普通 Javaweb项目模板的搭建
普通 Javaweb项目模板的搭建
1、 创建一个web项目模板的maven项目
2、配置 Tomcat 服务器
3、先测试一下该空项目
4、注入 maven 依赖
5、创建项目的包结构
6、编写基础公共类
6.1、 数据库配置文件(db.properties)
6.2、 编写数据库的公共类
1、 创建一个web项目模板的maven项目
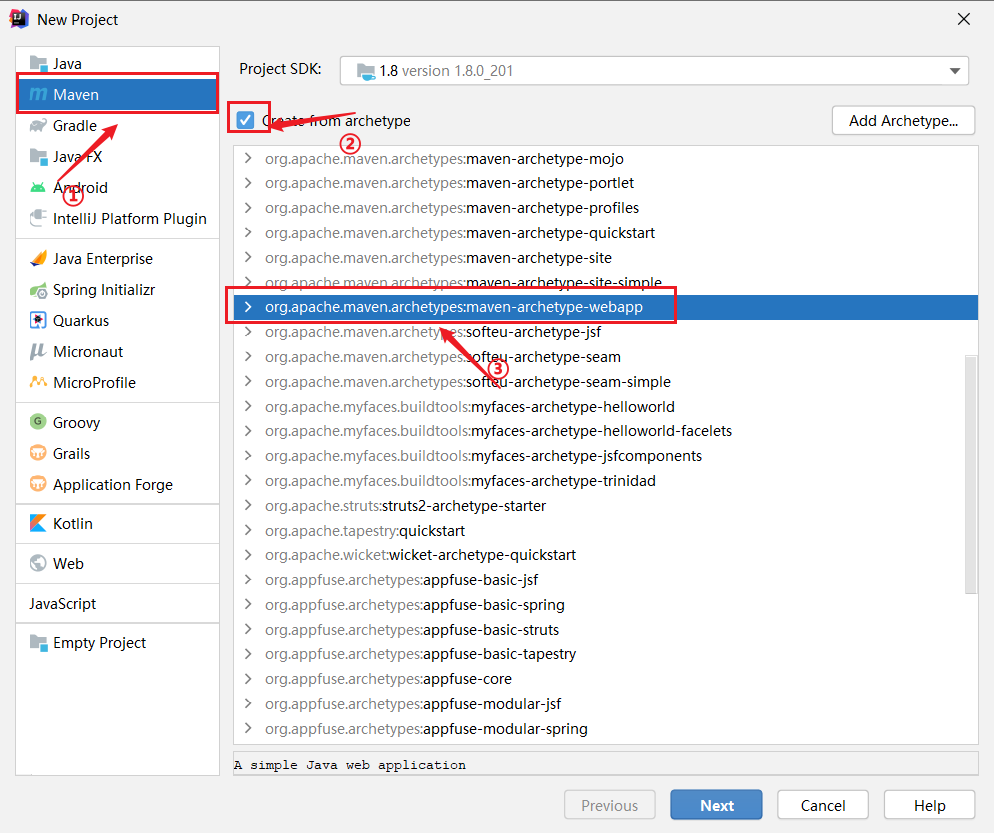
