codebuider 使用方法
在使用之前我下载我的东东 去http://xuxianpeng.cnblogs.com/articles/373687.html看看
先看图:
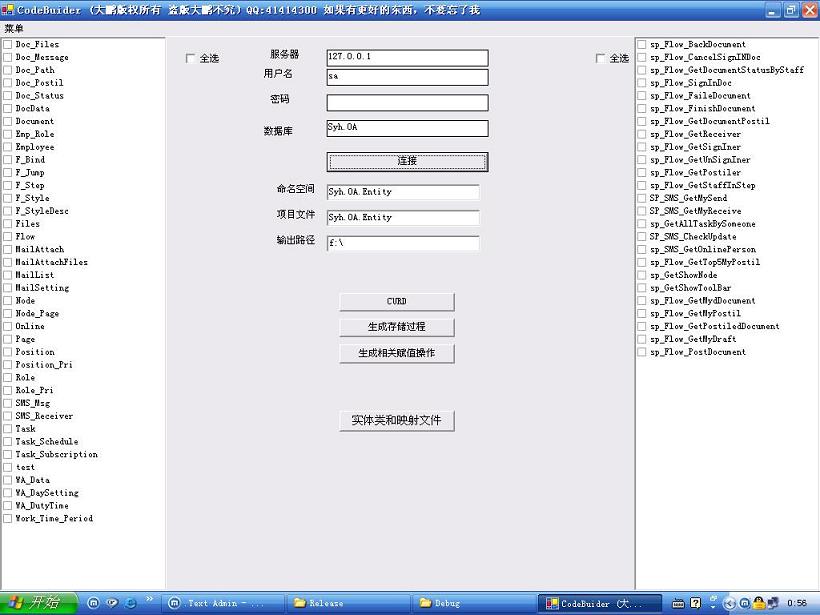

自动生成的实体类代码:
<?xml version="1.0" encoding="utf-8" ?>
<hibernate-mapping xmlns="urn:nhibernate-mapping-2.0">
<class name="Syh.OA.Entity.Employee, Syh.OA.Entity" table="Employee">
<id name="Staff_ID" column="Staff_ID" type="Int32"><generator class="identity" /></id>
<property name="Staff_Name" type="String(50)" column="Staff_Name" />
<property name="Password" type="String(255)" column="Password" />
<property name="RealName" type="String(50)" column="RealName" />
<property name="Sex" type="Boolean" column="Sex" />
<property name="Email" type="String(500)" column="Email" />
<property name="Status" type="String(50)" column="Status" />
<property name="RegistedDate" type="DateTime" column="RegistedDate" />
<property name="Phone" type="String(50)" column="Phone" />
<property name="Mobile" type="String(50)" column="Mobile" />
<property name="Birthday" type="DateTime" column="Birthday" />
<property name="Style" type="Int32" column="Style" />
<property name="PostionID" type="Int32" column="PostionID" />
</class>
</hibernate-mapping>
生成的业务操作类:
using System;
using System.Collections;
using System.Data;

