LeetCode——Best Time to Buy and Sell Stock III
Description:
Say you have an array for which the ith element is the price of a given stock on day i.
Design an algorithm to find the maximum profit. You may complete at most two transactions.
Note:
You may not engage in multiple transactions at the same time (ie, you must sell the stock before you buy again).
给出股价表,最多两次交易,求出最大收益。
动态规划,分解成两个子问题,在第i天之前(包括第i天)的最大收益,在第i天之后(包括第i天)的最大收益,这样就变成了求一次交易的最大收益了,同系列问题I,
最后遍历一次求子问题最大收益之和的最大值,就是最后的解。时间复杂度O(n),空间复杂度O(n)
public class Solution { public int maxProfit(int[] prices) { int len = prices.length; if(len == 0) return 0; int[] leftProfit = new int[len]; int[] rightProfit = new int[len]; Arrays.fill(leftProfit, 0); Arrays.fill(rightProfit, 0); //计算左边的最大收益,从前向后找 int lowestPrice = prices[0]; for(int i=1; i<len; i++) { lowestPrice = min(lowestPrice, prices[i]); leftProfit[i] = max(leftProfit[i-1], prices[i] - lowestPrice); } //计算右边的最大收益,从后往前找 int highestPrice = prices[len-1]; for(int i=len-2; i>=0; i--) { highestPrice = max(highestPrice, prices[i]); rightProfit[i] = max(rightProfit[i+1], highestPrice-prices[i]); } int maxProfit = leftProfit[0] + rightProfit[0]; //遍历找出经过最多两次交易后的最大了收益 for(int i=1; i<len; i++) { maxProfit = max(maxProfit, leftProfit[i] + rightProfit[i]); } return maxProfit; } public int min(int a, int b) { return a > b ? b : a; } public int max(int a, int b) { return a > b ? a : b; } }
作者:Pickle
声明:对于转载分享我是没有意见的,出于对博客园社区和作者的尊重一定要保留原文地址哈。
致读者:坚持写博客不容易,写高质量博客更难,我也在不断的学习和进步,希望和所有同路人一道用技术来改变生活。觉得有点用就点个赞哈。
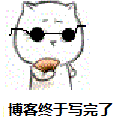

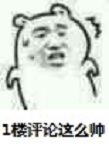
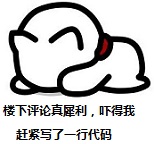
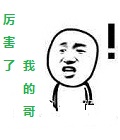
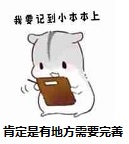

