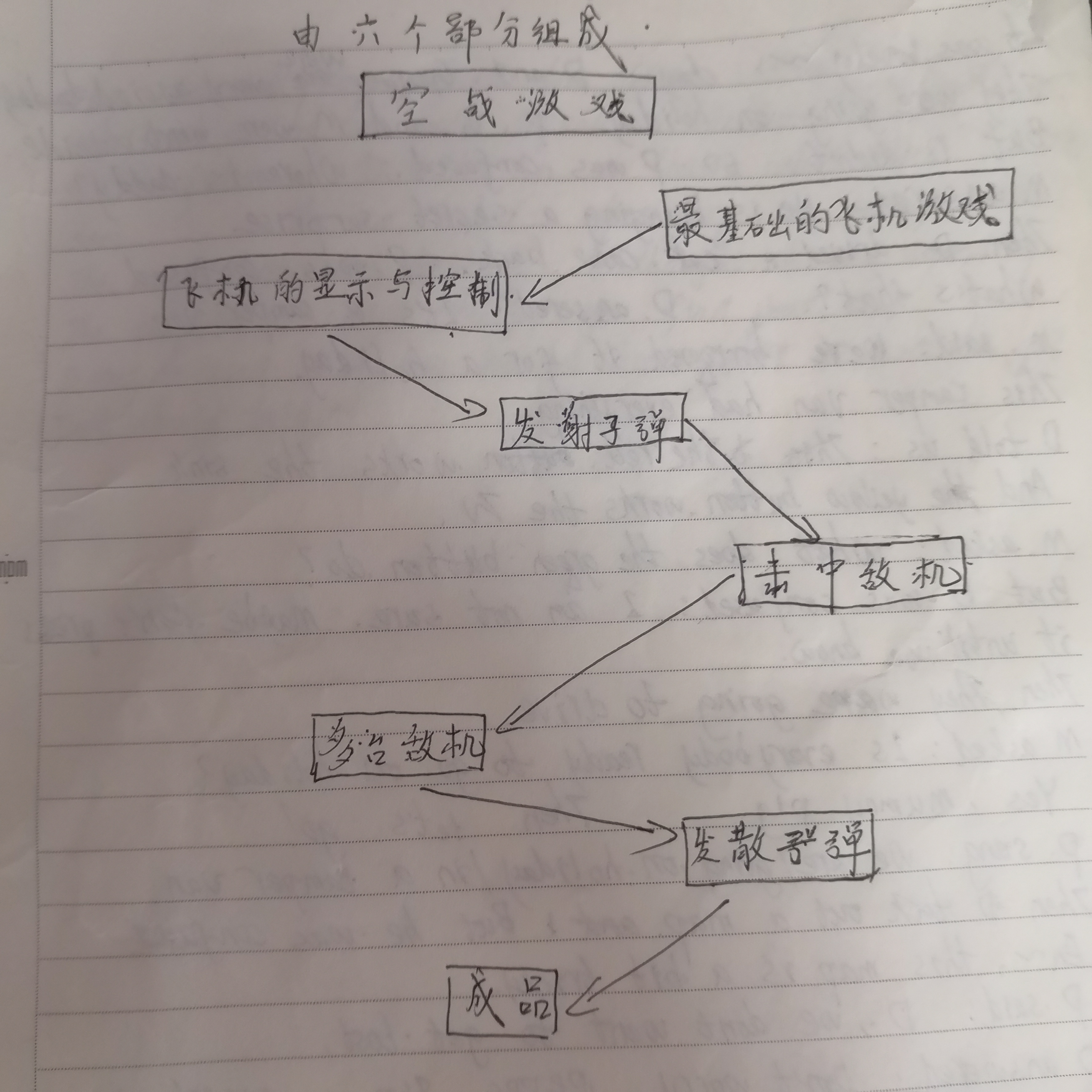
1)飞机的显示与控制
###**实验代码**
#include<stdio.h>
#include<stdlib.h>
#include<conio.h>
#include<windows.h>
#define High 25
#define Width 50 /*游戏画面尺寸*/
/*全局变量*/
int position_x,position_y; /*飞机位置*/
int canvas[High][Width]={0}; /*飞机画面尺寸*/
void gotoxy(int x,int y) /*将光标移动到(x,y)位置*/
{
HANDLE handle = GetStdHandle(STD_OUTPUT_HANDLE);
COORD pos;
pos.X=x;
pos.Y=y;
SetConsoleCursorPosition(handle,pos);
}
void startup() /*数据初始化*/
{
position_x=High/2;
position_y=Width/2;
canvas[position_x] [position_y]=1;
}
void show() /*显示画面*/
{
gotoxy(0,0);
int i,j;
for(i=0;i<High;i++)
{
for(j=0;j<Width;j++)
{
if(canvas[i][j]==0)
printf(" "); /*输出空格*/
else if(canvas[i][j]==1)
printf(" * "); /*输出飞机*/
}
printf("/n");
}
}
void updateWithoutInput() /*与用户输入有关的更新*/
{
}
void updateWithInput()
{
char input;
if(kbhit()) /*判断是否输入*/
{
input = getch(); /*根据用户的不同输入来移动,不必输入回车*/
if (input == 'a')
{
canvas[position_x] [position_y]=0;
position_y--; /*位置左移*/
canvas[position_x] [position_y]=1;
}
else if(input =='d')
{
canvas[position_x] [position_y]=0;
position_y++; /*位置右移*/
canvas[position_x] [position_y]=1;
}
else if(input =='w')
{
canvas[position_x] [position_y]=0;
position_x--; /*位置上移*/
canvas[position_x] [position_y]=1;
}
else if(input =='s')
{
canvas[position_x] [position_y]=0;
position_x--; /*位置下移*/
canvas[position_x] [position_y]=1;
}
}
}
int main()
{
startup(); /*数据的初始化*/
while(1) /*游戏循环执行*/
{
show(); /*显示画面*/
updateWithoutInput(); /*与用户输入无关的更新*/
updateWithInput(); /*与用户输入无关的更新*/
}
return 0;
}
2)发射子弹
###**实验代码**
#include<stdio.h>
#include<stdlib.h>
#include<conio.h>
#include<windows.h>
#define High 25
#define Width 50
int position_x,position_y;
int canvas[High][Width] = {0};
void gotoxy(int x,int y)
{
HANDLE handle = GetStdHandle(STD_OUTPUT_HANDLE);
COORD pos;
pos.X = x;
pos.Y = y;
SetConsoleCursorPosition(handle,pos);
}
void startup()
{
position_x = High/2;
position_y = Width/2;
