C语言,PHP扩展开发,类1
c代码:
/* xuxiaobo extension for PHP */ #ifdef HAVE_CONFIG_H #include "config.h" #endif #include <stdlib.h> #include <stdio.h> #include "php.h" #include "ext/standard/info.h" #include "zend_smart_str.h" #include "zend_exceptions.h" #include "php_xuxiaobo.h" //第一个参数表示必传的参数个数,第二个参数表示最多传入的参数个数 //https://blog.csdn.net/qq_21891743/article/details/131386438 //https://www.jianshu.com/p/05616d23c0dc //https://blog.csdn.net/qq_40647372/article/details/132872085 //Z_PARAM_OPTIONAL 对应(分隔符) | Z_PARAM_STR 对应 S //Z_PARAM_OPTIONAL 只在有些参数可以不传的时候使用 #ifndef ZEND_PARSE_PARAMETERS_NONE #define ZEND_PARSE_PARAMETERS_NONE() \ ZEND_PARSE_PARAMETERS_START(0, 0) \ ZEND_PARSE_PARAMETERS_END() #endif // 自定义排序函数 void bubble_sort(zval *array_arg) { int i, j, temp; zval *entry_swap1, *entry_swap2; int count = zend_hash_num_elements(Z_ARRVAL_P(array_arg)); for (j = 0; j < count - 1; j++) { for (i = 0; i < count - 1 - j; i++) { entry_swap1 = zend_hash_index_find(Z_ARRVAL_P(array_arg), i); entry_swap2 = zend_hash_index_find(Z_ARRVAL_P(array_arg), i + 1); if (Z_LVAL_P(entry_swap1) > Z_LVAL_P(entry_swap2)) { temp = Z_LVAL_P(entry_swap1); ZVAL_LONG(entry_swap1, Z_LVAL_P(entry_swap2)); ZVAL_LONG(entry_swap2, temp); } } } } // 自定义排序函数 void bubble_sort_cn(zval *array_arg) { int i, j; zval *entry_swap1, *entry_swap2; int count = zend_hash_num_elements(Z_ARRVAL_P(array_arg)); for (j = 0; j < count - 1; j++) { for (i = 0; i < count - 1 - j; i++) { entry_swap1 = zend_hash_index_find(Z_ARRVAL_P(array_arg), i); entry_swap2 = zend_hash_index_find(Z_ARRVAL_P(array_arg), i + 1); char str1[256]; sprintf(str1, "%s", Z_STRVAL_P(entry_swap1)); char str2[256]; sprintf(str2, "%s", Z_STRVAL_P(entry_swap2)); if (strlen(str1) > strlen(str2)) { ZVAL_STRING(entry_swap1, str2); ZVAL_STRING(entry_swap2, str1); } } } } PHP_METHOD(Xuxiaobo, extload) { ZEND_PARSE_PARAMETERS_NONE(); php_printf("扩展%s已经加载并运行", "xuxiaobo"); } PHP_METHOD(Xuxiaobo, hello) { char *var = "世界"; size_t var_len = sizeof("世界") - 1; zend_string *retval; ZEND_PARSE_PARAMETERS_START(0, 1) Z_PARAM_OPTIONAL Z_PARAM_STRING(var, var_len) ZEND_PARSE_PARAMETERS_END(); retval = strpprintf(0, "你好,%s", var); RETURN_STR(retval); } PHP_METHOD(Xuxiaobo, hellomust) { char *var; size_t var_len; zend_string *retval; ZEND_PARSE_PARAMETERS_START(1, 1) Z_PARAM_STRING(var, var_len) ZEND_PARSE_PARAMETERS_END(); retval = strpprintf(0, "你好,%s", var); RETURN_STR(retval); } PHP_METHOD(Xuxiaobo, hellomuch) { char *name; size_t name_len; char *other = "你好"; size_t other_len = sizeof("你好") - 1; zend_string *retval; ZEND_PARSE_PARAMETERS_START(1, 2) Z_PARAM_STRING(name, name_len) Z_PARAM_OPTIONAL Z_PARAM_STRING(other, other_len) ZEND_PARSE_PARAMETERS_END(); retval = strpprintf(0, "%s,%s", other, name); RETURN_STR(retval); } PHP_METHOD(Xuxiaobo, inputints) { zend_long num1, num2, num3, num4; zend_string *retval; ZEND_PARSE_PARAMETERS_START(4, 4) Z_PARAM_LONG(num1) Z_PARAM_LONG(num2) Z_PARAM_LONG(num3) Z_PARAM_LONG(num4) ZEND_PARSE_PARAMETERS_END(); // 输出数字 array_init(return_value); add_index_long(return_value, 0, num1); add_index_long(return_value, 1, num2); add_index_long(return_value, 2, num3); add_index_long(return_value, 3, num4); } PHP_METHOD(Xuxiaobo, inputstrs) { char *name1, *name2, *name3, *name4, *name5; size_t name_len1, name_len2, name_len3, name_len4, name_len5; zend_string *retval; ZEND_PARSE_PARAMETERS_START(5, 5) Z_PARAM_STRING(name1, name_len1) Z_PARAM_STRING(name2, name_len2) Z_PARAM_STRING(name3, name_len3) Z_PARAM_STRING(name4, name_len4) Z_PARAM_STRING(name5, name_len5) ZEND_PARSE_PARAMETERS_END(); // 输出数字 array_init(return_value); add_index_string(return_value, 0, name1); add_index_string(return_value, 1, name2); add_index_string(return_value, 2, name3); add_index_string(return_value, 3, name4); add_index_string(return_value, 4, name5); } PHP_METHOD(Xuxiaobo, inputarray) { zval *array; // 检查参数数量和类型 if (zend_parse_parameters(ZEND_NUM_ARGS(), "a", &array) == FAILURE) { RETURN_NULL(); } // 将数组拷贝到返回数组中 RETVAL_ARR(zend_array_dup(Z_ARRVAL_P(array))); } PHP_METHOD(Xuxiaobo, sortarray) { zval *array; // 检查参数数量和类型 if (zend_parse_parameters(ZEND_NUM_ARGS(), "a", &array) == FAILURE) { RETURN_NULL(); } bubble_sort(array); RETURN_ZVAL(array, 1, 0); } // 扩展函数,用于排序中文数组 PHP_METHOD(Xuxiaobo, sortarraycn) { zval *array; // 检查参数数量和类型 if (zend_parse_parameters(ZEND_NUM_ARGS(), "a", &array) == FAILURE) { RETURN_NULL(); } bubble_sort_cn(array); RETURN_ZVAL(array, 1, 0); } PHP_RINIT_FUNCTION(xuxiaobo) { #if defined(ZTS) && defined(COMPILE_DL_XUXIAOBO) ZEND_TSRMLS_CACHE_UPDATE(); #endif return SUCCESS; } // 请求清理 PHP_RSHUTDOWN_FUNCTION(xuxiaobo) { return SUCCESS; } // 清理资源 PHP_MSHUTDOWN_FUNCTION(xuxiaobo) { return SUCCESS; } PHP_MINFO_FUNCTION(xuxiaobo) { php_info_print_table_start(); php_info_print_table_header(2, "xuxiaobo support", "enabled"); php_info_print_table_end(); } const zend_function_entry xuxiaobo_functions[] = { PHP_ME(Xuxiaobo, extload, NULL, ZEND_ACC_PUBLIC) PHP_ME(Xuxiaobo, hello, NULL, ZEND_ACC_PUBLIC) PHP_ME(Xuxiaobo, hellomust, NULL, ZEND_ACC_PUBLIC) PHP_ME(Xuxiaobo, hellomuch, NULL, ZEND_ACC_PUBLIC) PHP_ME(Xuxiaobo, inputints, NULL, ZEND_ACC_PUBLIC) PHP_ME(Xuxiaobo, inputstrs, NULL, ZEND_ACC_PUBLIC) PHP_ME(Xuxiaobo, inputarray, NULL, ZEND_ACC_PUBLIC) PHP_ME(Xuxiaobo, sortarray, NULL, ZEND_ACC_PUBLIC) PHP_ME(Xuxiaobo, sortarraycn, NULL, ZEND_ACC_PUBLIC) {NULL, NULL, NULL} }; // 注册类或者函数等 PHP_MINIT_FUNCTION(xuxiaobo) { zend_class_entry xuxiaobo_ce; INIT_CLASS_ENTRY(xuxiaobo_ce, "Xuxiaobo", xuxiaobo_functions); zend_register_internal_class(&xuxiaobo_ce TSRMLS_CC); return SUCCESS; } zend_module_entry xuxiaobo_module_entry = { STANDARD_MODULE_HEADER, PHP_XUXIAOBO_EXTNAME, NULL, //xuxiaobo_functions PHP_MINIT(xuxiaobo), NULL, //PHP_MSHUTDOWN(xuxiaobo) NULL, //PHP_RINIT(xuxiaobo) NULL, //PHP_RSHUTDOWN(xuxiaobo) NULL, //PHP_MINFO(xuxiaobo) PHP_XUXIAOBO_VERSION, STANDARD_MODULE_PROPERTIES }; #ifdef COMPILE_DL_XUXIAOBO # ifdef ZTS ZEND_TSRMLS_CACHE_DEFINE() # endif ZEND_GET_MODULE(xuxiaobo) #endif
php代码:
<?php header("Content-type:text/html;charset=utf-8"); error_reporting(E_ALL); ini_set('display_errors', 1); ini_set('memory_limit', '-1'); set_time_limit(0); $arr1 = ['苏颜回', '柳如是', '于又微', '李之仪', '高渐离', '张九龄', '关汉卿', '卢照邻', '卓文君', '王羲之', '许唐', '陆宇', '中央嘉措','纳兰容若', '陆九渊', '顾恺之', '李清照', '王昭君', '白居易', '张若虚', '颜真卿', '林丰眠', '温庭筠', '关山月', '南怀瑾', '梁思成','林徽因']; $xxb = new Xuxiaobo(); echo "<title>PHP扩展开发</title>\n\n"; echo "<h1>PHP扩展开发</h1>\n\n"; echo "<b>1、Xuxiaobo::extload() : </b><pre>\n"; $xxb->extload(); echo "</pre>\n\n"; echo "<b>2、Xuxiaobo::hello() : </b>\n"; echo "<pre>".