namespace Syh.OA.BLL
{
public class Employee
{
public Employee()
{
}
/// <summary>
/// Employee_新增
/// </summary>
/// <param name="employee1 ">Employee_实体类</param>
/// <returns></returns>
public string Employee_Add(Syh.OA.Entity.Employee employee1)
{
string result = "";
try
{
DataAPI.ObjectBroker ob = new Syh.OA.DataAPI.ObjectBroker();
ob.Create(employee1);
result = SeNet.Base.Config.Well;
}
catch(System.Exception ex)
{
result = ex.Message;
}
return result;
}
/// <summary>
/// Employee_更新
/// </summary>
/// <param name="employee1 ">Employee_实体类</param>
/// <returns></returns>
public string Employee_Update(Syh.OA.Entity.Employee employee1)
{
string result = "";
try
{
DataAPI.ObjectBroker ob = new Syh.OA.DataAPI.ObjectBroker();
ob.Update(employee1);
result = SeNet.Base.Config.Well;
}
catch(System.Exception ex)
{
result = ex.Message;
}
return result;
}
/// <summary>
/// Employee_删除
/// </summary>
/// <param name="employee1 ">Employee_实体类</param>
/// <returns></returns>
public string Employee_Delete(Syh.OA.Entity.Employee employee1)
{
string result = "";
try
{
DataAPI.ObjectBroker ob = new Syh.OA.DataAPI.ObjectBroker();
ob.Delete(employee1);
result = SeNet.Base.Config.Well;
}
catch(System.Exception ex)
{
result = ex.Message;
}
return result;
}
/// <summary>
/// Employee_删除(按主键)
/// </summary>
/// <param name="staff_id">Employee_主键</param>
/// <returns></returns>
public string Employee_Delete(int staff_id)
{
Syh.OA.Entity.Employee employee1 = new Syh.OA.Entity.Employee();
string result = "";
try
{
DataAPI.ObjectBroker ob = new Syh.OA.DataAPI.ObjectBroker();
ob.Load(employee1,staff_id);
ob.Delete(employee1);
result = SeNet.Base.Config.Well;
}
catch(System.Exception ex)
{
result = ex.Message;
}
return result;
}
/// <summary>
/// Employee_按主键载入对象
/// </summary>
/// <param name="staff_id">Employee_主键</param>
/// <returns></returns>
public Syh.OA.Entity.Employee Employee_LoadByPK(int staff_id)
{
Syh.OA.Entity.Employee employee1 = new Syh.OA.Entity.Employee();
try
{
DataAPI.ObjectBroker ob = new Syh.OA.DataAPI.ObjectBroker();
ob.Load(employee1,staff_id);
}
catch(System.Exception ex)
{
employee1 = null;
throw new Exception("可能该编号的对象已经不存在!",ex);
}
return employee1;
}
/// <summary>
/// Employee_取得全部对象
/// </summary>
/// <returns>DataTable</returns>
public DataTable Employee_GetAll()
{
DataTable dt = null;
Syh.OA.Entity.Employee employee1 = new Syh.OA.Entity.Employee();
try
{
dt = Syh.OA.DataAPI.ObjectBroker.dtGetAll(employee1);
}
catch(System.Exception ex)
{
throw new Exception("Employee取得全部对象出错!",ex);;
}
return dt;
}
}
}
s
先看图:
自动生成的实体类代码:
1
using System;
2
namespace Syh.OA.Entity
3
{
4
public class Employee
5
{
6
public Employee()
7
{
8
}
9
private int _Staff_ID;
10
/// <summary>
11
/// 员工ID
12
/// </summary>
13
public int Staff_ID
14
{
15
get { return _Staff_ID;}
16
set { _Staff_ID =value; }
17
}
18
private string _Staff_Name;
19
/// <summary>
20
/// 员工名称
21
/// </summary>
22
public string Staff_Name
23
{
24
get { return _Staff_Name;}
25
set { _Staff_Name =value; }
26
}
27
private string _Password;
28
/// <summary>
29
/// 密码
30
/// </summary>
31
public string Password
32
{
33
get { return _Password;}
34
set { _Password =value; }
35
}
36
private string _RealName;
37
/// <summary>
38
/// 真实姓名
39
/// </summary>
40
public string RealName
41
{
42
get { return _RealName;}
43
set { _RealName =value; }
44
}
45
private bool _Sex;
46
/// <summary>
47
/// 性别
48
/// </summary>
49
public bool Sex
50
{
51
get { return _Sex;}
52
set { _Sex =value; }
53
}
54
private string _Email;
55
/// <summary>
56
/// 电子邮件
57
/// </summary>
58
public string Email
59
{
60
get { return _Email;}
61
set { _Email =value; }
62
}
63
private string _Status;
64
/// <summary>
65
/// 状态
66
/// </summary>
67
public string Status
68
{
69
get { return _Status;}
70
set { _Status =value; }
71
}
72
private DateTime _RegistedDate;
73
/// <summary>
74
/// 注册日期
75
/// </summary>
76
public DateTime RegistedDate
77
{
78
get { return _RegistedDate;}
79
set { _RegistedDate =value; }
80
} 90
private string _Phone;
91
/// <summary>
92
/// 电话
93
/// </summary>
94
public string Phone
95
{
96
get { return _Phone;}
97
set { _Phone =value; }
98
}
99
private string _Mobile;
100
/// <summary>
101
/// 手机
102
/// </summary>
103
public string Mobile
104
{
105
get { return _Mobile;}
106
set { _Mobile =value; }
107
}
108
private DateTime _Birthday;
109
/// <summary>
110
/// 生日
111
/// </summary>
112
public DateTime Birthday
113
{
114
get { return _Birthday;}
115
set { _Birthday =value; }
116
}
117
private int _Style;
118
/// <summary>
119
///
120
/// </summary>
121
public int Style
122
{
123
get { return _Style;}
124
set { _Style =value; }
125
}
126
private int _PostionID;
127
/// <summary>
128
/// 职位ID
129
/// </summary>
130
public int PostionID
131
{
132
get { return _PostionID;}
133
set { _PostionID =value; }
134
}
135
}
136
}
137
生成的配置文件:
2

3

4

5

6

7

8

9

10

11

12

13

14

15

16

17

18

19

20

21

22

23

24

25

26

27

28

29

30

31

32

33

34

35

36

37

38

39

40

41

42

43

44

45

46

47

48

49

50

51

52

53

54

55

56

57

58

59

60

61

62

63

64

65

66

67

68

69

70

71

72

73

74

75

76

77

78

79

80


91

92

93

94

95

96

97

98

99

100

101

102

103

104

105

106

107

108

109

110

111

112

113

114

115

116

117

118

119

120

121

122

123

124

125

126

127

128

129

130

131

132

133

134

135

136

137



















生成的业务操作类:





































































































































