什么是Postman
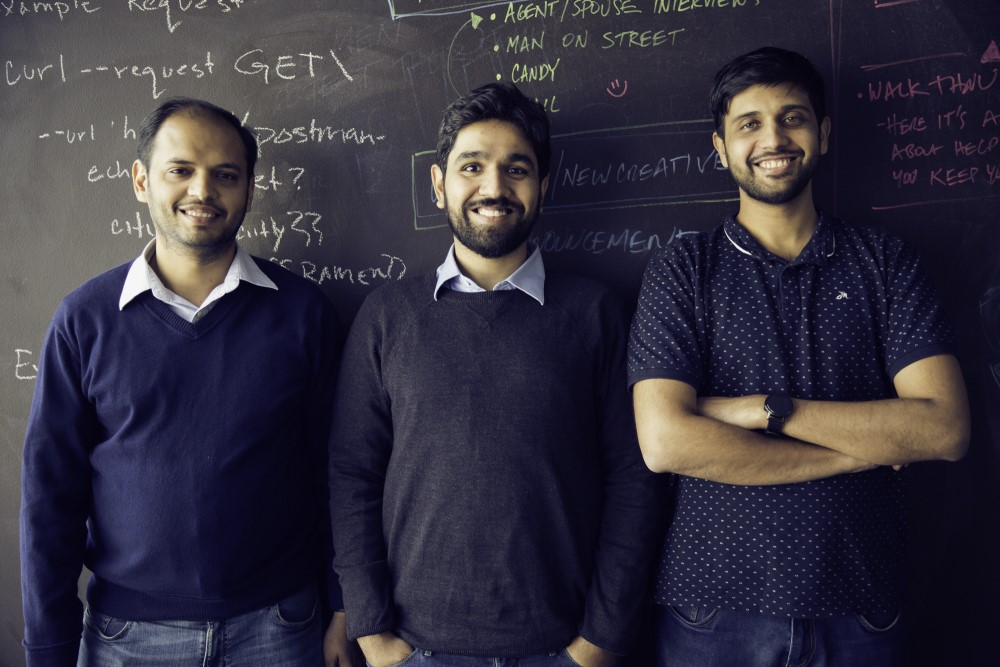
环境变量(Environments)
全局协议
描述 |
变量 |
初始值 |
当前值 |
请求协议 |
request_protocol |
http |
http |
授权信息
描述 |
变量 |
初始值 |
当前值 |
授权-子系统Token |
auth_token_subsystem |
|
|
授权-用户Token |
auth_token_user |
|
|
授权-管理员Token |
auth_token_user_admin |
|
|
授权-客户端Token |
auth_token_client |
|
|
网关信息
描述 |
变量 |
初始值 |
当前值 |
网关域名 |
gateway_hostname |
gateway.xxxxx.com |
gateway.xxxxx.com |
请求地址 |
request_hostname |
gateway.xxxxx.com |
gateway.xxxxx.com |
对外授权
描述 |
变量 |
初始值 |
当前值 |
授权-应用Id |
auth_app_id |
|
|
授权-应用密钥 |
auth_app_secret |
|
|
授权-签名方式 |
auth_sign_type |
|
|
内部授权
描述 |
变量 |
初始值 |
当前值 |
子系统授权-服务密钥 |
auth_sub_system_secret |
|
|
子系统授权-服务标识 |
auth_sub_system_service_id |
|
|
终端请求标识 |
auth_request_flag |
|
|
请求路径
描述 |
变量 |
初始值 |
当前值 |
子系统授权-请求路径 |
auth_sub_system_token_path |
|
|
对外签名-请求路径 |
auth_app_create_sign_path |
|
|
Web后台-登录请求路径 |
auth_token_user_path |
|
|
账号信息
描述 |
变量 |
初始值 |
当前值 |
Web后台-登录用户名 |
web_portal_user_name |
|
|
Web后台-登录用户密码 |
web_portal_user_pwd |
|
|
Web后台-登录终端域名 |
web_portal_hostname |
|
|
Web后台-用户密码摘要 |
web_portal_user_pwd_hash |
|
|
Web后台-管理用户名 |
web_portal_admin_name |
|
|
Web后台-管理用户密码 |
web_portal_admin_pwd |
|
|
Web后台-管理终端域名 |
web_portal_admin_hostname |
|
|
Web后台-用户密码摘要 |
web_portal_admin_pwd_hash |
|
|
请求前置脚本(Pre-request Script)
Base64加解密字符串
CryptoJS
// 待加密字符串
var byEncryptStr = "";
// 加密Base64
var base64Encrypt = CryptoJS.enc.Base64.stringify(CryptoJS.enc.Utf8.parse(byEncryptStr));
console.log('base64Encrypt:', base64Encrypt);
// 解密Base64
var base64Decrypt = CryptoJS.enc.Base64.parse(base64Encrypt).toString(CryptoJS.enc.Utf8);
console.log('base64Decrypt:', base64Decrypt);
MD5摘要字符串
CryptoJS
// 待加密字符串
var byEncryptStr = "";
var hash = CryptoJS.MD5(byEncryptStr).toString();
移除请求内容体的Json注释
// 移除Json注释
const rawData = pm.request.body.toString();
const strippedData = rawData.replace(/\\"|"(?:\\"|[^"])*"|(\/\/.*|\/\*[\s\S]*?\*\/)/g, (m, g) => g ? "" : m)
pm.request.body.update(strippedData);
// 获取当前环境请求体
var requestJson = JSON.parse(pm.request.body.raw);
获取并赋值子系统Token
// 获取当前环境请求协议
var request_protocol = pm.environment.get("request_protocol");
console.log("获取当前环境请求协议:" + request_protocol);
// 获取当前环境网关域名
var gateway_hostname = pm.environment.get("gateway_hostname");
console.log("获取当前环境网关域名:" + gateway_hostname);
// 获取当前环境用户服务
var gateway_service_user = pm.environment.get("gateway_service_user");
