专业课图谱
Black Hat Python
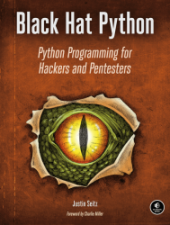
- Download Chapter 7: GitHub Command and Control
- Download the resources and code samples from the book
- Download the Kali Linux virtual machine used in the book (i486)
“The difference between script kiddies and professionals is the difference between merely using other people's tools and writing your own.”
—Charlie Miller, from the Foreword
Featured in ZDNet's list of "Cybersecurity reads for every hacker's bookshelf"
When it comes to creating powerful and effective hacking tools, Python is the language of choice for most security analysts. But just how does the magic happen?
In Black Hat Python, the latest from Justin Seitz (author of the best-selling Gray Hat Python), you’ll explore the darker side of Python’s capabilities—writing network sniffers, manipulating packets, infecting virtual machines, creating stealthy trojans, and more. You’ll learn how to:
- Create a trojan command-and-control using GitHub
- Detect sandboxing and automate common malware tasks, like keylogging and screenshotting
- Escalate Windows privileges with creative process control
- Use offensive memory forensics tricks to retrieve password hashes and inject shellcode into a virtual machine
- Extend the popular Burp Suite web-hacking tool
- Abuse Windows COM automation to perform a man-in-the-browser attack
- Exfiltrate data from a network most sneakily
Insider techniques and creative challenges throughout show you how to extend the hacks and how to write your own exploits.
When it comes to offensive security, your ability to create powerful tools on the fly is indispensable. Learn how in Black Hat Python.
Uses Python 2
Introduction
Chapter 1: Setting Up Your Python Environment
Chapter 2: The Network: Basics
Chapter 3: The Network: Raw Sockets and Sniffing
Chapter 4: Owning the Network with Scapy
Chapter 5: Web Hackery
Chapter 6: Extending Burp Proxy
Chapter 7: GitHub Command and Control
Chapter 8: Common Trojaning Tasks on Windows
Chapter 9: Fun With Internet Explorer
Chapter 10: Windows Privilege Escalation
Chapter 11: Automating Offensive Forensics
Index
View the detailed Table of Contents (PDF)
View the Index (PDF)
"Another incredible Python book. With a minor tweak or two many of these programs will have at least a ten year shelf life, and that is rare for a security book."
—Stephen Northcutt, founding president of the SANS Technology Institute
"A great book using Python for offensive security purposes."
—Andrew Case, Volatility core developer and coauthor of The Art of Memory Forensics
"If you truly have a hacker’s mindset, a spark is all you need to make it your own and do something even more amazing. Justin Seitz offers plenty of sparks."
—Ethical Hacker (Read More)
"Whether you're interested in becoming a serious hacker/penetration tester or just want to know how they work, this book is one you need to read. Intense, technically sound, and eye-opening."
—Sandra Henry-Stocker, IT World (Read More)
"Definitely a recommended read for the technical security professional with some basic previous exposure to Python."
—Richard Austin, IEEE Cipher (Read More)
"A well-written book that will put you on track to being able to write powerfuland potentially scarytools. It’s up to you to use them for good."
—Steve Mansfield-Devine, editor of Elsevier's Network Security Newsletter
"A well implemented read with lots of good ideas for fun offensive Python projects. So enjoy, and don't forget it's all about the code!"
—Dan Borges, LockBoxx
"A useful eye-opener."
—MagPi Magazine
The download location for Kali Linux has changed. You can grab various virtual machines here:
https://www.offensive-security.com/kali-linux-vmware-arm-image-download/
import math
dir(math) 列出math所有的...
help(math.sin) q #退出
exit() #退出命令行
---------------二进制存储----------------
>>> 1.1+2.2
3.3000000000000003
因为二进制只能表示2的n次方的数,n可以取负值,3.3无法用2的n次方的数组合计算出来,所以无法精确表示:3.3=1*2+1*1+0*1/2+1*1/4+0*1/8+0*1/16+1*1/32+...
