Java第十六次作业
Cola公司的雇员分为以下若干类:(知识点:多态)
(1) ColaEmployee :这是所有员工总的父类。
属性:员工的姓名,员工的生日月份。
方法:getSalary(int month) 根据参数月份来确定工资,如果该月员工过生日,则公司会额外奖励100 元。
属性:员工的姓名,员工的生日月份。
方法:getSalary(int month) 根据参数月份来确定工资,如果该月员工过生日,则公司会额外奖励100 元。
1 package date519; 2 3 public class ColaEmployee { 4 String name; 5 int month; 6 7 public ColaEmployee() { 8 } 9 10 public ColaEmployee(String name, int month) { 11 this.name = name; 12 this.month = month; 13 } 14 15 public double getSalary(int month) { 16 return 0; 17 } 18 }
(2) SalariedEmployee :ColaEmployee 的子类,拿固定工资的员工。
属性:月薪。
1 package date519; 2 3 public class SalariedEmployee extends ColaEmployee { 4 double monthSalary; 5 6 public SalariedEmployee(String name, int month, double monthSalary) { 7 super(name, month); 8 this.monthSalary = monthSalary; 9 } 10 11 public double getSalary(int month) { 12 if (super.month == month) { 13 return monthSalary + 100; 14 } else { 15 return monthSalary; 16 } 17 } 18 }
(3) HourlyEmployee :ColaEmployee 的子类,按小时拿工资的员工,
每月工作超出160 小时的部分按照1.5 倍工资发放。
属性:每小时的工资、每月工作的小时数。
属性:每小时的工资、每月工作的小时数。
1 package date519; 2 3 public class HourlyEmployee extends ColaEmployee { 4 double hoursalary; 5 int hour; 6 7 public HourlyEmployee(String name, int month, double hoursalary, int hour) { 8 super(name, month); 9 this.hoursalary = hoursalary; 10 this.hour = hour; 11 } 12 13 public double getSalary(int month) { 14 if (super.month == month) { 15 if (hour <= 160) { 16 return month * hoursalary + 100; 17 } else { 18 return month * hoursalary + (hour - 160) * hoursalary * 1.5 + 100; 19 } 20 } else { 21 if (hour <= 160) { 22 return month * hoursalary; 23 } else { 24 return month * hoursalary + (hour - 160) * hoursalary * 1.5; 25 } 26 } 27 } 28 }
(4) SalesEmployee :ColaEmployee 的子类,销售人员,工资由月销售额和提成率决定。
属性:月销售额、提成率。
1 package date519; 2 3 public class SalesEmployee extends ColaEmployee { 4 private int monthSales; 5 private double royaltyRate; 6 7 public SalesEmployee(String name, int month, int monthSales, double royaltyRate) { 8 super(name, month); 9 this.monthSales = monthSales; 10 this.royaltyRate = royaltyRate; 11 } 12 13 public double getSalary(int month) { 14 if (super.month == month) { 15 return monthSales * royaltyRate + 100; 16 } else { 17 return monthSales * royaltyRate; 18 } 19 } 20 }
(5) 定义一个类Company,在该类中写一个方法,调用该方法可以打印出某月某个员工的工资数额,
写一个测试类TestCompany,在main方法,把若干各种类型的员工放在一个ColaEmployee 数组里,
并单元出数组中每个员工当月的工资。
1 package date519; 2 3 public class Company { 4 public void getsalary(ColaEmployee c, int month) { 5 System.out.println(c.name + "在" + c.month + "的月薪为" + c.getSalary(month) + "元"); 6 } 7 8 public static void main(String[] args) { 9 ColaEmployee[] c = { new SalariedEmployee("月薪员工", 6, 3500), new HourlyEmployee("时薪员工", 8, 16, 360), 10 new SalesEmployee("销售员工", 9, 188, 30), }; 11 for (int i = 0; i < c.length; i++) { 12 new Company().getsalary(c[i], 5); 13 } 14 } 15 }
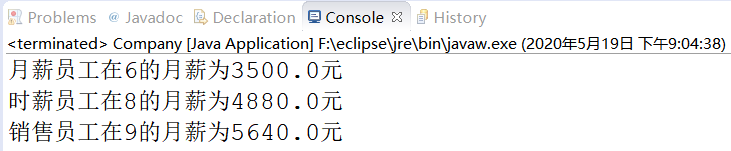