console.log("获取当前环境用户服务:" + gateway_service_user);
// 获取当前环境子系统授权路径
var auth_sub_system_token_path = pm.environment.get("auth_sub_system_token_path");
console.log("获取当前环境子系统授权路径:" + auth_sub_system_token_path);
// 拼接并获取子系统授权的请求地址
var getSubSystemTokenUrl = request_protocol + '://' + gateway_hostname + "/" + gateway_service_user + '/' + auth_sub_system_token_path;
console.log("拼接并获取子系统授权的请求地址:" + getSubSystemTokenUrl);
// 获取当前环境子系统密钥
var auth_sub_system_secret = pm.environment.get("auth_sub_system_secret");
console.log("获取当前环境子系统密钥:" + auth_sub_system_secret);
// 获取当前环境子系统身份标识
var auth_sub_system_service_id = pm.environment.get("auth_sub_system_service_id");
console.log("获取当前环境子系统身份标识:" + auth_sub_system_service_id);
// 定义Post请求方法及参数
const echoPostRequest = {
url: getSubSystemTokenUrl,
method: 'POST',
header: [
"Content-Type: application/json",
"Accept: application/json"
],
body: {
mode: 'raw',
raw: JSON.stringify({
"secret": auth_sub_system_secret,
"sid": auth_sub_system_service_id
})
}
};
// 发送自定义请求
pm.sendRequest(echoPostRequest, function (err, response) {
// 将返回结果中的Data赋值到环境变量
pm.environment.set("auth_token_subsystem", response.json().data);
});
获取并赋值对外接口签名
// 获取当前环境请求协议
var request_protocol = pm.environment.get("request_protocol");
console.log("获取当前环境请求协议:" + request_protocol);
// 获取当前环境网关地址
var gateway_path = pm.environment.get("gateway_path");
console.log("获取当前环境网关地址:" + gateway_path);
// 获取当前环境对外服务
var gateway_service_public = pm.environment.get("gateway_service_public");
console.log("获取当前环境对外服务:" + gateway_service_public);
// 获取当前环境生成签名的路径
var auth_app_create_sign_path = pm.environment.get("auth_app_create_sign_path");
console.log("获取当前环境生成签名的路径:" + auth_app_create_sign_path);
// 拼接并获取对外授权签名的请求地址
var createSignUrl = "";
// 判断纯数字的正则表达式
var reg = /^[0-9]+.?[0-9]*$/;
// 如果网关服务名称是纯数字,即服务端口号,说明不走网关模式,即调式模式
if(reg.test(gateway_service_public))
{
createSignUrl = request_protocol + '://' + gateway_path + ":" + gateway_service_public + '/' + auth_app_create_sign_path;
}
else
{
createSignUrl = request_protocol + '://' + gateway_path + "/" + gateway_service_public + '/' + auth_app_create_sign_path;
}
console.log("拼接并获取对外授权签名的请求地址:" + createSignUrl);
// 获取当前环境应用密钥
var auth_app_secret = pm.environment.get("auth_app_secret");
console.log("获取当前环境应用密钥:" + auth_app_secret);
// 定义Post请求方法及参数
const echoPostRequest = {
url: createSignUrl,