其中分式的分母只能是2的倍数(二进制所限),3.3的二进制表示是11.01001.....
有些数比如1/3就无法精确计算,只能无限逼近
bin()、oct()、hex()的返回值均为字符串,且分别带有0b、0o、0x前缀
---------------**幂次运算------------------------------
2**2**3 # 2的2次方的3次方 =256
‘abc’**3 # abc的三次方 = abcabcabc或者‘abcabcabc’
-------------and or not--逻辑与或非 false true-----------优先级:非与或(由高到低----
123and456 #456
123or456 #123
and: 如果所有值都为真,那么 and 返回最后一个值。
如果某个值为假,则 and 返回第一个假值。
or 如果有一个值为真,or 立刻返回该值。
如果所有的值都为假,or 返回最后一个假值。
通俗讲就是程序读到哪里能够被满足然后就可以结束,然后返回最后的满足值。
>>> help('and')
Boolean operations
******************
or_test ::= and_test | or_test "or" and_test
and_test ::= not_test | and_test "and" not_test
not_test ::= comparison | "not" not_test
In the context of Boolean operations, and also when expressions are
used by control flow statements, the following values are interpreted
as false: "False", "None", numeric zero of all types, and empty
strings and containers (including strings, tuples, lists,
dictionaries, sets and frozensets). All other values are interpreted
as true. (See the "__nonzero__()" special method for a way to change
this.)
The operator "not" yields "True" if its argument is false, "False"
otherwise.
The expression "x and y" first evaluates *x*; if *x* is false, its
value is returned; otherwise, *y* is evaluated and the resulting value
is returned.
The expression "x or y" first evaluates *x*; if *x* is true, its value
is returned; otherwise, *y* is evaluated and the resulting value is
-- More --
-----------------------------标识符----
首:字母、下划线
含:字母 下划线 数字 不能关键字(查看关键字类型的 x = '10.0' print type(x) --程序结果--<type 'str'>)
标准【控制台输入输出: raw_input("prompts") print
for instance
1.
pi = 3.14
radius = float(raw_input ('Radius:')) #The default is a character string , here requires a cast float.
area = pi * radius ** 2
print area
----
2.
input = raw_input()
>>>123
print input * 3
123123123
-----------------------------
input = int (raw_input())
>>>123
print input * 3
369
---------------------
数据量比较大时,使用生成器表达式 并不创建列表而是返回一个生成器 # ()
数据量不太大时,考虑使用列表解析 #{}
[i+1 for i in range(10)]
Out[1]: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
[i+1 for i in range(10) if i%2 == 0]
Out[2]: [1, 3, 5, 7, 9]
(i+1 for i in range(10) if i%2 == 0)
Out[3]: <generator object <genexpr> at 0x09B648C8>
-----------------
from random import randint
x = randint(0,300)
for count in range(0,5):
print 'please input a number between 0~300:'
digit = input()
if digit == x:
print'Bingo!'
elif digit > x:
print'too lare!'
elif digit < x:
print'too small!'
-------------------
哥德巴赫猜想
2-100 prime
----第二周测试---------
3填空(1分)
对于一元二次方程,若有 ,则其解是什么?若有多个解,则按照从小到大的顺序在一行中输出,中间使用空格分隔。解保留2位小数(四舍五入)。
正确答案:-3.58 -0.42
4填空(1分)
假设你每年初往银行账户中1000元钱,银行的年利率为4.7%。
一年后,你的账户余额为:
1000 * ( 1 + 0.047) = 1047 元
第二年初你又存入1000元,则两年后账户余额为:
(1047 + 1000) * ( 1 + 0.047) = 2143.209 元
以此类推,第10年年末,你的账户上有多少余额?