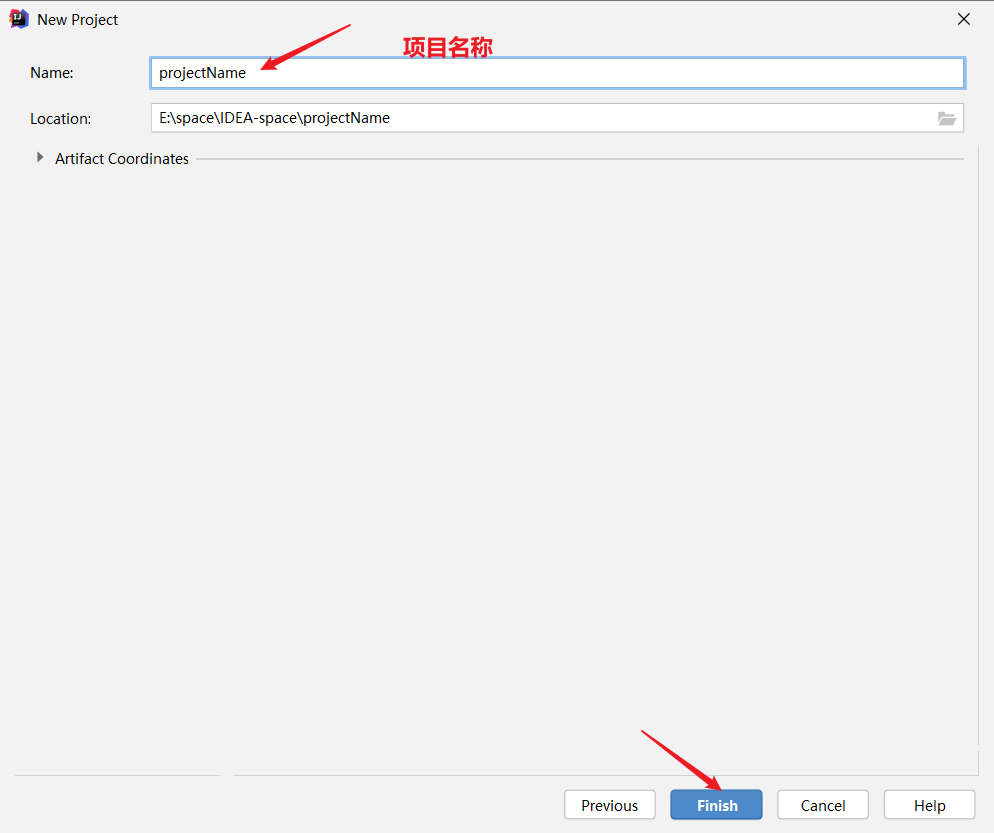
现在就是一个web项目了。
2、 配置 Tomcat 服务器
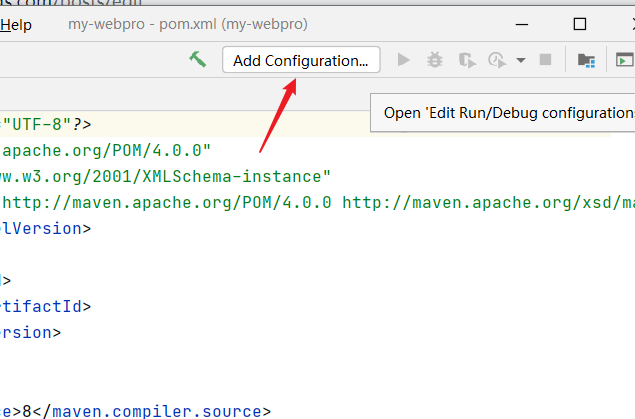
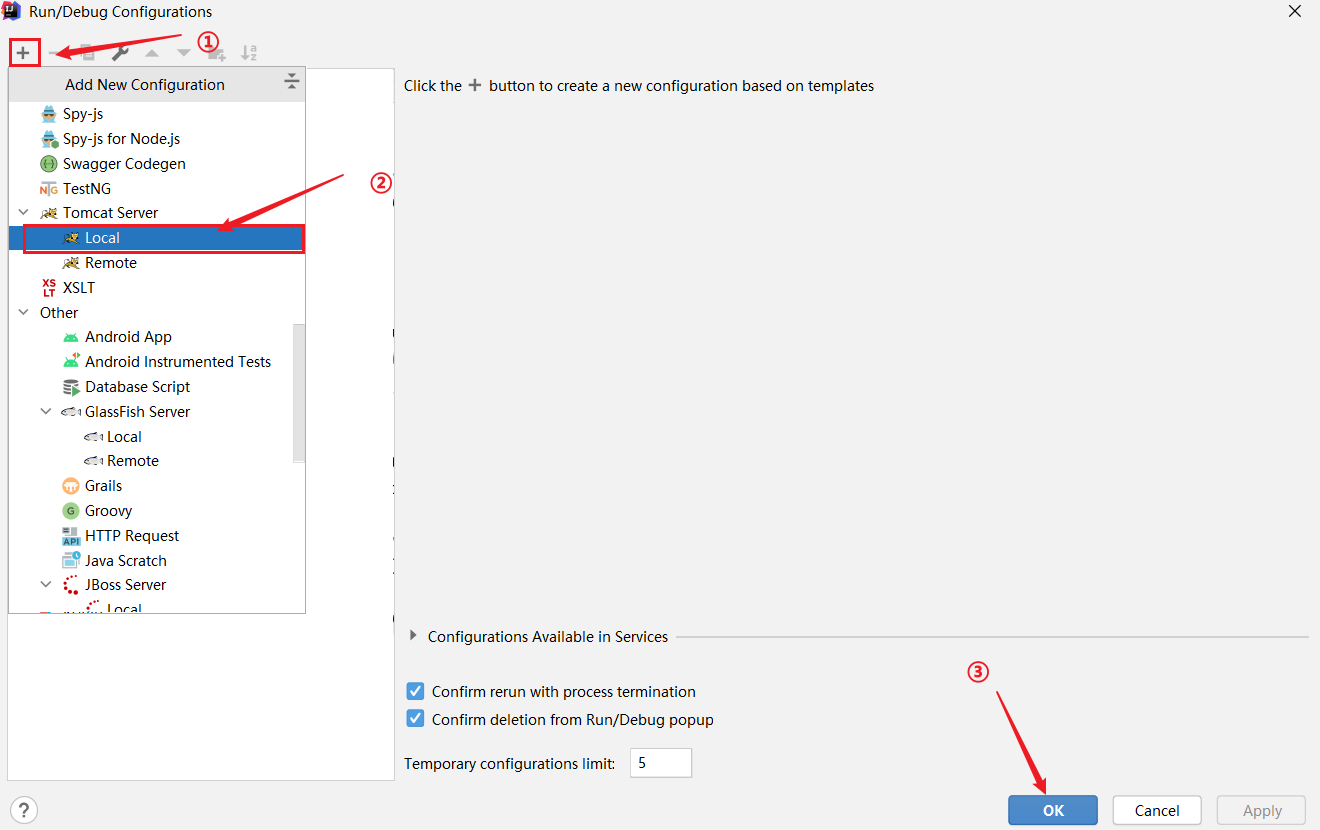