method: 'POST',
header: [
"Content-Type: multipart/form-data",
"Accept: application/json"
],
body: {
mode: 'formdata',
formdata: [
{
key: "SignKey", value: auth_app_secret, disabled: false, description: { content:"", type:"text/plain" }
},
{
key: "Content", value: pm.request.body.raw, disabled: false, description: { content:"", type:"text/plain" }
}
]
}
};
// 发送自定义请求
pm.sendRequest(echoPostRequest, function (err, response) {
// 将返回结果中的Sign赋值到环境变量
pm.environment.set("request_sign", response.json().data.sign);
});
登陆并获取Web后台授权Token
// 获取当前环境请求协议
var request_protocol = pm.environment.get("request_protocol");
console.log("获取当前环境请求协议:" + request_protocol);
// 获取当前环境网关域名
var gateway_hostname = pm.environment.get("gateway_hostname");
console.log("获取当前环境网关域名:" + gateway_hostname);
// 获取当前环境用户服务
var gateway_service_user = pm.environment.get("gateway_service_user");
console.log("获取当前环境用户服务:" + gateway_service_user);
// 获取当前环境Web后台登陆路径
var auth_token_user_path = pm.environment.get("auth_token_user_path");
console.log("获取当前环境Web后台登陆路径:" + auth_token_user_path);
// 拼接并获取Web后台登陆的请求地址
var getWebPortalTokenUrl = request_protocol + '://' + gateway_hostname + "/" + gateway_service_user + '/' + auth_token_user_path;
console.log("拼接并获取Web后台登陆的请求地址:" + getWebPortalTokenUrl);
// 获取当前环境Web后台登陆的域名
var web_portal_hostname = pm.environment.get("web_portal_hostname");
console.log("获取当前环境Web后台登陆的域名:" + web_portal_hostname);
// 获取当前环境Web后台登陆的用户名
var web_portal_user_name = pm.environment.get("web_portal_user_name");
console.log("获取当前环境Web后台登陆的用户名:" + web_portal_user_name);
// 获取当前环境Web后台登陆的密码
var web_portal_user_pwd = pm.environment.get("web_portal_user_pwd");
console.log("获取当前环境Web后台登陆的密码:" + web_portal_user_pwd);
// 获取当前环境Web后台登陆的密码摘要
var web_portal_user_pwd_hash = CryptoJS.MD5(web_portal_user_pwd).toString();
console.log("获取当前环境Web后台登陆的密码摘要:" + web_portal_user_pwd_hash);
// 定义Post请求方法及参数
const echoPostRequest = {
url: getWebPortalTokenUrl,
method: 'POST',
header: [
"Content-Type: application/json",
"Accept: application/json"
],
body: {
mode: 'raw',
raw: JSON.stringify({
"username": web_portal_user_name,
"password": web_portal_user_pwd_hash,
"domain": web_portal_hostname
})
}
};
// 发送自定义请求
pm.sendRequest(echoPostRequest, function (err, response) {
// 将返回结果中的Data赋值到环境变量
pm.environment.set("auth_token_user", response.json().data.token);
});
登陆并获取管理后台授权Token