canvas[position_x][position_y]=1;
}
void show()
{
gotoxy(0,0);
int i,j;
for (i=0;i<High;i++){
for(j=0;j<Width;j++){
if (canvas[i][j]==0)
printf(" ");
else if(canvas[i][j]==1)
printf(" * ");
else if (canvas[i][j]==2)
printf(" | ");
}
printf("\n");
}
}
void updateWithoutInput()
{
int i,j;
for (i=0;i<High;i++){
for(j=0;j<Width;j++){
if (canvas[i][j]==2) {
canvas[i][j]=0;
if(i>0)
canvas[i-1][j]=2;
}
}
}
}
void updateWithInput()
{
char input;
if((kbhit))
{
input = getch();
if (input = 'a')
{
canvas[position_x][position_y] = 0;
position_y--;
canvas[position_x][position_y] = 1;
}
else if (input = 'd') {
canvas[position_x][position_y] = 0;
position_y++;
canvas[position_x][position_y] = 1;
}
else if (input = 'w')
{
canvas[position_x][position_y] = 0;
position_x--;
canvas[position_x][position_y] = 1;
}
else if (input = 's')
{
canvas[position_x][position_y] = 0;
position_x++;
canvas[position_x][position_y] = 1;
}
else if (input = ' ')
{
canvas[position_x][position_y] = 2;
}
}
}
int main ()
{
startup();
while (1) {
show();
updateWithoutInput();
updateWithInput();
}
return 0;
}
3)击中敌机
###**实验代码**
#include<stdio.h>
#include<stdlib.h>
#include<conio.h>
#include<windows.h>
#define High 15
#define Width 25
int position_x,position_y;
int enemy_x,enemy_y;
int canvas[High][Width] = {0};
int score;
void gotoxy(int x,int y)
{
HANDLE handle = GetStdHandle(STD_OUTPUT_HANDLE);
COORD pos;
pos.X = x;
pos.Y = y;
SetConsoleCursorPosition(handle,pos);
}
void startup()
{
position_x = High-1;
position_y = Width/2;
canvas[position_x][position_y]=1;
enemy_x=0;
enemy_y=position_y;
canvas[enemy_x][enemy_y]=3;
score=0;
}
void show()
{
gotoxy(0,0);
int i,j;
for (i=0;i<High;i++){
for(j=0;j<Width;j++){
if (canvas[i][j]==0)
printf(" ");
else if(canvas[i][j]==1)
printf(" * ");
else if (canvas[i][j]==2)
printf(" | ");
else if(canvas[i][j]==3)
printf("@");
}
printf("\n");
}
printf("得分:%3d\n",score);
Sleep(20);
}
