LeetCode - 2. Add Two Numbers
You are given two non-empty linked lists representing two non-negative integers. The digits are stored in reverse order and each of their nodes contain a single digit. Add the two numbers and return it as a linked list.
You may assume the two numbers do not contain any leading zero, except the number 0 itself.
Input: (2 -> 4 -> 3) + (5 -> 6 -> 4)
Output: 7 -> 0 -> 8
调整顺序即可
/** * Definition for singly-linked list. * public class ListNode { * int val; * ListNode next; * ListNode(int x) { val = x; } * } */ class Solution { public ListNode addTwoNumbers(ListNode l1, ListNode l2) { String s1 = toStr(l1); String s2 = toStr(l2); return toNode(addTowStr(s1, s2)); } private String toStr(ListNode node) { StringBuilder sr = new StringBuilder(); if (node == null) return sr.toString(); while (node != null) { sr.append(node.val); node = node.next; } return sr.toString(); } private ListNode toNode(String str) { if (str == null) return null; ListNode cur = new ListNode(str.charAt(0)-'0'); ListNode head = cur; for (int i=1; i<str.length(); i++) { cur.next = new ListNode(str.charAt(i)-'0'); cur = cur.next; } return head; } private String addTowStr(String s1, String s2) { if (s1 == null || s1.length() <= 0 || s1.equals("0")) return s2; if (s2 == null || s2.length() <= 0 || s2.equals("0")) return s1; if (s1.length() < s2.length()) { String t = s1; s1 = s2; s2 = t; } int minLen = s2.length(); int maxLen = s1.length(); int over = 0; StringBuilder ret = new StringBuilder(); for (int i=0,j=0; i<=minLen-1 && j<=minLen; i++,j++) { int sum = (s1.charAt(j)-'0') + (s2.charAt(i)-'0') + over; if (sum < 10) { ret.append(sum+""); over = 0; } else { ret.append((sum % 10)+""); over = sum / 10; } } for (int i=minLen; i<maxLen; i++) { int sum = (s1.charAt(i)-'0') + over; if (sum < 10) { ret.append(sum+""); over = 0; } else { over = sum / 10; ret.append((sum % 10)+""); } } if (over > 0) ret.append(over); return ret.toString(); } }
作者:Pickle
声明:对于转载分享我是没有意见的,出于对博客园社区和作者的尊重一定要保留原文地址哈。
致读者:坚持写博客不容易,写高质量博客更难,我也在不断的学习和进步,希望和所有同路人一道用技术来改变生活。觉得有点用就点个赞哈。
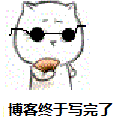

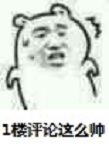
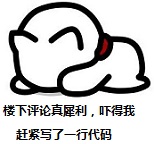
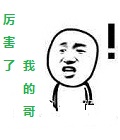
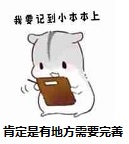

