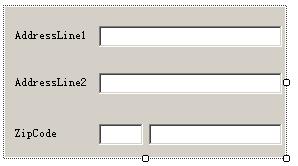
用户控件效果如上图
代码如下:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Drawing;
using System.Data;
using System.Text;
using System.Windows.Forms;

namespace WindowsControlLibrary1


{
public partial class UserControl1 : UserControl

{
public event EventHandler AddressLine1Changed;
public event EventHandler AddressLine2Changed;
public event EventHandler ZoneChanged;
public event EventHandler NumberChanged;

private void TextBoxControls_TextChanged(object sender, EventArgs e)

{
switch (((TextBox)sender).Name)

{
case "txtAddress1":
if (AddressLine1Changed != null)

{
AddressLine1Changed(this, EventArgs.Empty);
}
break;
case "txtAddress2":
if (AddressLine2Changed != null)
AddressLine2Changed(this, EventArgs.Empty);
break;
case "txtZone":
if (ZoneChanged != null)
ZoneChanged(this, EventArgs.Empty);
break;
case "txtNumber":
if (NumberChanged != null)
NumberChanged(this, EventArgs.Empty);
break;
}
}
[Category("NumberData"), DescriptionAttribute("Gets or sets the Number value"), DefaultValue("")]
public string Number

{

get
{ return this.txtNumber .Text; }
set

{
if (txtNumber.Text != value)

{
txtNumber.Text = value;
if (NumberChanged != null)
NumberChanged(this, PropertyChangedEventArgs.Empty);
}
}
}
[Category("AddressData"), DescriptionAttribute("Gets or sets the AddressLine1 value"), DefaultValue("")]
public string AddressLine1

{

get
{ return txtAddress1.Text; }
set

{
if (txtAddress1.Text != value)

{
txtAddress1.Text = value;
if (AddressLine1Changed != null)
AddressLine1Changed(this, PropertyChangedEventArgs.Empty);
}
}
}
[Category("AddressData"), DescriptionAttribute("Gets or sets the AddressLine2 value"), DefaultValue("")]
public string AddressLine2

{

get
{ return txtAddress2.Text; }
set

{
if (txtAddress2.Text != value)

{
txtAddress2.Text = value;
if (AddressLine2Changed != null)
AddressLine2Changed(this, PropertyChangedEventArgs.Empty);
}
}
}
[Category("ZoneData"), DescriptionAttribute("Gets or sets the Zone value"), DefaultValue("")]
public string Zone

{

get
{ return txtZone.Text; }
set

{
if (txtZone.Text != value)

{
txtZone.Text = value;
if (ZoneChanged!= null)
ZoneChanged(this, PropertyChangedEventArgs.Empty);
}
}
}
public UserControl1()

{
InitializeComponent();
}
}
}