ssh之雇员管理系统(8)-增加其他功能
一、增加雇员
前面我们已经讲得有大部分关键技术啦,而现在也就是我们要扩展,写出来自己的水平啦,当然,逻辑和你的经验将是成功的保障。
- 增加雇员的话,我们先将这个页面打通
<a href="${pageContext.request.contextPath}/employee.do?flag=addEmpUi">添加雇员</a><br/>
这句话中flag是你要调用的那个函数的名称,那我们就开始写这个函数,我们将这些逻辑都写在叫EmployeeAction.java的action类中
public ActionForward addEmpUi(ActionMapping mapping, ActionForm form, HttpServletRequest request, HttpServletResponse response) throws Exception { // TODO Auto-generated method stub return mapping.findForward("addEmpUi"); }
这个就是我们调转页面的函数,相信大家都能一目了然啦吧。
那我们这时候就得配置页面跳转的逻辑啦,看struts-config.xml啦,在<action-mapping>中添加这个页面跳转。
<action path="/employee" name="employeeForm" parameter="flag"> <forward name="addEmpUi" path="/WEB-INF/addEmpUi.jsp" /> </action>
别忘啦在applicationContext.xml中配置这个action,以为spring接管啦struts,那他就接管啦struts的action。
<bean id="departmentService" class="com.wang.service.imp.DepartmentService">
<property name="sessionFactory" ref="sessionFactory"></property>
</bean>
- 那我们就得触及到spring啦,注入。。。那怎样注入呢?
先把添加雇员的表单的信息取到吧,我们还根据原先的表单EmployeeForm.java来配置吧,这样省事啦,

package com.wang.web.form; import org.apache.struts.action.ActionForm; public class EmployeeForm extends ActionForm{ private String id; private String pwd; private String email; private String grade; private String name; private String salary; private String departmentId; public String getEmail() { return email; } public void setEmail(String email) { this.email = email; } public String getGrade() { return grade; } public void setGrade(String grade) { this.grade = grade; } public String getName() { return name; } public void setName(String name) { this.name = name; } public String getSalary() { return salary; } public void setSalary(String salary) { this.salary = salary; } public String getDepartmentId() { return departmentId; } public void setDepartmentId(String departmentId) { this.departmentId = departmentId; } public String getId() { return id; } public void setId(String id) { this.id = id; } public String getPwd() { return pwd; } public void setPwd(String pwd) { this.pwd = pwd; } }
现在我们表单啦,我们还得再action中添加一个叫addEmp的方法,来接收表单的信息啦,但是当我们接收后,添加到Employee类中,那个部门的字段怎么办,他是这样定义的饿,private Department department; 我们从表单中取到的是部门的id,这里是部门的对象,所以我们就得建一个接口为DepartmentInterfaces.java,看到我们这个方法getDepartmentById,根据id得到部门对象,所以当然是返回Department类型,有一个参数用于接收id

package com.wang.service.interfaces; import java.io.Serializable; import com.wang.domain.Department; public interface DepartmentInterfaces { //增加部门的方法 public void addDepartment(Department d); //根据department的id得到部门名称 public Department getDepartmentById(Serializable id); }
现在去实现这个接口DepartmentService.java,

package com.wang.service.imp; import java.io.Serializable; import org.hibernate.SessionFactory; import org.springframework.transaction.annotation.Transactional; import com.wang.domain.Department; import com.wang.service.interfaces.DepartmentInterfaces; @Transactional public class DepartmentService implements DepartmentInterfaces { public SessionFactory sessionFactory; public SessionFactory getSessionFactory() { return sessionFactory; } public void setSessionFactory(SessionFactory sessionFactory) { this.sessionFactory = sessionFactory; } public void addDepartment(Department d) { // TODO Auto-generated method stub sessionFactory.getCurrentSession().save(d); } public Department getDepartmentById(Serializable id) { return (Department) sessionFactory.getCurrentSession().get(Department.class, id); } }
现在是关键所在,action类中这样取得注入的bean呢,EmployeeAction.java

package com.wang.web.action; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import org.apache.struts.action.Action; import org.apache.struts.action.ActionForm; import org.apache.struts.action.ActionForward; import org.apache.struts.action.ActionMapping; import org.apache.struts.actions.DispatchAction; import com.wang.domain.Department; import com.wang.domain.Employee; import com.wang.service.imp.DepartmentService; import com.wang.service.imp.EmployeeService; import com.wang.service.interfaces.DepartmentInterfaces; import com.wang.service.interfaces.EmployeeServiceInter; import com.wang.web.form.EmployeeForm; public class EmployeeAction extends DispatchAction { public DepartmentInterfaces departmentInterfaces; public EmployeeServiceInter employeeServiceInter; public void setEmployeeServiceInter(EmployeeServiceInter employeeServiceInter) { this.employeeServiceInter = employeeServiceInter; } public void setDepartmentInterfaces(DepartmentInterfaces departmentInterfaces) { this.departmentInterfaces = departmentInterfaces; } public ActionForward addEmpUi(ActionMapping mapping, ActionForm form, HttpServletRequest request, HttpServletResponse response) throws Exception { // TODO Auto-generated method stub return mapping.findForward("addEmpUi"); } /** * 用于添加雇员的处理函数 * @param mapping * @param form * @param request * @param response * @return * @throws Exception */ public ActionForward addEmp(ActionMapping mapping, ActionForm form, HttpServletRequest request, HttpServletResponse response) throws Exception { EmployeeForm employeeForm = (EmployeeForm)form; Employee employee = new Employee(); employee.setEmail(employeeForm.getEmail()); employee.setGrade(Integer.parseInt(employeeForm.getGrade())); employee.setName(employeeForm.getName()); employee.setPwd(employeeForm.getPwd()); employee.setSalary(Float.parseFloat(employeeForm.getSalary())); //怎样取得department呢 employee.setDepartment(departmentInterfaces.getDepartmentById(Integer.parseInt(employeeForm.getDepartmentId()))); employeeServiceInter.addEmployee(employee); if(employee!=null){ return mapping.findForward("opereok"); }else { return mapping.findForward("opererr"); } } }
最后在applicationContext.xml中配置注入

