/**
* 将字符串采用编码<b>_encoding</b>转化为字节数组
*
* @param _str 字符串
* @param _encoding 编码方式
* @throws ParamValidateException 如果编码方式不支持时掷出
* @return 字节数组
*/
public static byte[] toBytes(String _str, String _encoding) throws IllegalArgumentException{
if( _str == null) {
return null;
}
byte[] b = null;
try {
b = _str.getBytes(_encoding);
} catch (Exception e) {
throw new IllegalArgumentException("不支持的编码方式:" + _encoding);
}
return b;
}
/**
* 将双精浮点数代表的金额转化中文大写形式
*
* @param _dMoney 代表双精浮点数的金额
* @return 金额的中文大写形式,如果输入参数<b>dMoney</b>大于10^8或小于0.01返回空串。
*/
public static String toChinese(double _dMoney) {
String[] strArr = { "零", "壹", "贰", "叁", "肆", "伍", "陆", "柒", "捌", "玖" };
String[] strArr1 = { "分", "角", "圆", "拾", "佰", "仟", "万", "拾", "佰", "仟" };
String[] strArr2 = new String[10];
String sRtn = "";
int iTmp;
double dTmp;
try {
_dMoney += 0.001;
if ((_dMoney >= 100000000) || (_dMoney < 0.01)) {
sRtn = "";
} else {
for (int i = 0; i < 10; i++) {
dTmp = _dMoney / Math.pow(10, 7 - i);
iTmp = (new Double(dTmp)).intValue();
_dMoney -= iTmp * Math.pow(10, 7 - i);
if (iTmp != 0) {
strArr2[i] = strArr[iTmp] + strArr1[9 - i];
} else {
strArr2[i] = "";
}
}
boolean bFlag = false;
for (int i = 0; i < 10; i++) {
if (!"".equals(strArr2[i])) {
sRtn += strArr2[i];
bFlag = true;
} else {
if (i == 3) {
sRtn += "万";
bFlag = true;
} else if (i == 7) {
sRtn += "圆";
bFlag = true;
} else if (bFlag) {
sRtn += "零";
bFlag = false;
}
}
}
if (sRtn.startsWith("万")) {
sRtn = sRtn.substring(1, sRtn.length());
}
if (sRtn.startsWith("圆")) {
sRtn = sRtn.substring(1, sRtn.length());
}
while (sRtn.startsWith("零")) {
sRtn = sRtn.substring(1, sRtn.length());
}
if (sRtn.lastIndexOf("零") == (sRtn.length() - 1)) {
sRtn = sRtn.substring(0, sRtn.length() - 1);
}
if (sRtn.startsWith("圆")) {
sRtn = sRtn.substring(1, sRtn.length());
}
iTmp = sRtn.indexOf("圆");
if (iTmp != -1) {
if ("零".equals(sRtn.substring(iTmp - 1, iTmp))) {
sRtn =
sRtn.substring(0, iTmp - 1)
+ sRtn.substring(iTmp, sRtn.length());
}
}
iTmp = sRtn.indexOf("万");
if (iTmp != -1) {
if ("零".equals(sRtn.substring(iTmp - 1, iTmp))) {
sRtn =
sRtn.substring(0, iTmp - 1)
+ sRtn.substring(iTmp, sRtn.length());
}
}
while (sRtn.startsWith("零")) {
sRtn = sRtn.substring(1, sRtn.length());
}
sRtn += "整";
}
} catch (Exception ex) {
}
return sRtn;
}
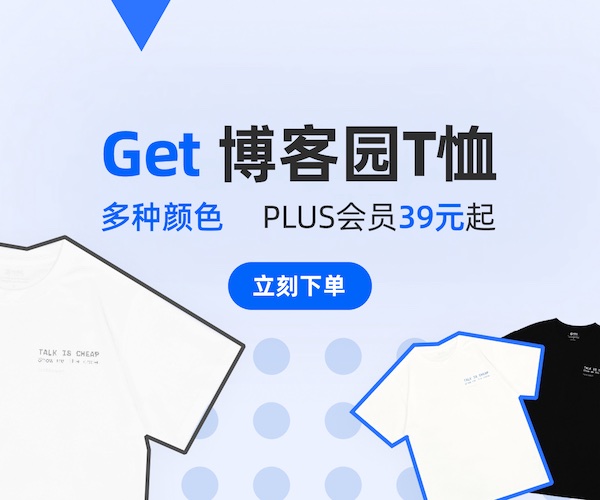