POJ 2488 A Knight's Journey(dfs)
题目代号:POJ 2488
题目链接:http://poj.org/problem?id=2488
Language:
A Knight's Journey
Time Limit: 1000MS | Memory Limit: 65536K | |
Total Submissions: 46017 | Accepted: 15657 |
Description
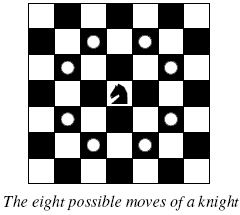
The knight is getting bored of seeing the same black and white squares again and again and has decided to make a journey
around the world. Whenever a knight moves, it is two squares in one direction and one square perpendicular to this. The world of a knight is the chessboard he is living on. Our knight lives on a chessboard that has a smaller area than a regular 8 * 8 board, but it is still rectangular. Can you help this adventurous knight to make travel plans?
Problem
Find a path such that the knight visits every square once. The knight can start and end on any square of the board.
Input
The input begins with a positive integer n in the first line. The following lines contain n test cases. Each test case consists of a single line with two positive integers p and q, such that 1 <= p * q <= 26. This represents a p * q chessboard, where p describes how many different square numbers 1, . . . , p exist, q describes how many different square letters exist. These are the first q letters of the Latin alphabet: A, . . .
Output
The output for every scenario begins with a line containing "Scenario #i:", where i is the number of the scenario starting at 1. Then print a single line containing the lexicographically first path that visits all squares of the chessboard with knight moves followed by an empty line. The path should be given on a single line by concatenating the names of the visited squares. Each square name consists of a capital letter followed by a number.
If no such path exist, you should output impossible on a single line.
If no such path exist, you should output impossible on a single line.
Sample Input
3 1 1 2 3 4 3
Sample Output
Scenario #1: A1 Scenario #2: impossible Scenario #3: A1B3C1A2B4C2A3B1C3A4B2C4
Source
TUD Programming Contest 2005, Darmstadt, Germany
题目大意:国际象棋中的马只能走的路径如图所示,第一列的位置是A,第一行的位置是1,所以1,1的点位置是A1,以此类推,现在让你求能否有一种方式能走完棋盘上所有的点,如果有则输出路径中按字典序排最小的那一种路径。
题目思路:假设一个位置有8种方向可以选择,那么把8个方向对应的点的坐标按字典序排就好了,顺序一定不能错,然后dfs遍历一遍就行了,这条路径一定是字典序最小的那个。
AC代码:
# include <stdio.h> # include <string.h> # include <stdlib.h> # include <iostream> # include <fstream> # include <vector> # include <queue> # include <stack> # include <map> # include <math.h> # include <algorithm> using namespace std; # define pi acos(-1.0) # define mem(a,b) memset(a,b,sizeof(a)) # define FOR(i,a,n) for(int i=a; i<=n; ++i) # define For(i,n,a) for(int i=n; i>=a; --i) # define FO(i,a,n) for(int i=a; i<n; ++i) # define Fo(i,n,a) for(int i=n; i>a ;--i) typedef long long LL; typedef unsigned long long ULL; int a[30][30],n,m,flag; int cx[]={-2,-2,-1,-1,1,1,2,2}; int cy[]={-1,1,-2,2,-2,2,-1,1}; map<int,string>M; void dfs(int i,int j,int step) { if(flag)return; //if(i<1||i>m||j<1||j>n||a[i][j])return; a[i][j]=1; M[step]=""; M[step]+='A'-1+i,M[step]+=j+'0'; if(step==n*m) { flag=1; return; } for(int k=0;k<8;k++) { int x=i+cx[k]; int y=j+cy[k]; if(x>0&&x<=n&&y>0&&y<=m&&!a[x][y]&&!flag) { dfs(x,y,step+1); a[x][y]=0; } } } int main() { int t,i=0; scanf("%d",&t); while(t--) { scanf("%d%d",&m,&n); if(i)puts(""); printf("Scenario #%d:\n",++i); flag=0; mem(a,0); M.clear(); dfs(1,1,1); if(flag) { for(int i=1;i<=n*m;i++) cout<<M[i]; cout<<endl; } else { printf("impossible\n"); } } return 0; }