注:结果保留2位小数(四舍五入)。
total = 0
for x in xrange(0,10):
total = (total + 1000) * (1 + 0.047)
print total
正确答案:12986.11
5填空(1分)
Python提供了众多的模块。你能找到一个合适的模块,输出今天的日期吗?格式为“yyyy-mm-dd”。可以查找任何搜索引擎和参考资料,并在下面的空白处写出相应的模块名。
正确答案:datetime 或 time 或 calendar
------------二分法求平方根-------
x = 2
low = 0
high = x
guess = (high+ low) / 2
while abs(guess ** 2 -x) >1e-4:
if guess ** 2 > x:
high = guess
else :
low guess
guess = (low + high)/2
print "the root of x is :", guess
------
x<0?
x<1?
ERROR: execution aborted
----------------------prime----
x = 8
for i in range(2,x):
if x % i == 0:
print'x is not a prime!'
break
else:
print'x is a prime'
---------------enhanced edition prime-
x = 8
for i in range (2, int(math.sqrt(x)+1)):
if x % i == 0:
print'x is not a prime!'
break
else:
print'x is a prime'
假设一个合数c(非素数)可以因式分解成两个大于其开方结果的数a,b
则有如下表达式:c=a*b
a*b>c
与假设c=a*b矛盾
所以假设不成立
即合数的因式分解时,至少有一个结果不大于该数开方结果。
不存在这个结果,就说明是素数。
------python格式等快捷键----
-----前50个素数-------
x = 2
count = 0
while count < 50:
for i in range(2,x):
if x%i == 0:
#print x,' is not a prime'
break
else:
print x,'is a prime!'
count += 1
x += 1
-------回文数---
num = 123
num_p = 0
num_t = num
while num != 0:
num_p = num_p * 10 + num% 10
num = num / 10
if num_t == num_p :
print'yes!'
else:
print'NO!'
------
----------------------先存着------------
Python | 静觅
http://cuiqingcai.com/category/technique/python
零基础写python爬虫之爬虫编写全记录_python_脚本之家
http://www.jb51.net/article/57161.htm
--------------------------------------------
感觉电脑神经兮兮的,明明下的正版,还一遍一个样。。。。
妈卖批,刚才真的试了好多次不行,重启,tm的就好了。。。。。
好吧,这种情况很多软件都会有,,,٩(๑òωó๑)۶
还有版本不同有些东西还真tm的完全不同。。。。。
绝逼有问题,往右一动,再回来曾经被覆盖的就再也不出现了,妈卖批。。。。。
粘贴的正确姿势:
------------------------------
也不知这是进了什么模式还是安装包有问题,真恶心。。。
------------------2运行不出来准备换3或者在虚拟机中运行----------------------
import requests
from bs4 import BeautifulSoup
import bs4
def getHTMLText(url):
try:
r = requests.get(url,timeout = 30)
r.raise_for_status()
r.encoding = r.apparent_encoding
return r.text
except:
return""
#return""
def fillUnivList(ulist,html):
soup = BeautifulSoup(html,"html.parser")
for tr in soup.find('tbody').children:
if isinstance(tr,bs4.element.Tag):
tds = tr('td')
ulist.append([tds[0].string,tds[1].string,tds[2].string])
#pass
def printUnivList(uList,num):
# print("Suc"+str(num))
print("{:^10}\t{:^6}\t{:^10}".format("排名","学校","总分"))
for i in range(num):
u = ulist[i]
print("{:^10}\t{:^6}\t{:^10}".format(u[0],u[1]),u[2])
#print("Suc"+str(num))
def main():
uinfo = []
url = 'http://www.zuihaodaxue.cn/zuihaodaxuepaiming2016.html'
html = getHTMLText(url)
fillUnivList(uinfo,html)
printUnivList(uinfo,20) # 20 univs
main()
------------------------------------------
如何打开requests库get方法的源代码?