// 获取当前环境请求协议
var request_protocol = pm.environment.get("request_protocol");
console.log("获取当前环境请求协议:" + request_protocol);
// 获取当前环境网关域名
var gateway_hostname = pm.environment.get("gateway_hostname");
console.log("获取当前环境网关域名:" + gateway_hostname);
// 获取当前环境用户服务
var gateway_service_user = pm.environment.get("gateway_service_user");
console.log("获取当前环境用户服务:" + gateway_service_user);
// 获取当前环境Web后台登陆路径
var auth_token_user_path = pm.environment.get("auth_token_user_path");
console.log("获取当前环境Web后台登陆路径:" + auth_token_user_path);
// 拼接并获取Web后台登陆的请求地址
var getWebPortalTokenUrl = request_protocol + '://' + gateway_hostname + "/" + gateway_service_user + '/' + auth_token_user_path;
console.log("拼接并获取Web后台登陆的请求地址:" + getWebPortalTokenUrl);
// 获取当前环境Web后台登陆的域名
var web_portal_admin_hostname = pm.environment.get("web_portal_admin_hostname");
console.log("获取当前环境Web后台登陆的域名:" + web_portal_admin_hostname);
// 获取当前环境Web后台登陆的用户名
var web_portal_admin_name = pm.environment.get("web_portal_admin_name");
console.log("获取当前环境Web后台登陆的用户名:" + web_portal_admin_name);
// 获取当前环境Web后台登陆的密码
var web_portal_admin_pwd = pm.environment.get("web_portal_admin_pwd");
console.log("获取当前环境Web后台登陆的密码:" + web_portal_admin_pwd);
// 获取当前环境Web后台登陆的密码摘要
var web_portal_admin_pwd_hash = CryptoJS.MD5(web_portal_admin_pwd).toString();
console.log("获取当前环境Web后台登陆的密码摘要:" + web_portal_admin_pwd_hash);
// 定义Post请求方法及参数
const echoPostRequest = {
url: getWebPortalTokenUrl,
method: 'POST',
header: [
"Content-Type: application/json",
"Accept: application/json"
],
body: {
mode: 'raw',
raw: JSON.stringify({
"username": web_portal_admin_name,
"password": web_portal_admin_pwd_hash,
"domain": web_portal_admin_hostname
})
}
};
// 发送自定义请求
pm.sendRequest(echoPostRequest, function (err, response) {
// 将返回结果中的Data赋值到环境变量
pm.environment.set("auth_token_user_admin", response.json().data.token);
});
请求后置脚本(Tests)
响应结果可视化(Visualize)
var template = `
<table bgcolor="#FFFFFF">
<tr>
<th>商户ID</th>
<th>商户名称</th>
<th>开始时间</th>
<th>结束时间</th>
<th>耗时(秒)</th>
</tr>
{{#each response}}
<tr>
<td>{{tenantId}}</td>
<td>{{tenantName}}</td>
<td>{{startTime}}</td>
<td>{{endTime}}</td>
<td>{{costTime}}</td>
</tr>
{{/each}}
</table>
`;
// Set visualizer
pm.visualizer.set(template, {
// Pass the response body parsed as JSON as `data`
response: pm.response.json().data.details.dataSource
});
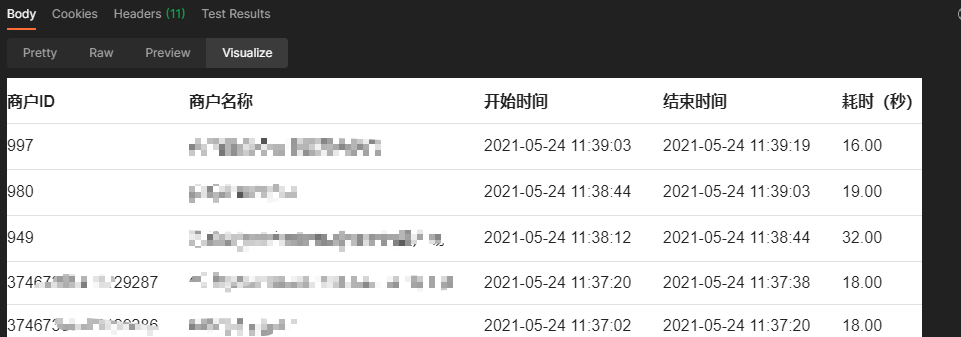
参考