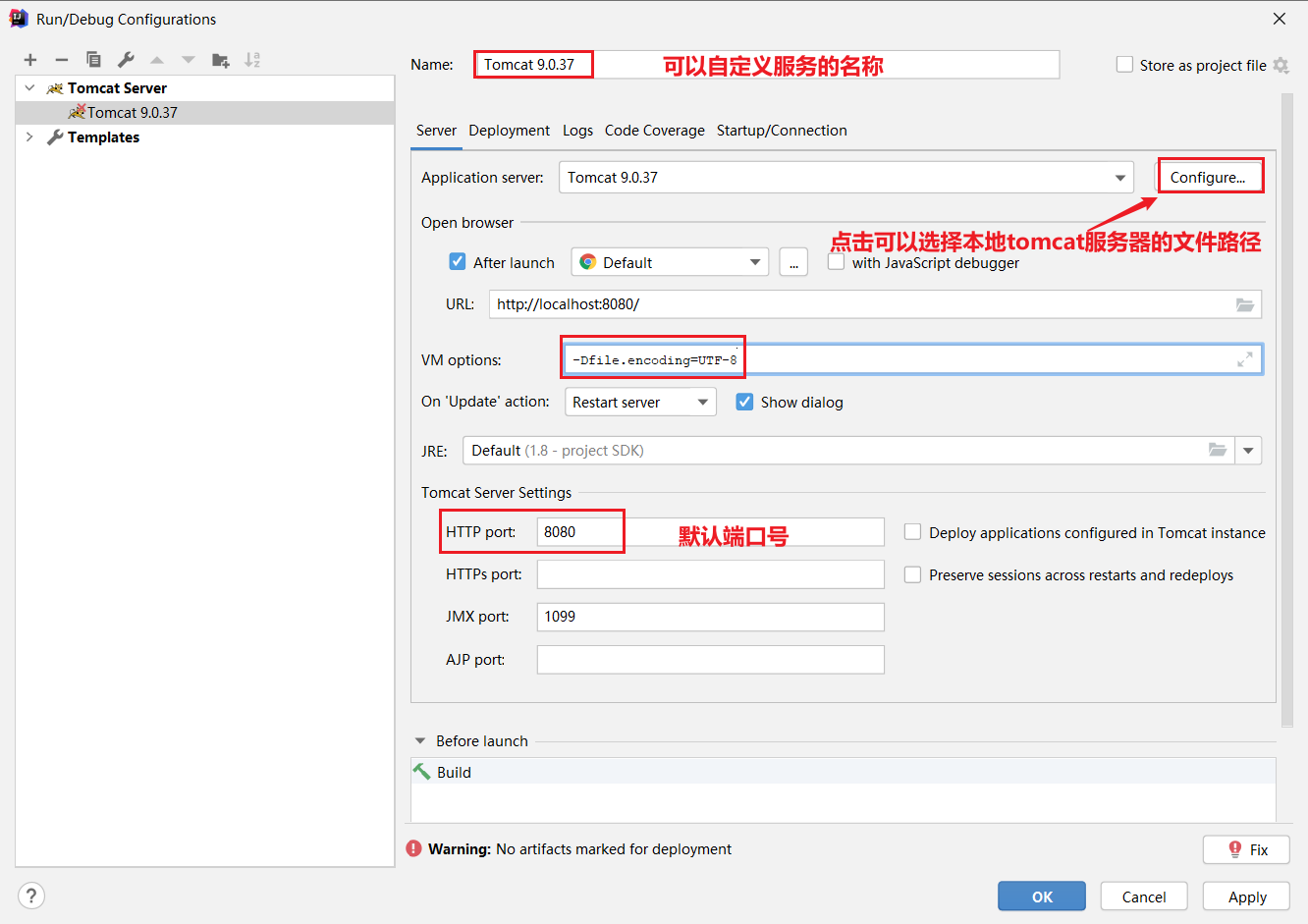
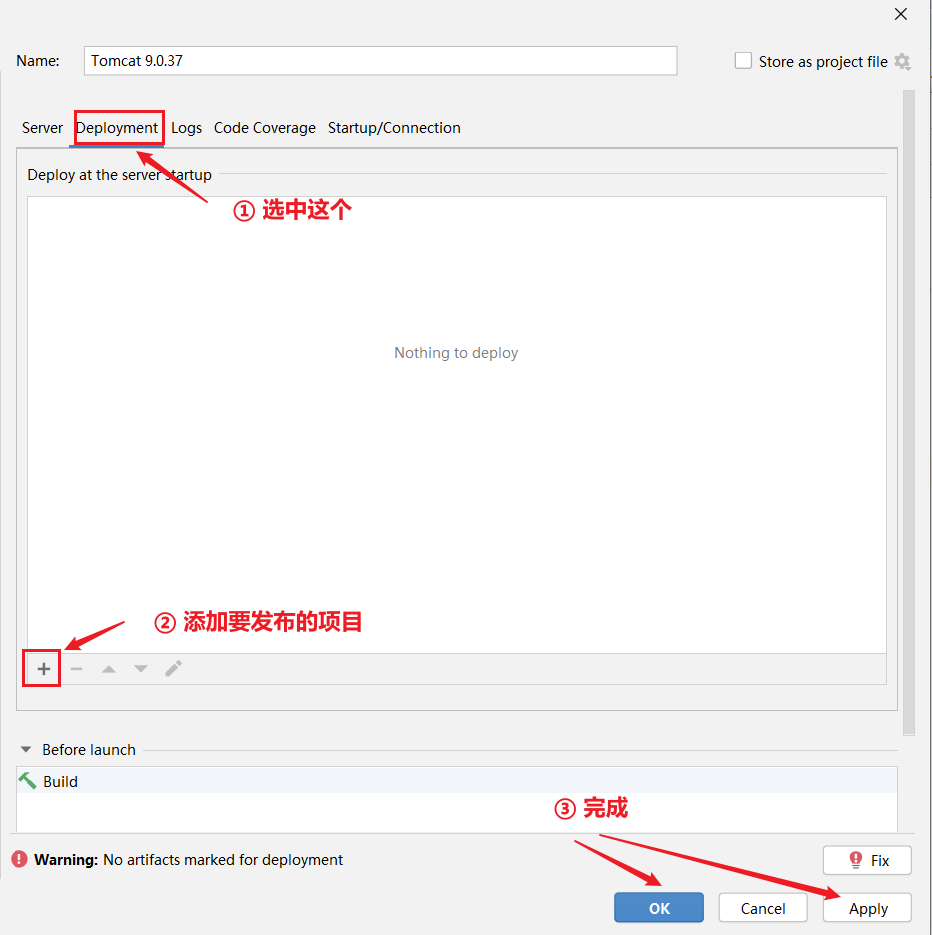
现在就配置好 Tomcat 服务器了!