<!-- 使用注解的方式配置逻辑对象 --> <bean id="employeeService" class="com.wang.service.imp.EmployeeService" /> <bean id="departmentService" class="com.wang.service.imp.DepartmentService"> <property name="sessionFactory" ref="sessionFactory"></property> </bean> <!-- 配置action --> <bean name="/login" scope="prototype" class="com.wang.web.action.LoginAction"> <property name="employeeServiceInter" ref="employeeService"></property> </bean> <bean name="/employee" scope="prototype" class="com.wang.web.action.EmployeeAction"> <property name="departmentInterfaces" ref="departmentService"></property> <property name="employeeServiceInter" ref="employeeService"></property> </bean>
作者:少帅
出处:少帅的博客--http://www.cnblogs.com/wang3680
您的支持是对博主最大的鼓励,感谢您的认真阅读。
本文版权归作者所有,欢迎转载,但请保留该声明。
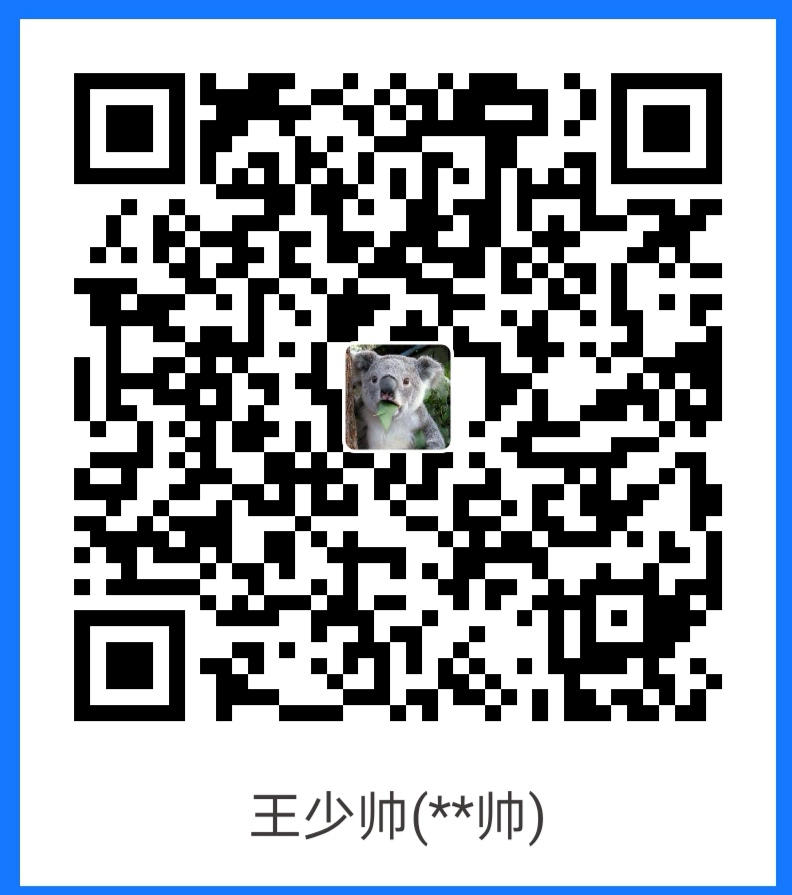
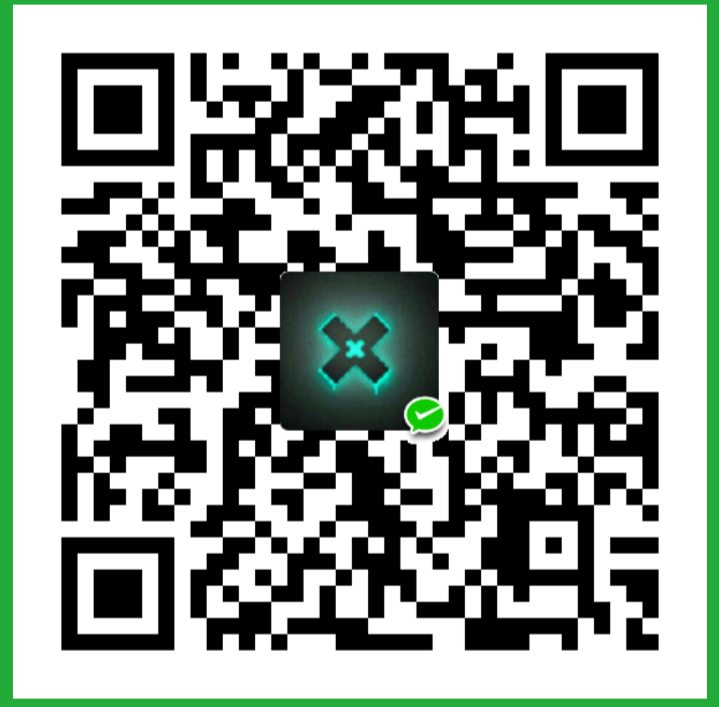