- 类图
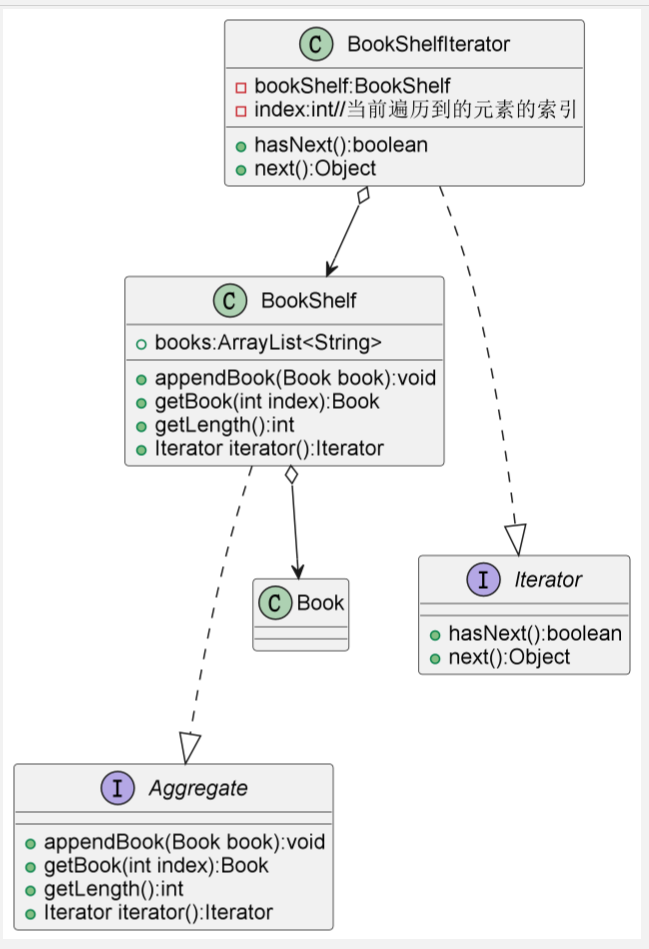
package com.designer.practice4;
import com.designer.practice4.Iterator;
//所要遍历的集合的接口
public interface Aggregate {
//添加图书
public void appendBook(Book book);
//获取图书
public Book getBook(int index);
//获取当前书架的容量
public int getLength();
//遍历方法(返回一个迭代器)
public Iterator iterator();
}
package com.designer.practice4;
import java.util.ArrayList;
//书架
public class BookShelf implements Aggregate{
//当前书架书籍的数量
//书籍
private ArrayList<Book> books;
//创建书架
public BookShelf(){
books=new ArrayList<>();
}
//添加图书
@Override
public void appendBook(Book book){
books.add(book);
}
@Override
//获取图书
public Book getBook(int index){
return (Book) books.get(index);
}
@Override
//获取当前书架的容量
public int getLength(){
return books.size();
}
@Override
public Iterator iterator() {
return new BookShelfIterator(this);
}
}
package com.designer.practice4;
//书架迭代器
public class BookShelfIterator implements Iterator {
private BookShelf bookShelf;
private int index;//当前遍历到的元素的索引
public BookShelfIterator(BookShelf bookShelf){
this.bookShelf=bookShelf;
this.index=0;
}
@Override
public boolean hasNext() {
//判断下一个元素是否存在
if(index < bookShelf.getLength()){
return true;
}
return false;
}
@Override
public Object next() {
//获取当前元素,并将Index指针后移一位
Book book = bookShelf.getBook(index);
index++;
return book;
}
}
package com.designer.practice4;
public interface Iterator {
boolean hasNext();
Object next();
}
package com.designer.practice4;
public class Book {
private String name;
public Book() {
}
public Book(String name) {
this.name = name;
}
/**
* 获取
* @return name
*/
public String getName() {
return name;
}
/**
* 设置
* @param name
*/
public void setName(String name) {
this.name = name;
}
}
package com.designer.practice4;
public class Main {
public static void main(String[] args) {
//创建图书
Book book1 = new Book("Java");
Book book2 = new Book("C++");
Book book3 = new Book("Python");
//创建书架
Aggregate abstractBookShelf = new BookShelf();
//添加图书
abstractBookShelf.appendBook(book1);
abstractBookShelf.appendBook(book2);
abstractBookShelf.appendBook(book3);
//遍历
Iterator iterator = abstractBookShelf.iterator();//将图书注入到了书架遍历器当中
while (iterator.hasNext()) {
Book book = (Book) iterator.next();
System.out.println(book.getName());
}
}
}