3、先测试一下该空项目
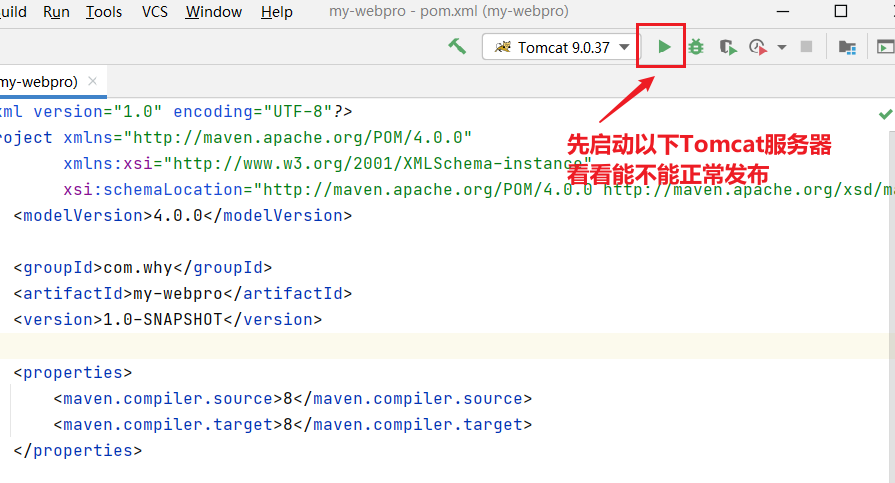
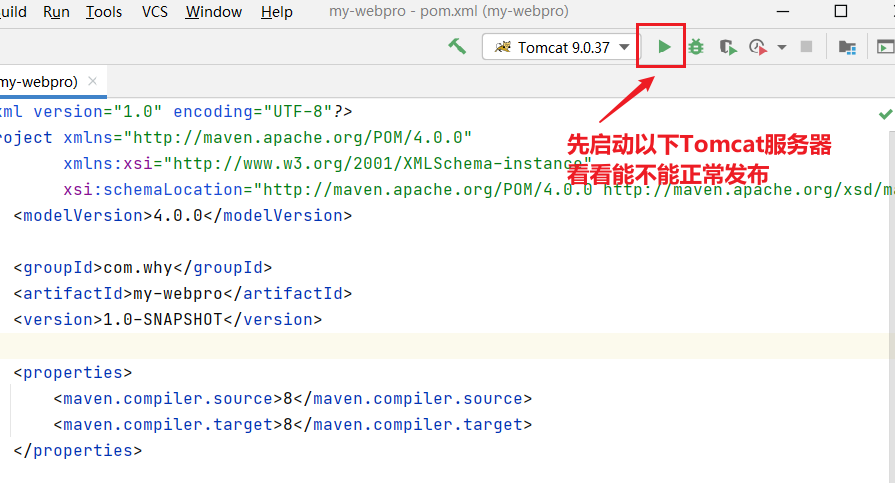
如果能够正常访问到页面,则服务器配置没有什么问题;否则,则出现问题。那就得另行解决了。。。。
4、 注入 maven 依赖
web项目需要的jar包:jsp,servlet,mysql驱动,jdbc,jstl,standard .....
