【APP自动化基础】Appium自动化
Appium架构
环境搭建
获取app的Activity和Package的五种方式
方式一:通过dumpsys window获取
#获取当前页面的Package和Activity
adb shell dumpsys window w | findstr \/ | findstr name=
#或者:
adb shell dumpsys window | findstr mCurrentFocus
adb shell dumpsys window | grep mCu
#获取模拟器正在运行的APP的Package和Activity
adb shell dumpsys activity | find "mFocusedActivity"
adb shell dumpsys window windows | findstr mFocusedApp
#获取真机正在运行的APP的Package和Activity
adb shell dumpsys activity | find "mResumedActivity"
#获取带端口号的Activity
adb shell dumpsys activity top | findstr ACTIVITY
方式二:列出模拟器或真机中所有软件的Package
#获取设备的所有apk对应的包名和路径
adb shell pm list package -f
#获取第三方apk的包
adb shell pm list package -3 -f
方式三:logcat日志抓START
adb shell logcat | grep START
方式四:logcat日志抓ActivityManager(实时查看)
adb shell logcat | grep ActivityManager
adb logcat | grep ActivityManager
adb logcat | grep -i ActivityManager.*Displayed
方式五:通过aapt工具获取
aapt dump badging C:\apk\weixin01.apk
元素定位获取工具
- uiautomatorviewer
- Appium Inspector
- weditor
ADB启动app
adb shell am start com.tencent.tmgp.WePop/.PermissionActivity
adb shell am start -W -n com.tencent.tmgp.WePop/com.tencent.tmgp.WePop.PermissionActivity -S
-W:等待启动完成。
-n component 指定带有软件包名称前缀的组件名称以创建显式 intent,如 com.example.app/.ExampleActivity。
-S:在启动 Activity 前,强行停止目标应用。
具体am参数解释参考:https://blog.csdn.net/weixin_44380181/article/details/129698064
UI自动化项目Demo
import time
from appium import webdriver
from selenium.webdriver.support.wait import WebDriverWait
from selenium.webdriver.common.by import By
from appium.webdriver.common.touch_action import TouchAction
class Base:
"""基类公共方法封装"""
def __init__(self, driver):
"""初始化"""
self.driver = driver
def base_find(self, loc, timeout=5, poll_frequency=0.5):
"""获取查找元素"""
# 显示等待
return WebDriverWait(self.driver,
timeout=timeout,
poll_frequency=poll_frequency) \
.until(lambda x: x.find_element(*loc))
def base_input(self, loc, value):
"""输入操作"""
# 获取元素
el = self.base_find(loc)
# 清空(防止缓存数据)
el.clear()
# 输入
el.send_keys(value)
def base_click(self, loc):
"""点击操作"""
self.base_find(loc).click()
def base_get(self, loc):
"""获取文本"""
return self.base_find(loc).text
def base_xy_click(self, x, y):
"""根据xy轴位置进行点击"""
return TouchAction(self.driver).press(x=x, y=y).release().perform()
def base_switch_handle(self):
"""窗口句柄切换"""
handle = self.driver.current_window_handle # 当前窗体句柄
print("当前窗体句柄:", handle)
handles = self.driver.window_handles # 所有窗体句柄
print("窗体句柄", handles) # 获取浏览器所有窗体的句柄
