Qt实现表格树控件-支持多级表头
原文链接:Qt实现表格树控件-支持多级表头
一、概述
之前写过一篇关于表格控件多级表头的文章,喜欢的话可以参考Qt实现表格控件-支持多级列表头、多级行表头、单元格合并、字体设置等。今天这篇文章带来了比表格更加复杂的控件-树控件多级表头实现。
在Qt中,表格控件包含有水平和垂直表头,但是常规使用模式下都是只能实现一级表头,而树控件虽然包含有了branch分支,这也间接的削弱了他自身的表头功能,细心的同学可能会发现Qt自带的QTreeView树控件只包含有水平表头,没有了垂直表头。
既然Qt自带的控件中没有这个功能,那么我们只能自己去实现了。
要实现多级表头功能方式也多种多样,之前就看到过几篇关于实现多级表头的文章,总体可以分为如下两种方式
- 表头使用一个表格来模拟
- 通过给表头自定义Model
今天这篇文章我们是通过方式2来实现多级表头。如效果图所示,实现的是一个树控件的多级表头,并且他还包含了垂直列头,实现这个控件所需要完成的代码量还是比较多的。本篇文章可以算作是一个开头吧,后续会逐步把关键功能的实现方式分享出来。
二、效果展示
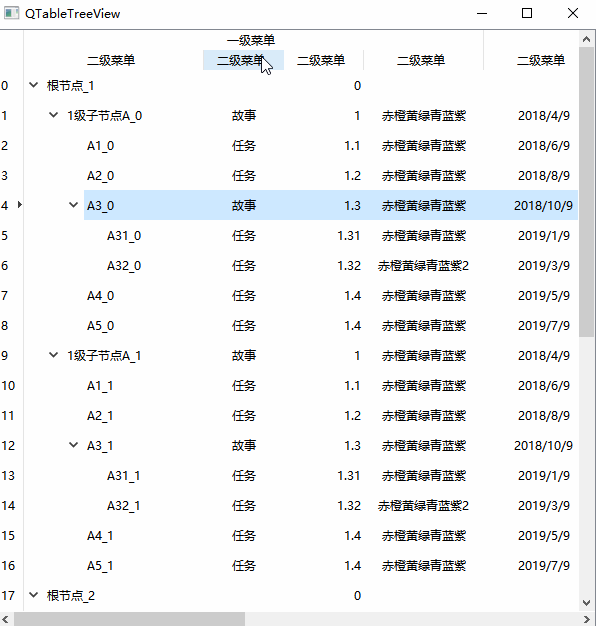
三、实现方式
本篇文章中的控件看起来是一个树控件,但是他又具备了表格控件该有的一些特性,比如垂直表头、多级水平表头等等。要实现这样的树控件,我们有两个大的方向可以去考虑
- 重写表格控件,实现branch
- 表格控件+树控件
方式1重写表格控件实行branch的工作量是比较大的,而且需要把Qt原本的代码迁出来,工作量会比加大,个人选择了发你。
方式2是表格控件+树控件的实现方式,说白了就是表格控件提供水平和垂直表头,树控件提供内容展示,听起来好像没毛病,那么还等什么,直接干呗。
既然大方向定了,那么接下来可能就是一些细节问题的确定。
- 多级水平表头
- 垂直列头拖拽时,实现树控件行高同步变动
- 自绘branch
以上三个问题都是实现表格树控件时遇到的一些比较棘手的问题,后续会分别通过单独的文章来进行讲解,今天这篇文章也是我们的第一讲,怎么实现水平多级表头
四、多级表头
第一节我们也说了,实现多级表头我们使用重写model的方式来实现,接下来就是贴代码的时候。
1、数据源
经常重写model的同学对如下代码应该不陌生,对于继承自QAbstractItemModel的数据源肯定是需要重写该类的所有纯虚方法,包括所有间接父类的纯虚方法。
除此之外自定义model应该还需要提供一个可以合并单元格的方法,为什么呢?因为我们多级表头需要。比如说我们一级表头下有3个二级表头,那这就说明一级表头合并了3列,使得本身的3列数据变成一列。
class HHeaderModel : public QAbstractItemModel
{
struct ModelData //模型数据结构
{
QString text;
ModelData() : text("")
{
}
};
Q_OBJECT
public:
HHeaderModel(QObject * parent = 0);
~HHeaderModel();
public:
void setItem(int row, int col, const QString & text);
QString item(int row, int col);
void setSpan(int firstRow, int firstColumn, int rowSpanCount, int columnSpanCount);
const CellSpan& getSpan(int row, int column);
public:
virtual QModelIndex index(int row, int column, const QModelIndex & parent) const override;
virtual QModelIndex parent(const QModelIndex & child) const override;
virtual int rowCount(const QModelIndex & parent) const override;
virtual int columnCount(const QModelIndex & parent) const override;
virtual QVariant data(const QModelIndex & index, int role) const override;
virtual Qt::ItemFlags flags(const QModelIndex & index) const override;
virtual bool setData(const QModelIndex & index, const QVariant & value, int role = Qt::EditRole) override;
virtual QVariant headerData(int section, Qt::Orientation orientation, int role) const override;
private:
//找到对应的模型数据
ModelData * modelData(const QModelIndex & index) const;
private:
//key rowNo, key colNo
QMap<int, QMap<int, ModelData *> > m_modelDataMap;
int m_iMaxCol;
CellSpan m_InvalidCellSpan;
QList<CellSpan> m_cellSpanList;
};
以上便是model的头文件声明,其中重写父类的虚方法这里就不做过多说明,和平时重写其他数据源没有区别,这里多了重点说明下setSpan接口。
void HHeaderModel::setSpan(int firstRow, int firstColumn, int rowSpanCount, int columnSpanCount)
{
