LeetCode - 872. Leaf-Similar Trees
Consider all the leaves of a binary tree. From left to right order, the values of those leaves form a leaf value sequence.
For example, in the given tree above, the leaf value sequence is (6, 7, 4, 9, 8)
.
Two binary trees are considered leaf-similar if their leaf value sequence is the same.
Return true
if and only if the two given trees with head nodes root1
and root2
are leaf-similar.
Note:
- Both of the given trees will have between
1
and100
nodes.
先序遍历,找到叶子节点依次比较
/** * Definition for a binary tree node. * public class TreeNode { * int val; * TreeNode left; * TreeNode right; * TreeNode(int x) { val = x; } * } */ class Solution { private List<TreeNode> nodes1 = new ArrayList<>(); private List<TreeNode> nodes2 = new ArrayList<>(); public boolean leafSimilar(TreeNode root1, TreeNode root2) { frontTraversal(root1, nodes1); frontTraversal(root2, nodes2); int len1 = nodes1.size(); int len2 = nodes2.size(); if (len1 != len2) return false; for (int i=0; i<len1; i++) { if (nodes1.get(i).val != nodes2.get(i).val) return false; } return true; } public void frontTraversal(TreeNode root, List<TreeNode> nodes) { if (root == null) return; if (root.left != null) frontTraversal(root.left, nodes); if (root.left == null && root.right == null) nodes.add(root); if (root.right != null) frontTraversal(root.right, nodes); } }
作者:Pickle
声明:对于转载分享我是没有意见的,出于对博客园社区和作者的尊重一定要保留原文地址哈。
致读者:坚持写博客不容易,写高质量博客更难,我也在不断的学习和进步,希望和所有同路人一道用技术来改变生活。觉得有点用就点个赞哈。
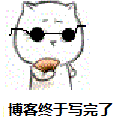

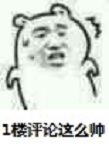
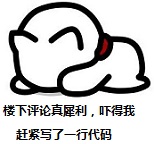
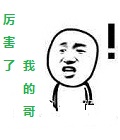
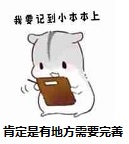