$xxb->hello()."</pre>\n\n"; $input = $arr1[random_int(0, count($arr1)-1)]; echo "<b>2.1、Xuxiaobo::hello(\"{$input}\") : </b>\n"; echo "<pre>".$xxb->hello($input)."</pre>\n\n"; $input = $arr1[random_int(0, count($arr1)-1)]; echo "<b>3、Xuxiaobo::hellomust(\"{$input}\") : </b>\n"; echo "<pre>".$xxb->hellomust($input)."</pre>\n\n"; echo "<b>3.1、Xuxiaobo::hellomust() : </b>\n"; echo "<pre>".$xxb->hellomust()."</pre>\n\n"; $input1 = $arr1[random_int(0, count($arr1)-1)]; $input2 = "卧槽"; echo "<b>4、Xuxiaobo::hellomuch(\"{$input1}\") : </b>\n"; echo "<pre>".$xxb->hellomuch($input1)."</pre>\n\n"; echo "<b>4.1、Xuxiaobo::hellomuch(\"{$input1}\", \"{$input2}\") : </b>\n"; echo "<pre>".$xxb->hellomuch($input1, $input2)."</pre>\n\n"; $a = random_int(1, 10); $b = random_int(1, 10); $c = random_int(1, 10); $d = random_int(1, 10); $e = $xxb->inputints($a, $b, $c, $d); $f = json_encode($e, JSON_UNESCAPED_UNICODE | JSON_UNESCAPED_SLASHES); //print_r($e, true); echo "<b>5、Xuxiaobo::inputints({$a}, {$b}, {$c}, {$d}) : </b>\n"; echo "<pre>".$f."</pre>\n\n"; $a = $arr1[random_int(0, count($arr1)-1)]; $b = $arr1[random_int(0, count($arr1)-1)]; $c = $arr1[random_int(0, count($arr1)-1)]; $d = $arr1[random_int(0, count($arr1)-1)]; $e = $arr1[random_int(0, count($arr1)-1)]; $f = $xxb->inputstrs($a, $b, $c, $d, $e); $g = json_encode($f, JSON_UNESCAPED_UNICODE | JSON_UNESCAPED_SLASHES); //print_r($f, true); echo "<b>6、Xuxiaobo::inputstrs(\"{$a}\", \"{$b}\", \"{$c}\", \"{$d}\", \"{$e}\") : </b>\n"; echo "<pre>".$g."</pre>\n\n"; $a = [ $arr1[random_int(0, count($arr1)-1)], $arr1[random_int(0, count($arr1)-1)], $arr1[random_int(0, count($arr1)-1)], $arr1[random_int(0, count($arr1)-1)], ]; $ainput = json_encode($a, JSON_UNESCAPED_UNICODE | JSON_UNESCAPED_SLASHES); $b = $xxb->inputarray($a); $c = json_encode($b, JSON_UNESCAPED_UNICODE | JSON_UNESCAPED_SLASHES); //print_r($b, true); echo "<b>7、Xuxiaobo::inputarray({$ainput}) : </b>\n"; echo "<pre>".$c."