vue3.0学习
ts代码自动编译
运行任务 tsc监视
//tsc --init
export导出
https://www.jianshu.com/p/541256d8abb3
webpack
vue3 之 Setup
vue3和vue2不同的地方就是不必写 data、methods、等代码块了
所有的东西都可以在 setup 中返回
setup 可以返回两种值:
1、返回对象,对象中的属性、方法都可以直接在模板中使用
vue基础语法
绑定数据
//绑定数据:
{{data}}
//绑定html
<span v-html='data'></span>
//pic 绑定属性
<img v-bind:src="data">
<img :src="data" :title="title">
//动态参数
:[type]="msg
//:key 不能丢
<div v-for="(item,index) in list" :key="index">
{{item}}---{{index}}
</div>
<template>
<a :title="msg" :[type]="msg">{{user.name}}</a>
</template>
<script>
export default {
data(){
return {
msg:"http://www.baidu.com",
user:{name:"tim"},
type:'href',
list:['张三','李四','王二麻子']
}
}
}
</script>
<style>
div {color: red;}
</style>
绑定事件
@click='setMsg()'
v-on:click='setMsg()'
methods:{
setMsg(){
this.user.name='haha';
}
}
获取dom节点
//原生js
document.querySelector('#username').val
<a href='' ref='age'>
this.$refs.age.value
数据绑定
v-mode
条件
v-if v-else v-else-if v-show
v-if 如果有多个标签需要放在 template里显示
dom操作
v-show 是css操作
计算属性
computed:{
testMsg(){
return this.msg+="11111";
}
}
watch监听数据变化
watch:{
msg:function (val) {
this.msg2=val+'new';
}
回车事件
@keyup.enter=''
monted页面加载的时候触发的方法
mountd(){
}
@chang监听事件变化
@chang=''
vue集成SaaS sacc
cnpm install -D sass-loader node-sass
<style lang="scss" scoped>
//scoped标识css局部作用
组件
组件定义
//1.定义组件
//1.导入组件
import home from './components/Home.vue'
//2.挂载组件
components:{
home
}
//3.使用组件
<home/>
父子组件传值
父向子传值
//子组件
<template>
home 组件 {{title}}
</template>
<script>
export default {
//props接收
props:["title"]
}
</script>
//父组件 this 传递整个父对象过去 子组件可以使用父对象的属性和方法
<home :title="tt" :home="this"/>
return{
tt:'ttttddddddddddtt'
}
//这种方式传递过去的值,子组件修改了变量的值的话不会影响父组件
//也就是不能双向绑定
//如果需要双向绑定参考6.26
父组件调用子组件
//通过ref
<Home ref="home"/>
//调用
this.$refs.home.属性
this.$refs.home.方法
子组件主动调用父组件
this.$parent.属性
this.$parent.方法
子组件给父组件传值
//父组件 自定义一个事件
<input @run-parent="GetMsg"></input>
//子组件 执行父组件的自定义事件
this.$emit('run-parent','data');
//传递前可以进行验证
emits:{
run-parent:()=>{
}
}
非父子组件的传值
//使用第三方组件 mitt