for (int row = firstRow; row < firstRow + rowSpanCount; ++row)
{
for (int col = firstColumn; col < firstColumn + columnSpanCount; ++col)
{
m_cellSpanList.append(CellSpan(row, col, rowSpanCount, columnSpanCount, firstRow, firstColumn));
}
}
}
const CellSpan& HHeaderModel::getSpan(int row, int column)
{
for (QList<CellSpan>::const_iterator iter = m_cellSpanList.begin(); iter != m_cellSpanList.end(); ++iter)
{
if ((*iter).curRow == row && (*iter).curCol == column)
{
return *iter;
}
}
return m_InvalidCellSpan;
}
setSpan接口存储了哪些列或者行被合并了,在绘制表格头时我们也可以根据这些信息来计算绘制的大小和位置。
2、表格
数据源介绍完,接下来看看表头视图类,这个类相对Model来说会复杂很多,主要也是根据Model中存储的合并信息来进行绘制。
由于这个类代码比价多,这里我就不贴头文件声明了,下面还是主要介绍下关键函数
a、paintEvent绘制函数
void HHeaderView::paintEvent(QPaintEvent * event)
{
QPainter painter(viewport());
QMultiMap<int, int> rowSpanList;
int cnt = count();
int curRow, curCol;
HHeaderModel * model = qobject_cast<HHeaderModel *>(this->model());
for (int row = 0; row < model->rowCount(QModelIndex()); ++row)
{
for (int col = 0; col < model->columnCount(QModelIndex()); ++col)
{
curRow = row;
curCol = col;
QStyleOptionViewItemV4 opt = viewOptions();
QStyleOptionHeader header_opt;
initStyleOption(&header_opt);
header_opt.textAlignment = Qt::AlignCenter;
// header_opt.icon = QIcon("./Resources/logo.ico");
QFont fnt;
fnt.setBold(true);
header_opt.fontMetrics = QFontMetrics(fnt);
opt.fontMetrics = QFontMetrics(fnt);
QSize size = style()->sizeFromContents(QStyle::CT_HeaderSection, &header_opt, QSize(), this);
// size.setHeight(25);
header_opt.position = QStyleOptionHeader::Middle;
//判断当前行是否处于鼠标悬停状态
if (m_hoverIndex == model->index(row, col, QModelIndex()))
{
header_opt.state |= QStyle::State_MouseOver;
// header_opt.state |= QStyle::State_Active;
}
opt.text = model->item(row, col);
header_opt.text = model->item(row, col);
CellSpan span = model->getSpan(row, col);
int rowSpan = span.rowSpan;
int columnSpan = span.colSpan;
if (columnSpan > 1 && rowSpan > 1)
{
//单元格跨越多列和多行, 不支持,改为多行1列
continue;
/*header_opt.rect = QRect(sectionViewportPosition(logicalIndex(col)), row * size.height(), sectionSizes(col, columnSpan), rowSpan * size.height());
opt.rect = header_opt.rect;
col += columnSpan - 1; */
}
else if (columnSpan > 1)//单元格跨越多列
{
header_opt.rect = QRect(sectionViewportPosition(logicalIndex(col)), row * size.height(), sectionSizes(col, columnSpan), size.height());
opt.rect = header_opt.rect;
col += columnSpan - 1;
}
else if (rowSpan > 1)//单元格跨越多行
{
header_opt.rect = QRect(sectionViewportPosition(logicalIndex(col)), row * size.height(), sectionSize(logicalIndex(col)), size.height() * rowSpan);
opt.rect = header_opt.rect;
for (int i = row + 1; i <= rowSpan - 1; ++i)
{
rowSpanList.insert(i, col);
}
}
else