-------------------------------
-------------如果有Python3你还用2 那我就呵呵呵了-----------
安装Python3_没毛病,就是不识字。。。。。
http://jingyan.baidu.com/article/a17d5285ed78e88098c8f222.html?st=2&net_type=&bd_page_type=1&os=0&rst=&word=www.10010
安装python2-这个别用了除非你有毛病!!!还要配置环境变量,3貌似不用了,,,,
http://jingyan.baidu.com/article/7908e85c78c743af491ad261.html
3.6.1rc1 Documentation
https://docs.python.org/3.6/index.html
---------------------
慢的要死
-------------------
--------------------------
有坑-----------------------
----呵呵卸载了python马上就好了-----
千万别把两个功能相同的软件同时安装,指不定就出什么毛病,毕竟操作系统等等好多内容都不会
-----------------------------------好特么慢!!!!---------------------
-----------------3.21------------------
conda list 列出自带的库
1.Python3
Q:
pip install scrapy(失败!!!!)
A:
从
下载好的是一个whl文件
然后pip install xxx.whl就行了

把它放D盘,然后pip install D:\Scrapy-1.3.3-py2.py3-none-any.whl
为啥..我用whl还是提示我没有c库..
那就需要安装一下缺少的库了,我刚安装完,然后再次pip install 就不报错了
-------------------------
2.
anaconda
conda install scrapy(成)
--------------结论:版本不同,很多东西都tm不同----------------------要是自己玩,会死的很惨--------
------------Python3的33个关键字----------
-------------3.27.2017------
四种遍历方式:
--------------
--------------------------------
Q: if __name__ == "__main__":
A:
__name__是指示当前py文件调用方式的方法。如果它等于"__main__"就表示是直接执行,如果不是,则用来被别的文件调用,这个时候if就为False,那么它就不会执行最外层的代码了。
比如你有个Python文件里面
def XXXX():
#body
print "asdf"
这样的话,就算是别的地方导入这个文件,要调用这个XXXX函数,也会执行print "asdf",因为他是最外层代码,或者叫做全局代码。但是往往我们希望只有我在执行这个文件的时候才运行一些代码,不是的话(也就是被调用的话)那就不执行这些代码,所以一般改为
def XXXX():
#body
if __name__="__main__":
print "asdf"
这个表示执行的是此代码所在的文件。 如果这个文件是作为模块被其他文件调用,不会执行这里面的代码。 只有执行这个文件时, if 里面的语句才会被执行。 这个功能经常可以用于进行测试。
>>> import re
>>> match = re.match(r'[1-9]\d{5}','100081 BIT')
>>> if match:
... match.group(0)
...
'100081'
>>> print(match.group(1))
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
IndexError: no such group
------------------------
>>> import re
>>> re.split(r'[1-9]\d{5}','BIT100032 BIO100067 BOF100025 BFC100027',maxsplit =2)
['BIT', ' BIO', ' BOF100025 BFC100027']
>>>
------------------------
>>> import re
>>> re.sub(r'[1-9]\d{5}',':zipcode','BIT100032 BIR100065 BSM123035')
'BIT:zipcode BIR:zipcode BSM:zipcode'
。即。
\. 即任意字符
【】里只取一个字符
{n}前一字符重复n次
-------------------------
函数性用法:一次性操作
>>> rst = re.search(r'[1-9]\d{5}','BIT 100081')
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
NameError: name 're' is not defined
>>> import re
>>> rst = re.search(r'[1-9]\d{5}','BIT 100081')
>>>
>>> rst
<_sre.SRE_Match object; span=(4, 10), match='100081'>
>>>
------------------------
面向对象的用法:编译后的多次操作(regex可换成其他英文变量表示)
re型字符串编译成正则表达式类型生成regex对象;然后直接调用regex的search方法
>>> import re
>>> regex = re.compile(r'[1-9]\d{5}')
>>> rst = regex.search('BIT 100049')
>>>
>>> rst
<_sre.SRE_Match object; span=(4, 10), match='100049'>
>>>