void updateWithoutInput()
{
int i,j;
for (i=0;i<High;i++){
for(j=0;j<Width;j++){
if (canvas[i][j]==2) {
score++;
canvas[enemy_x][enemy_y]=0;
enemy_x=0;
enemy_y=rand()%Width;
canvas[enemy_x][enemy_y]=3;
canvas[i][j]=0;
}
canvas[i][j]=0;
if(i>0)
canvas[i-1][j]=2;
}
}
}
if((position_x==enemy_x)&&(position_y==enemy_y))
{
printf("失败!\n");
Sleep(3000);
systm("pause");
exit(0);
}
if (enemy_x>High)
{
canvas[enemy_x][enemy_y]=0;
enemy_x=0;
enemy_y=rand()%Width;
canvas[enemy_x][enemy_y]=3;
score--;
}
static int speed = 0;
if (speed<10)
speed++;
if(speed==10)
{
canvas[enemy_x][enemy_y]=0;
enemy_x++;
speed ==0;
canvas[enemy_x][enemy_y]=3;
}
}
void updateWithInput()
{
char input;
if((kbhit))
{
input = getch();
if (input = 'a')
{
canvas[position_x][position_y] = 0;
position_y--;
canvas[position_x][position_y] = 1;
}
else if (input = 'd') {
canvas[position_x][position_y] = 0;
position_y++;
canvas[position_x][position_y] = 1;
}
else if (input = 'w')
{
canvas[position_x][position_y] = 0;
position_x--;
canvas[position_x][position_y] = 1;
}
else if (input = 's')
{
canvas[position_x][position_y] = 0;
position_x++;
canvas[position_x][position_y] = 1;
}
else if (input = ' ')
{
canvas[position_x][position_y] = 2;
}
}
}
int main ()
{
startup();
while (1) {
show();
updateWithoutInput();
updateWithInput();
}
return 0;
}
4)多台敌机
###**实验代码**
#include<stdio.h>
#include<stdlib.h>
#include<conio.h>
#include<windows.h>
#define High 15 /*游戏画面尺寸*/
#define Width 25
#define EnemyNum 5 /*敌机数量*/
/*全局变量*/
int position_x,position_y; //飞机的位置
int enemy_x[EnemyNum],enemy_y[EnemyNum]; //敌机的位置
int canvas[High][Width] = {0}; //二维数组存游戏画面中对应的元素
//0为空格,1为飞机* ,2为子弹 ? ,3为敌机@ ,
int score; //得分
void gotoxy(int x,int y) //将光标移动到(x,y)位置
{
HANDLE handle = GetStdHandle(STD_OUTPUT_HANDLE);
COORD pos;
pos.X = x;
pos.Y = y;
SetConsoleCursorPosition(handle,pos);
}
void startup() //数据初始化
{
position_x = High-1;
position_y = Width/2;
