设计模式——原型模式
当创建给定的实例的过程很昂贵或非常复杂的时候,就使用原型模式来拷贝,这种方式在创建对象要耗费很多资源的时候效率提升显著。
实现深度克隆有两种方法,一种是实现Cloneable接口,重写clone()方法。另一种是通过序列化反序列化来获取对象的拷贝。
看一个介绍:http://blog.csdn.net/zhengzhb/article/details/7393528
看一个Demo:
public class Prototype implements Cloneable , Serializable{ String name; Date date; public Prototype(String name, Date date) { this.name = name; this.date = date; } public Prototype() { } public void setDate(Date date) { this.date = date; }public void setName(String name) { this.name = name; } @Override protected Object clone() throws CloneNotSupportedException { return super.clone(); } protected Object deepClone() throws CloneNotSupportedException { Object obj = this.clone(); Prototype p = (Prototype) obj; p.date = (Date) this.date.clone(); return obj; } } public class ClientOne { public static void shallowClone() throws Exception { Date date = new Date(12356565656L); Prototype p1 = new Prototype("原型对象", date); Prototype p2 = (Prototype) p1.clone(); System.out.println(p1); System.out.println(p1.date); //p1.setDate(new Date(323232326565656L)); date.setTime(36565656562626L); System.out.println(p2); System.out.println(p2.date); } public static void deepClone() throws Exception { Date date = new Date(12356565656L); Prototype p1 = new Prototype("原型对象",date); Prototype p2 = (Prototype) p1.deepClone(); System.out.println(p1); System.out.println(p1.date); date.setTime(36565656562626L); System.out.println(p2); System.out.println(p2.date); } public static void deepCloneSerialize() throws Exception { Date date = new Date(12356565656L); Prototype p1 = new Prototype("原型对象",date); //通过序列化反序列化来新建一个对象 ByteArrayOutputStream bos = new ByteArrayOutputStream(); ObjectOutputStream oos = new ObjectOutputStream(bos); oos.writeObject(p1); byte[] bytes = bos.toByteArray(); ByteArrayInputStream bis = new ByteArrayInputStream(bytes); ObjectInputStream ois = new ObjectInputStream(bis); Prototype p2 = (Prototype) ois.readObject(); System.out.println(p1); System.out.println(p1.date); date.setTime(36565656562626L); System.out.println(p2); System.out.println(p2.date); } public static void main(String[] args) throws Exception { System.out.println("Shallow clone:"); shallowClone(); System.out.println("Deep clone:"); deepClone(); System.out.println("Deep clone serialize:"); deepCloneSerialize(); System.exit(0); } }
作者:Pickle
声明:对于转载分享我是没有意见的,出于对博客园社区和作者的尊重一定要保留原文地址哈。
致读者:坚持写博客不容易,写高质量博客更难,我也在不断的学习和进步,希望和所有同路人一道用技术来改变生活。觉得有点用就点个赞哈。
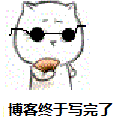

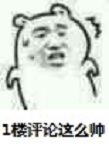
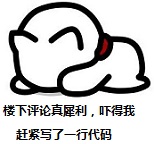
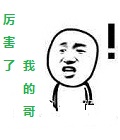
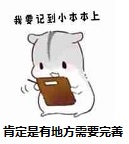