<!-- servlet依赖 -->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>3.1.0</version>
<scope>provided</scope>
</dependency>
<!-- JSP依赖 -->
<dependency>
<groupId>javax.servlet.jsp</groupId>
<artifactId>javax.servlet.jsp-api</artifactId>
<version>2.3.1</version>
<scope>provided</scope>
</dependency>
<!--JSTL表达式依赖-->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>jstl</artifactId>
<version>1.2</version>
</dependency>
<!--standard标签库-->
<dependency>
<groupId>taglibs</groupId>
<artifactId>standard</artifactId>
<version>1.1.2</version>
</dependency>
<!--jdbc -->
<dependency>
<groupId>org.clojure</groupId>
<artifactId>java.jdbc</artifactId>
<version>0.7.11</version>
</dependency>
<!--junit-->
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.12</version>
</dependency>
<!--连接mysql数据库依赖-->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>5.1.47</version>
</dependency>
<!--fastjson依赖-处理json字符串-->
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>fastjson</artifactId>
<version>1.2.62</version>
</dependency>
5、 创建项目的包结构
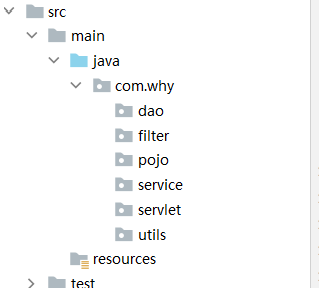
提前建好包,结构清晰,逻辑分明,方便代码的编写。。。。。
6、 编写基础公共类
6.1、 数据库配置文件(db.properties)
#加载驱动
driver=com.mysql.jdbc.Driver
#加载数据库
#dbname是要连接的数据库名称
url=jdbc:mysql://localhost:3306/dbname?useUnicode=true&characterEncoding=utf-8
#用户名
user=root
#密码
password=123456
6.2、 编写数据库的公共类
public class DBUtil {
//静态代码块,在类加载的时候执行
static{
init();
}
private static String driver;
private static String url;
private static String user;
private static String password;
//初始化连接参数,从配置文件里获得
public static void init(){
Properties params=new Properties();
String configFile = "db.properties";
InputStream is= DBUtil.class.getClassLoader().getResourceAsStream(configFile);
try {
params.load(is);
} catch (IOException e) {
e.printStackTrace();
}
driver=params.getProperty("driver");
url=params.getProperty("url");
user=params.getProperty("user");
password=params.getProperty("password");
}
/*
* 获得数据库连接
* */
public static Connection getConnection(){
Connection connection = null;
try {
Class.forName(driver);
connection = DriverManager.getConnection(url, user, password);
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return connection;
}
/*
* 查询操作
* params 是prepareStatement所执行sql语句中 占位符 ? 的值
* */
public static ResultSet execute(Connection connection, PreparedStatement pstm, ResultSet rs,
String sql, Object[] params) throws Exception{
pstm = connection.prepareStatement(sql);
for(int i = 0; i < params.length; i++){
pstm.setObject(i+1, params[i]);
}
rs = pstm.executeQuery();
return rs;
}
/*
* 更新操作
* */
public static int execute(Connection connection,PreparedStatement pstm,
String sql,Object[] params) throws Exception{
int updateRows = 0;
pstm = connection.prepareStatement(sql);
for(int i = 0; i < params.length; i++){
pstm.setObject(i+1, params[i]);
}
updateRows = pstm.executeUpdate();
return updateRows;
}
/*
* 资源回收
* */
public static boolean closeResource(Connection connection,PreparedStatement pstm,ResultSet rs){
boolean flag = true;
if(rs != null){
try {
rs.close();
rs = null;//GC回收
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
flag = false;
}
}
if(pstm != null){
try {
pstm.close();
pstm = null;//GC回收
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
flag = false;
}
}
if(connection != null){
try {
connection.close();
connection = null;//GC回收
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
flag = false;
}
}
return flag;
}
至此web项目的模板就已经构建的差不多了.....
不知道怎么回事,通过先创建一个普通 maven 项目,然后再将其变为一个 web 项目的方式,一直报 找不到过滤器 这个错误;必须通过使用模板构建的方式才能够使用过滤器!