canvas[position_x][position_y]=1;
int k;
for(k=0;k<EnemyNum;k++)
{
enemy_x[k]= rand() %2;
enemy_y[k]= rand() %Width;
canvas[enemy_x[k]][enemy_y[k]]=3;
}
score=0;
}
void show() //显示画面
{
gotoxy(0,0); //将光标移动到原点位置,以下画面清屏
int i,j;
for (i=0;i<High;i++){
for(j=0;j<Width;j++)
{
if (canvas[i][j]==0)
printf(" "); //输出空格
else if(canvas[i][j]==1)
printf(" * "); //输出飞机*
else if (canvas[i][j]==2)
printf(" | "); //输出飞机|
else if(canvas[i][j]==3)
printf("@"); //输出飞机@
}
printf("\n");
}
printf("得分:%3d\n",score);
Sleep(20);
}
void updateWithoutInput() //与用户输入无关的更新
{
int i,j,k;
for (i=0;i<High;i++)
{
for(j=0;j<Width;j++)
{
if (canvas[i][j]==2)
{
for(k=0;k<EnemyNum;k++)
{
if((i==enemy_x[k])&&(j==enemy_y[k])) //子弹击中敌机
{
score++; //分数加 1
canvas[enemy_x[k]][enemy_y[k]]=0;
enemy_x[k]= rand() %2; //产生新的飞机
enemy_y[k]= rand() %Width;
canvas[enemy_x[k]][enemy_y[k]]=3;
canvas[i][j] = 0; //子弹消失
}
}
//子弹向上移动
canvas[i][j]=0;
if(i>0)
canvas[i-1][j]=2;
}
}
}
static int speed = 0;
if(speed<20)
speed++;
for(k=0;k<EnemyNum;k++)
{
if((position_x==enemy_x[k])&&(position_y==enemy_y[k]))
{
printf("失败!\n");
Sleep(3000);
system("pause");
exit(0);
}
}
if (enemy_x[k]>High) //敌机跑出显示屏幕
{
canvas[enemy_x[k]][enemy_y[k]]=0;
enemy_x[k]= rand() %2; //产生新的飞机
enemy_y[k]= rand() %Width;
canvas[enemy_x[k]][enemy_y[k]]=3;
score--; //减分
}
if(speed == 20)
{
//敌机下落
for(k=0;k<EnemyNum;k++)
{
canvas[enemy_x[k]][enemy_y[k]]=0;
enemy_x[k]++;
speed = 0;
canvas[enemy_x[k]][enemy_y[k]]=3;
}
}
}
void updateWithInput() //与用户输入有关的更新
{
char input;
if((kbhit)) //判断是否有输入
{
input = getch(); //根据用户的不同输入来移动,不必输入回车
if (input == 'a')
{
canvas[position_x][position_y] = 0;
position_y--; //位置左移
canvas[position_x][position_y] = 1;
}
else if (input == 'd') {
canvas[position_x][position_y] = 0;
position_y++; //位置右移
canvas[position_x][position_y] = 1;
}
else if (input == 'w')
{
canvas[position_x][position_y] = 0;
position_x--; //位置上移
canvas[position_x][position_y] = 1;
