jdbc批量执行SQL insert 操作
- package com.file;
- import java.io.BufferedReader;
- import java.io.FileReader;
- import java.util.ArrayList;
- public class ResolvFile {
- public static String readFileContent(String filepath) {
- //1.读取每一行记录,保存到List中
- ArrayList<String> records = new ArrayList<String>();
- try {
- BufferedReader br = new BufferedReader(new FileReader(filepath));
- String aRecord;
- while((aRecord = br.readLine())!=null){
- records.add(aRecord);//把读取到的每一行记录保存到List中
- }
- br.close();//用完以后关闭流
- } catch (Exception e) {
- e.printStackTrace();
- }
- //2.处理每一条记录成SQL语句或保存为对象(a.去掉字段前后的分号b.拼接成SQL或者保存为对象)
- ArrayList<String> recordList = new ArrayList<String>();//用于保存生成的SQL或对象
- for(int i = 0;i<records.size();i++) {
- String record = records.get(i);
- String[] recArray = minusQuotation(record.split(","));
- //拼接SQL语句或保存为对象
- String recordSql = getRecordSql(recArray);
- if (null!=recordSql) {
- recordList.add(recordSql);
- }
- }
- //3.批量执行SQL或保存对象
- batchExecuteSql(recordList);
- return null;
- }
- public static int batchExecuteSql(ArrayList<String> sqlList) {
- System.out.println("接下来可以执行SQL语句或保存对象");
- System.out.println("========批量执行SQL语句==========");
- System.out.println("将所有语句加入到Statment stat中");
- for (int i = 0;i<sqlList.size();i++) {
- String string = sqlList.get(i);
- System.out.println("通过stat.addBatch(sql)来加入语句"+i+": '"+string+"'");
- }
- System.out.println("通过stat.executeBatch()来执行所有的SQL语句");
- System.out.println("========批量执行SQL语句结束==========");
- //int count = stat.executeBatch();
- //return count;//返回执行的语句数量
- return sqlList.size();
- }
- //生成每条记录的SQL
- public static String getRecordSql(String[] recArray) {
- if (null==recArray) {
- return null;
- }
- String recordSql = "insert into tablename (sms,no,time) values('"+recArray[0]+"','"+recArray[2]+"','"+recArray[5]+"')";
- return recordSql;
- }
- /**
- * 去掉数组中每一个元素的开头和结尾的引号
- * @param recArray 要处理的数组
- * @return 处理后的数组
- */
- public static String[] minusQuotation(String[] recArray) {
- for (int i = 0; i < recArray.length; i++) {
- String str = recArray[i];
- if (null!=str) {
- if(str.indexOf( "\"")==0)
- str = str.substring(1,str.length());//去掉开头的分号
- if(str.lastIndexOf("\"")==(str.length()-1))
- str = str.substring(0,str.length()-1); //去掉最后的分号
- }
- recArray[i] = str;
- }
- return recArray;
- }
- public static void main(String[] args) {
- String filepath = "E:\\sxySMS\\smstest.txt";
- readFileContent(filepath);
- }
- }
作者:少帅
出处:少帅的博客--http://www.cnblogs.com/wang3680
您的支持是对博主最大的鼓励,感谢您的认真阅读。
本文版权归作者所有,欢迎转载,但请保留该声明。
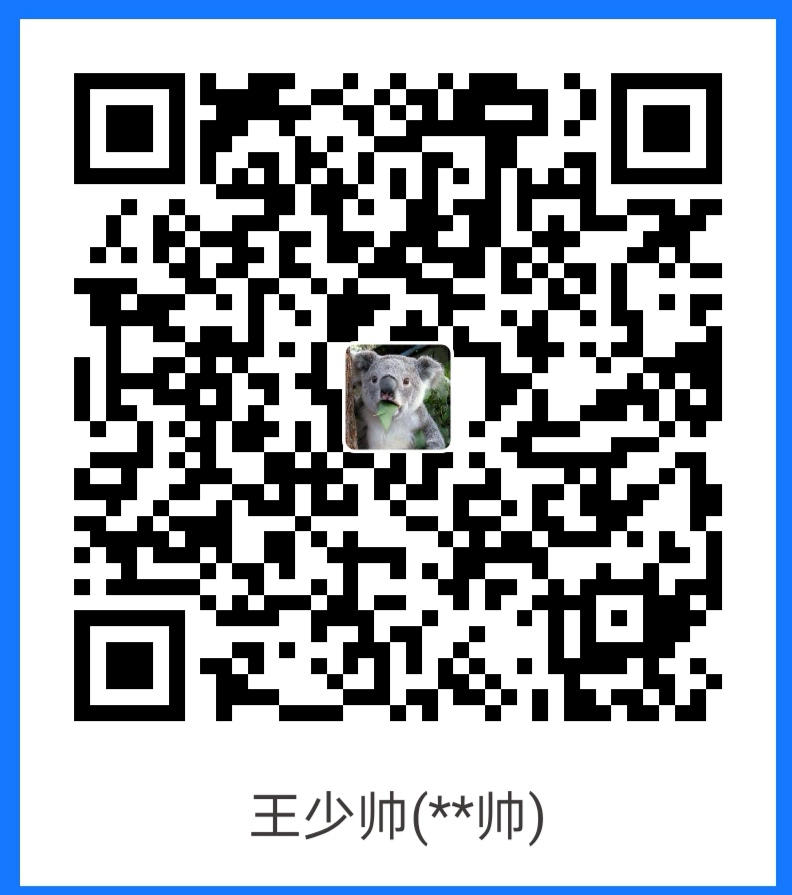
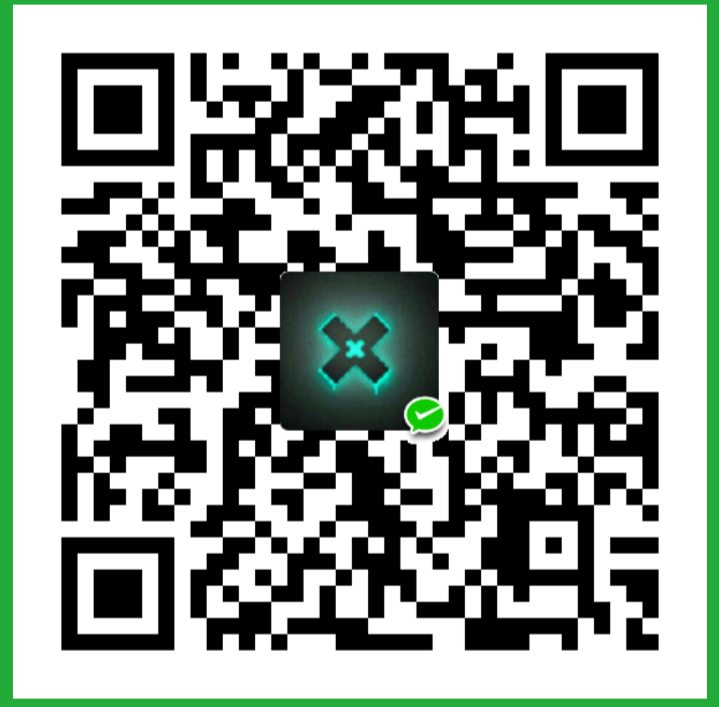