LeetCode - Merge Two Sorted Lists
依然是链表的简单操作,把两个链表按大小顺序和成一个链表,但是还是要注意细节。下面是效率不高但是简单易懂的一种解法。需要注意两个链表都为空的情况。
/** * Definition for singly-linked list. * public class ListNode { * int val; * ListNode next; * ListNode(int x) { * val = x; * next = null; * } * } */ public class Solution { public ListNode mergeTwoLists(ListNode l1, ListNode l2) { if(l1 == null && l2 == null) return null; if(l1==null && l2!=null) return l2; if(l1!=null && l2==null) return l1; ArrayList<Integer> set = new ArrayList<>(); ListNode p = new ListNode(1), q = new ListNode(1); p = l1; q = l2; while(p != null) { set.add(p.val); p = p.next; } while(q != null) { set.add(q.val); q = q.next; } Collections.sort(set); ListNode ans = null; ListNode head = new ListNode(1); for(int i=0; i<set.size(); i++) { if(i == 0) { ans = new ListNode(set.get(i)); head = ans; } else { ans.next = new ListNode(set.get(i)); ans = ans.next; } } return head; } }
作者:Pickle
声明:对于转载分享我是没有意见的,出于对博客园社区和作者的尊重一定要保留原文地址哈。
致读者:坚持写博客不容易,写高质量博客更难,我也在不断的学习和进步,希望和所有同路人一道用技术来改变生活。觉得有点用就点个赞哈。
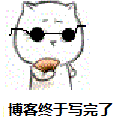

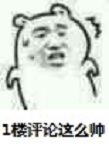
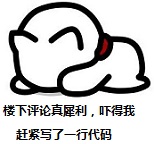
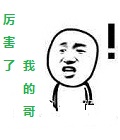
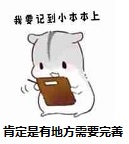