}
else if (input == 's')
{
canvas[position_x][position_y] = 0;
position_x++; //位置下移
canvas[position_x][position_y] = 1;
}
else if (input == ' ') //发射子弹
{
canvas[position_x-1][position_y] = 2; //发射子弹的初始位置在飞机的正上方
}
}
}
int main ()
{
startup(); //数据初始化
while (1) //游戏循环执行
{
show(); //显示画面
updateWithoutInput(); //与用户输入无关的更新
updateWithInput(); //与用户输入有关的更新
}
return 0;
}
5)发散子弹
###**实验代码**
#include<stdio.h>
#include<stdlib.h>
#include<conio.h>
#include<windows.h>
#define High 15 /*游戏画面尺寸*/
#define Width 25
#define EnemyNum 5 /*敌机数量*/
/*全局变量*/
int position_x,position_y; //飞机的位置
int enemy_x[EnemyNum],enemy_y[EnemyNum]; //敌机的位置
int canvas[High][Width] = {0}; //二维数组存游戏画面中对应的元素
//0为空格,1为飞机* ,2为子弹 ? ,3为敌机@ ,
int score; //得分
int BulletWidth; //子弹宽度
int EnemyMoveSpeed; //敌机的移动速度
void gotoxy(int x,int y) //将光标移动到(x,y)位置
{
HANDLE handle = GetStdHandle(STD_OUTPUT_HANDLE);
COORD pos;
pos.X = x;
pos.Y = y;
SetConsoleCursorPosition(handle,pos);
}
void startup() //数据初始化
{
position_x = High-1;
position_y = Width/2;
canvas[position_x][position_y]=1;
int k;
for(k=0;k<EnemyNum;k++)
{
enemy_x[k]= rand() %2;
enemy_y[k]= rand() %Width;
canvas[enemy_x[k]][enemy_y[k]]=3;
}
score = 0;
BulletWidth = 0;
EnemyMoveSpeed = 20;
}
void show() //显示画面
{
gotoxy(0,0); //将光标移动到原点位置,以下画面清屏
int i,j;
for (i=0;i<High;i++){
for(j=0;j<Width;j++)
{
if (canvas[i][j]==0)
printf(" "); //输出空格
else if(canvas[i][j]==1)
printf(" * "); //输出飞机*
else if (canvas[i][j]==2)
printf(" | "); //输出飞机|
else if(canvas[i][j]==3)
printf("@"); //输出飞机@
}
printf("\n");
}
printf("得分:%3d\n",score);
Sleep(20);
}
void updateWithoutInput() //与用户输入无关的更新
{
int i,j,k;
for (i=0;i<High;i++)
{
for(j=0;j<Width;j++)
{
if (canvas[i][j]==2)
{
for(k=0;k<EnemyNum;k++)
{
if((i==enemy_x[k])&&(j==enemy_y[k])) //子弹击中敌机
{
score++; //分数加 1
if(score%5==0&&EnemyMoveSpeed>3) //达到一定积分后敌机变快
EnemyMoveSpeed--;
if(score%5==0) //达到一定积分后子弹变厉害
BulletWidth++;
canvas[enemy_x[k]][enemy_y[k]]=0;
