Java第十三次作业
1、定义一个点类Point,包含2个成员变量x、y分别表示x和y坐标,2个构造器Point()和Point(intx0,y0),以及一个movePoint(int dx,int dy)方法,
实现点的位置移动,创建两个Point对象p1、p2,分别调用movePoint方法后,打印p1和p2的坐标。
1 package date430; 2 3 public class Point { 4 5 int x; 6 int y; 7 8 public Point() { 9 10 } 11 12 public Point(int x0, int y0) { 13 this.x = x0; 14 this.y = y0; 15 } 16 17 public void movePoint(int dx, int dy) { 18 this.x += dx; 19 this.y += dy; 20 } 21 22 public static void main(String[] args) { 23 Point p1 = new Point(6, 6); 24 p1.movePoint(8, 8); 25 System.out.println("p1的X坐标为:" + p1.x + ",p1的Y坐标为:" + p1.y); 26 Point p2 = new Point(); 27 p2.movePoint(6, 7); 28 System.out.println("p2的X坐标为:" + p2.x + ",p2的Y坐标为:" + p2.y); 29 } 30 }

2、定义一个矩形类Rectangle:
·定义三个方法:getArea()求面积、getPer()求周长,showAll()分别在控制台输出长、宽、面积、周长。
·有2个属性:长length、宽width。
·通过构造方法Rectangle(int width, int length),分别给两个属性赋值。
·创建一个Rectangle对象,并输出相关信息。
1 package date430; 2 3 public class Rectangle { 4 int length; 5 int width; 6 int Area; 7 int Per; 8 9 public int getArea() { 10 int Area; 11 Area = length * width; 12 return Area; 13 } 14 15 public int getPer() { 16 int Per; 17 Per = (length + width) * 2; 18 return Per; 19 } 20 21 public Rectangle(int length, int width) { 22 this.length = length; 23 this.width = width; 24 this.Area = Area; 25 this.Per = Per; 26 } 27 28 public void showAll() { 29 System.out.println("该矩形的长为:" + length + "\n" + "该矩形的宽为:" + width + "\n" + "该矩形的面积为:" + getArea() + "\n" 30 + "该矩形的周长为:" + getPer()); 31 } 32 33 public static void main(String[] args) { 34 Rectangle i = new Rectangle(6, 8); 35 i.showAll(); 36 } 37 38 }
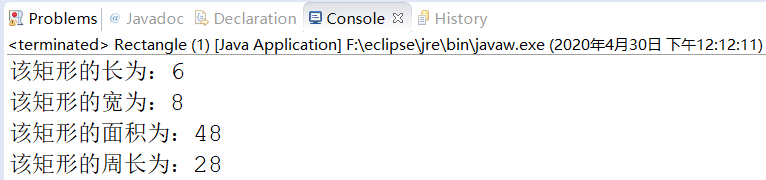
3.定义一个笔记本类,该类有颜色(char)和cpu型号(int)两个属性。
·无参和有参的两个构造方法;有参构造方法可以在创建对象的同时为每个属性赋值;
·输出笔记本信息的方法。
·然后编写一个测试类,测试笔记本类的各个方法。
1 package date430; 2 3 public class notebook { 4 5 char color; 6 int cpu; 7 8 public void getDate() { 9 } 10 11 public void getDate(char color, int cpu) { 12 this.color = color; 13 this.cpu = cpu; 14 } 15 16 void showAll() { 17 System.out.println("该笔记本颜色为:" + color + "\n" + "该笔记本cpu型号为:" + cpu); 18 } 19 20 public static void main(String[] args) { 21 notebook u = new notebook(); 22 u.getDate('灰', 5580); 23 u.showAll(); 24 } 25 }

4.定义两个类,描述如下:
·定义一个人类Person:
定义一个方法sayHello(),可以向对方发出问候语“hello,my name is XXX”。
有三个属性:名字、身高、年龄。
通过构造方法,分别给三个属性赋值。
·定义一个Constructor类:
创建两个对象,分别是zhangsan,33岁,1.73;lishi,44,1.74。
分别调用对象的sayHello()方法。
1 package date430; 2 3 public class Person { 4 String name; 5 int age; 6 double high; 7 8 public void sayHello() { 9 System.out.println("hello,my name is " + name + "."); 10 } 11 12 }
1 package date430; 2 3 public class Person0Constructor { 4 5 public static void main(String[] args) { 6 Person zhangsan = new Person(); 7 Person lisi = new Person(); 8 zhangsan.name = "zhangsan"; 9 zhangsan.age = 33; 10 zhangsan.high = 1.73; 11 lisi.name = "lisi"; 12 lisi.age = 44; 13 lisi.high = 1.74; 14 zhangsan.sayHello(); 15 lisi.sayHello(); 16 } 17 }