cnpm install --save mitt
//A组件
mitt.emit('事件1',value)
//B组件
mitt.on('事件1',(value)=>{ value })
组件上使用v-model实现双向绑定
子组件修改的时候,需要发出同步事件才可以
https://www.bilibili.com/video/BV1yt411e7xV?p=33&spm_id_from=pageDriver
//父组件里使用 v-model
<home v-model:title="tt" :home="this" ref="home" />
//子组件里
//使用 @input 事件
//可以写多个v-model
<input type="text" v-model="title" v-model="title2" v-model="title3" @input="$emit('update:title',$event.target.value)">
组件中间的值slot
<home>xxxx</home>
<slot></slot>
vue3.x非prop的attribute集成
一个非prop的attribute是指传向一个组件,但是该组件没有相应的props或emits定义的attribute.场景的实例包括class,style和id属性
会被子组件的根标签继承
可以通过设置 inheritAttrs:false 禁用默认继承
通过 子组件使用 v-bind="$attrs" 来指定继承
组件生命周期函数
keep-alive
当组件切换的时候,可以保持这些组件的状态,避免反复渲染导致的性能问题
在不同的路由切换的时候,想保持组件的状态也可以使用keep-alive
<keep-alive>
<home v-if="isshow"></home>
</keep-alive>
this.$nextTick
vue中可以把获取dom节点的代码放在mounted里面,但是如果是要在渲染完成后获取dom节点就需要用this.$nextTick
this.$nextTick(function(){
//仅在页面渲染完成以后调用
});
全局绑定组件,使用Axios和fetchjsonp请求真实api接口数据
axios不支持jsonp,
fetchjsonp支持
cnpm install axios --save
可以全局绑定组件,不用每个地方都引入
通过 this.Axios即可访问
import { createApp } from 'vue'
import App from './App.vue'
import './index.css'
import Axios from "axios";
// createApp(App).mount('#app')
var app=createApp(App);
app.config.globalProperties.Axios=Axios;
app.moun t('#app')
cnpm i fetch-jsonp --save
//防抖
mixins组件复用代码
全局使用mixin
Teleport将html挂载到指定的节点位置
composition API组合式API简单介绍
生命周期的hooks
setup
es6属性节点语法
toRefs--解构响应式对象数据
把一个响应式对象转化成普通对象,该普通对象的每个property都是一个ref和响应式对象peoperty一一对应
...运算符 可以合并对象
计算属性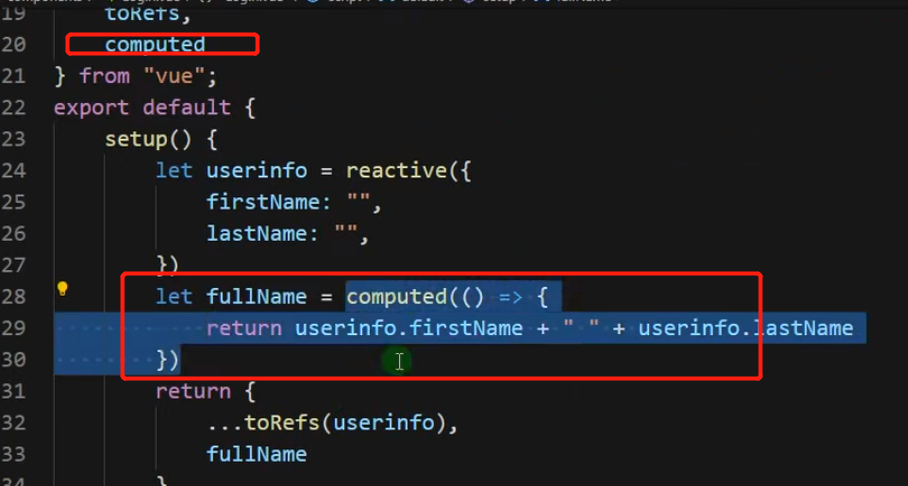
watch&watchEffect
Provider Inject
多层嵌套组件传值
无法进行双向绑定
父组件
子组件
可以双向绑定的写法
vue集成ts
vue add typescript
这样写会校验数据
路由
根据url自动挂载组件
cnpm install vue-router@next --save
配置路由
router.ts
动态路由:
get传值
业务逻辑路由跳转
HTML5 History模式
命名路由
路由重定向
redirect
路由别名
嵌套路由,父子嵌套
https://www.bilibili.com/video/BV1yt411e7xV?p=46&spm_id_from=pageDriver
状态管理模式Vuex
https://www.bilibili.com/video/BV1yt411e7xV?p=47&spm_id_from=pageDriver
serverless框架
开发者不用过多考虑服务器的问题