enemy_x[k]= rand() %2; //产生新的飞机
enemy_y[k]= rand() %Width;
canvas[enemy_x[k]][enemy_y[k]]=3;
canvas[i][j] = 0; //子弹消失
}
}
//子弹向上移动
canvas[i][j]=0;
if(i>0)
canvas[i-1][j]=2;
}
}
}
static int speed = 0;
if(speed<EnemyMoveSpeed)
speed++;
for(k=0;k<EnemyNum;k++)
{
if((position_x==enemy_x[k])&&(position_y==enemy_y[k])) //敌机撞到我机
{
printf("失败!\n");
Sleep(3000);
system("pause");
exit(0);
}
}
if (enemy_x[k]>High) //敌机跑出显示屏幕
{
canvas[enemy_x[k]][enemy_y[k]]=0;
enemy_x[k]= rand() %2; //产生新的飞机
enemy_y[k]= rand() %Width;
canvas[enemy_x[k]][enemy_y[k]]=3;
score--; //减分
}
if(speed == EnemyMoveSpeed)
{
//敌机下落
for(k=0;k<EnemyNum;k++)
{
canvas[enemy_x[k]][enemy_y[k]]=0;
enemy_x[k]++;
speed = 0;
canvas[enemy_x[k]][enemy_y[k]]=3;
}
}
}
void updateWithInput() //与用户输入有关的更新
{
char input;
if((kbhit)) //判断是否有输入
{
input = getch(); //根据用户的不同输入来移动,不必输入回车
if (input == 'a')
{
canvas[position_x][position_y] = 0;
position_y--; //位置左移
canvas[position_x][position_y] = 1;
}
else if (input == 'd') {
canvas[position_x][position_y] = 0;
position_y++; //位置右移
canvas[position_x][position_y] = 1;
}
else if (input == 'w')
{
canvas[position_x][position_y] = 0;
position_x--; //位置上移
canvas[position_x][position_y] = 1;
}
else if (input == 's')
{
canvas[position_x][position_y] = 0;
position_x++; //位置下移
canvas[position_x][position_y] = 1;
}
else if (input == ' ') //发射子弹
{
int left = position_y-BulletWidth;
int right = position_y+BulletWidth;
if(left<0)
left = 0;
if(right>Width-1)
right = Width-1;
int k;
for(k<left;k<=right;k++) //发射子弹
canvas[position_x-1][k] = 2; //发射子弹的初始位置在飞机的正上方
}
}
}
int main ()
{
startup(); //数据初始化
while (1) //游戏循环执行
{
show(); //显示画面
updateWithoutInput(); //与用户输入无关的更新
updateWithInput(); //与用户输入有关的更新
}
return 0;
}
四、效果截图
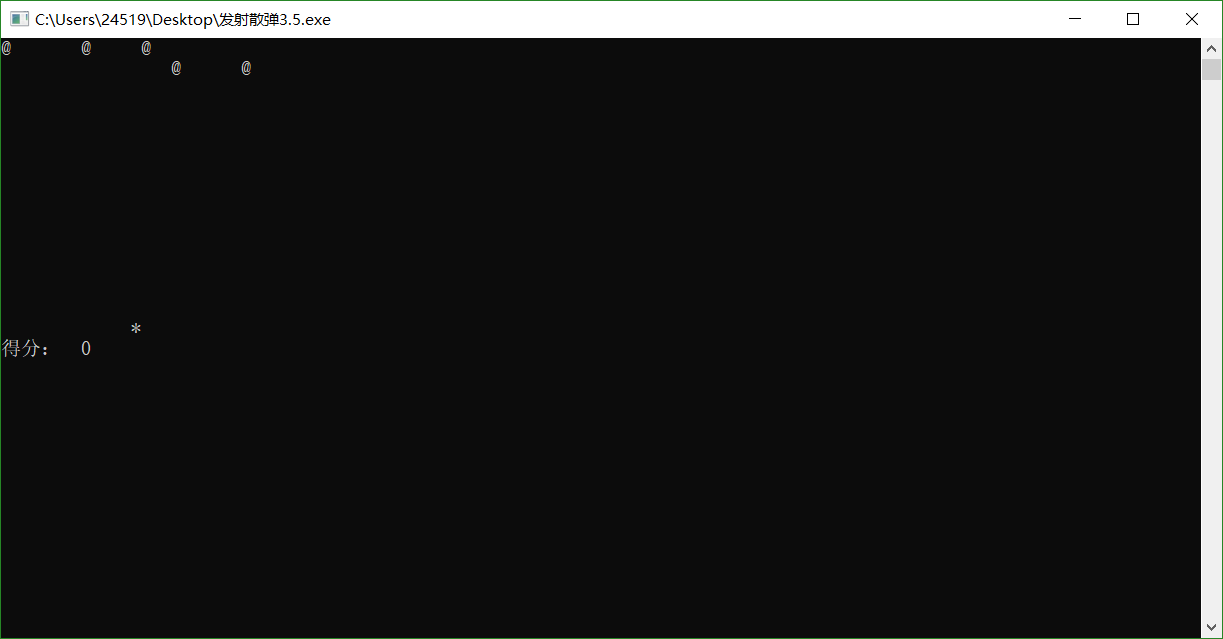
五、代码托管链接
https://gitee.com/William19991203/cruel
六、总结
本次写的游戏是在上次的《最简单的飞机游戏》基础上改变的。难度较上次有所提升,本次利用数组进一步改进了空战游戏。
1、 利用数组嵌入难度大
2、 解决办法,多看书。