</pre>\n\n"; $a = [ random_int(1, 100), random_int(1, 100), random_int(1, 100), random_int(1, 100), random_int(1, 100), ]; $ainput = json_encode($a, JSON_UNESCAPED_UNICODE | JSON_UNESCAPED_SLASHES); $b = $xxb->sortarray($a); $c = json_encode($b, JSON_UNESCAPED_UNICODE | JSON_UNESCAPED_SLASHES); //print_r($b, true); echo "<b>8、Xuxiaobo::sortarray({$ainput}) : </b>\n"; echo "<pre>".$c."</pre>\n\n"; $a = [ $arr1[random_int(0, count($arr1)-1)], $arr1[random_int(0, count($arr1)-1)], $arr1[random_int(0, count($arr1)-1)], $arr1[random_int(0, count($arr1)-1)], $arr1[random_int(0, count($arr1)-1)], $arr1[random_int(0, count($arr1)-1)], $arr1[random_int(0, count($arr1)-1)], $arr1[random_int(0, count($arr1)-1)], $arr1[random_int(0, count($arr1)-1)], ]; $ainput = json_encode($a, JSON_UNESCAPED_UNICODE | JSON_UNESCAPED_SLASHES); $b = $xxb->sortarraycn($a); $c = json_encode($b, JSON_UNESCAPED_UNICODE | JSON_UNESCAPED_SLASHES); //print_r($b, true); echo "<b>9、Xuxiaobo::sortarraycn({$ainput}) : </b>\n"; echo "<pre>".$c."</pre>\n\n";
编译重启部分sh命令:
#! /bin/sh ps -ef |grep php-cgi |awk '{print $2}'|xargs kill -9 cd /home/xuxb/html/php_ext/php-7.3.9/ext/xuxiaobo /usr/local/php-7.3.9/bin/phpize ./configure --with-php-config=/usr/local/php-7.3.9/bin/php-config make && make install nohup /usr/local/php-7.3.9/bin/php-cgi -b 127.0.0.1:9001 -c /usr/local/php-7.3.9/php.ini &
效果:
PHP扩展开发
1、Xuxiaobo::extload() :
扩展xuxiaobo已经加载并运行
2、Xuxiaobo::hello() :
你好,世界
2.1、Xuxiaobo::hello("张若虚") :
你好,张若虚
3、Xuxiaobo::hellomust("于又微") :
你好,于又微
3.1、Xuxiaobo::hellomust() :
Warning: Xuxiaobo::hellomust() expects exactly 1 parameter, 0 given in /var/www/html/php_ext/xuxiaobo.php on line 31
4、Xuxiaobo::hellomuch("陆九渊") :
你好,陆九渊
4.1、Xuxiaobo::hellomuch("陆九渊", "卧槽") :
卧槽,陆九渊
5、Xuxiaobo::inputints(8, 4, 8, 2) :
[8,4,8,2]
6、Xuxiaobo::inputstrs("颜真卿", "苏颜回", "顾恺之", "卢照邻", "关山月") :
["颜真卿","苏颜回","顾恺之","卢照邻","关山月"]
7、Xuxiaobo::inputarray(["陆宇","颜真卿","颜真卿","李之仪"]) :
["陆宇","颜真卿","颜真卿","李之仪"]
8、Xuxiaobo::sortarray([90,4,9,20,23]) :
[4,9,20,23,90]
9、Xuxiaobo::sortarraycn(["温庭筠","梁思成","白居易","张九龄","高渐离","许唐","高渐离","李之仪","白居易"]) :
["许唐","温庭筠","梁思成","白居易","张九龄","高渐离","高渐离","李之仪","白居易"]
本文来自博客园,作者:河北大学-徐小波,转载请注明原文链接:https://www.cnblogs.com/xuxiaobo/p/18129206
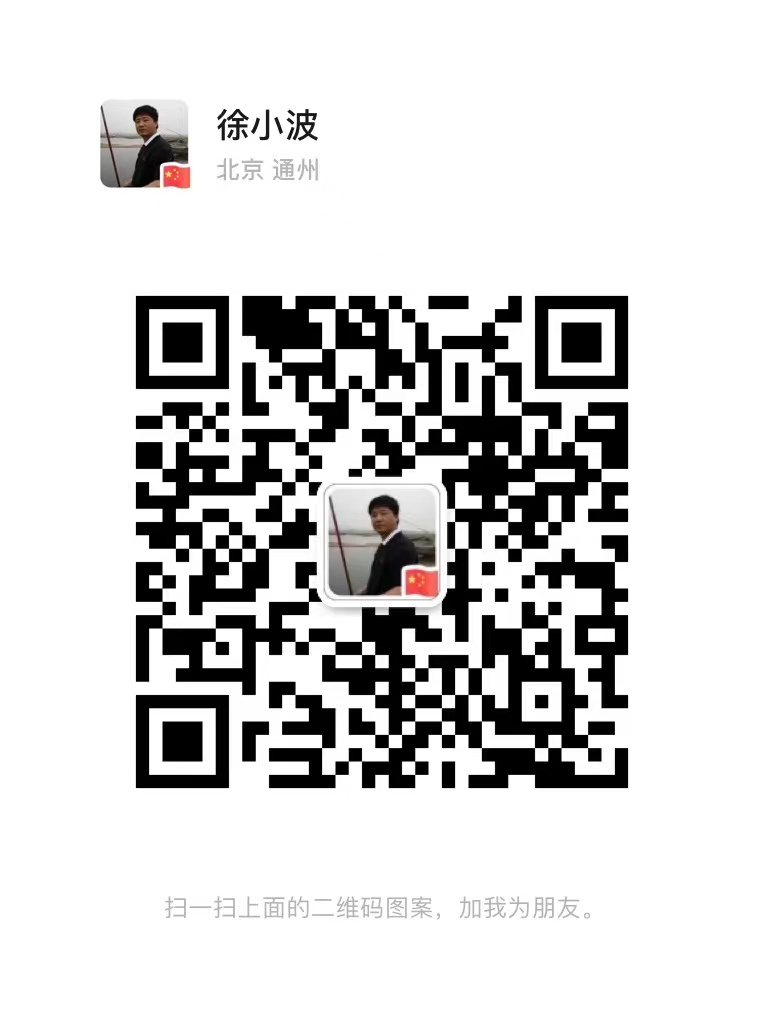