self.driver.switch_to.window(handles[-1]) # 切换至最新窗口句柄
class PaoPao:
# 1.初始化脚本
def __init__(self):
desired_caps = {
'platformName': 'Android', # 平台系统
'deviceName': 'emulator-5554', # 设备名称
'platformVersion': '7.1.2', # 系统版本
'appPackage': 'com.xueqiu.android', # 被测应用包
'appActivity': '.view.WelcomeActivityAlias', # 要打开的应用活动
# 'udid': '750BBKL22GDN' # 苹果配置
# 'app': 'xueqiu.apk', # 安装应用包
# 'autoLaunch': False, #是否让Appium自动安装和启动应用,默认为True
# 'automationName': "uiautomator1", # 自动化测试框架 (1.4以上的appium不用写)
'autoGrantPermissions': "true", # 默认允许app获取相关权限
'noReset': 'True', # 每次非首次启动(冷启动)可以记住登录
'unicodeKeyboard': 'true', # 此两行是为了解决字符输入不正确的问题
'resetKeyboard': 'true', # 运行完成后重置软键盘的状态
}
self.driver = webdriver.Remote("http://localhost:4723/wd/hub", desired_caps)
self.base = Base(self.driver)
# 2.执行脚本编写
def run(self):
# 判断登录状态
self.base.base_click((By.ID, "dialog_confirm_btn"))
print("tag")
time.sleep(2)
self.base.base_click((By.TAG_NAME, "同意"))
if __name__ == '__main__':
PaoPao().run()
POM设计模式
POM模式介绍
POM(page object model)页面对象模型,将每个待测页面都封装成一个page类。然后将那些繁琐的元素定位和元素操作都封装到这个类里,是一种封装思想。在自动化测试中引入POM设计模式,可以实现页面元素和测试用例的分离,能使测试代码的可读性、维护性和复用性变得更好。
POM设计思路
POM设计模式一般分为三层(分层设计模式)
- 第一层:对selenium进行二次封装,定义一个所有页面类都继承的BasePage类,封装selenium的常用方法,如元素的定位、输入、点击等,用那些封装那些。
- 第二层:将每个待测页面封装成一个page类,这个类中包含三个小层:
- 表现层,页面的可见元素。
- 操作层,对页面元素进行的操作,如输入、点击等。
- 业务层,对页面元素操作后实现的功能,如登录、注册、支付等。
- 第三层:使用单元测试框架对业务逻辑进行测试,并实现数据驱动(参数化)。
项目实战
============================= 提升自己 ==========================
进群交流、获取更多干货, 请关注微信公众号:
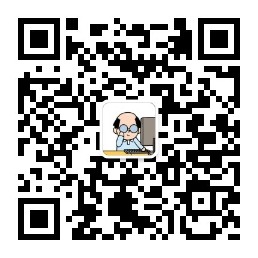
> > > 咨询交流、进群,请加微信,备注来意:sanshu1318 (←点击获取二维码)
> > > 学习路线+测试实用干货精选汇总:
https://www.cnblogs.com/upstudy/p/15859768.html
> > > 【自动化测试实战】python+requests+Pytest+Excel+Allure,测试都在学的热门技术:
https://www.cnblogs.com/upstudy/p/15921045.html
> > > 【热门测试技术,建议收藏备用】项目实战、简历、笔试题、面试题、职业规划:
https://www.cnblogs.com/upstudy/p/15901367.html
> > > 声明:如有侵权,请联系删除。
============================= 升职加薪 ==========================
更多干货,正在挤时间不断更新中,敬请关注+期待。
进群交流、获取更多干货, 请关注微信公众号:
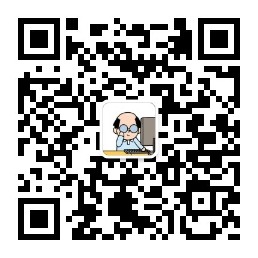
> > > 咨询交流、进群,请加微信,备注来意:sanshu1318 (←点击获取二维码)
> > > 学习路线+测试实用干货精选汇总:
https://www.cnblogs.com/upstudy/p/15859768.html
> > > 【自动化测试实战】python+requests+Pytest+Excel+Allure,测试都在学的热门技术:
https://www.cnblogs.com/upstudy/p/15921045.html
> > > 【热门测试技术,建议收藏备用】项目实战、简历、笔试题、面试题、职业规划:
https://www.cnblogs.com/upstudy/p/15901367.html
> > > 声明:如有侵权,请联系删除。
============================= 升职加薪 ==========================
更多干货,正在挤时间不断更新中,敬请关注+期待。