{
//正常的单元格
header_opt.rect = QRect(sectionViewportPosition(logicalIndex(col)), row * size.height(), sectionSize(logicalIndex(col)), size.height());
opt.rect = header_opt.rect;
}
opt.state = header_opt.state;
//opt.displayAlignment = Qt::AlignCenter;
//opt.icon = QIcon("./Resources/logo.ico");
//opt.backgroundBrush = QBrush(QColor(255, 0, 0));
QMultiMap<int, int>::iterator it = rowSpanList.find(curRow, curCol);
if (it == rowSpanList.end())
{
//保存当前item的矩形
m_itemRectMap[curRow][curCol] = header_opt.rect;
itemDelegate()->paint(&painter, opt, model->index(curRow, curCol, QModelIndex()));
painter.setPen(QColor("#e5e5e5"));
painter.drawLine(opt.rect.bottomLeft(), opt.rect.bottomRight());
}
else
{
//如果是跨越多行1列的情况,采用默认的paint
}
}
}
//painter.drawLine(viewport()->rect().bottomLeft(), viewport()->rect().bottomRight());
}
b、列宽发生变化
void HHeaderView::onSectionResized(int logicalIndex, int oldSize, int newSize)
{
if (0 == newSize)
{
//过滤掉隐藏列导致的resize
viewport()->update();
return;
}
static bool selfEmitFlag = false;
if (selfEmitFlag)
{
return;
}
int minWidth = 99999;
QFontMetrics metrics(font());
//获取这列上最小的字体宽度,移动的长度不能大于最小的字体宽度
HHeaderModel * model = qobject_cast<HHeaderModel *> (this->model());
for (int i = 0; i < model->rowCount(QModelIndex()); ++i)
{
QString text = model->item(i, logicalIndex);
if (text.isEmpty())
continue;
int textWidth = metrics.width(text);
if (minWidth > textWidth)
{
minWidth = textWidth;
}
}
if (newSize < minWidth)
{
selfEmitFlag = true;
resizeSection(logicalIndex, oldSize);
selfEmitFlag = false;
}
viewport()->update();
}
3、QStyledItemDelegate绘制代理
既然说到了自绘,那么有必要说下Qt自绘相关的一些东西。
a、paintEvent
paintEvent是QWidget提供的自绘函数,当界面刷新时该接口就会被调用。
b、复杂控件自绘
对于一些比较复杂的控件为了达到更好的定制型,Qt把paintEvent函数中的绘制过程进行了更为详细的切割,也可以让我们进行布局的重写。
比如今天说到的树控件,当paintEvent函数调用时,其实内部真正进行绘制的是如下3个函数,重写如下三个函数可以为我们带来更友好的定制性,并且很大程度上减轻了我们自己去实现的风险。
virtual void drawBranches(QPainter *painter, const QRect &rect, const QModelIndex &index) const
virtual void drawRow(QPainter *painter, const QStyleOptionViewItem &option, const QModelIndex &index) const
void drawTree(QPainter *painter, const QRegion ®ion) const
c、QStyledItemDelegate绘制代理
为了更好的代码管理和接口抽象,Qt在处理一些超级变态的控件时提供了绘制代理这个类,即使在一些力度很小的绘制函数中依然是调用的绘制代理去绘图。
刚好我们今天说的这个表头绘制就是如此。如下代码所示,是一部分的HHeaderItemDelegate::paint函数展示,主要是针对表头排序进行了定制。
void HHeaderItemDelegate::paint(QPainter * painter, const QStyleOptionViewItem & option, const QModelIndex & index) const
{
int row = index.row();
int col = index.column();
const int textMargin = QApplication::style()->pixelMetric(QStyle::PM_FocusFrameHMargin) + 1;
QStyleOptionHeader header_opt;
header_opt.rect = option.rect;
header_opt.position = QStyleOptionHeader::Middle;
header_opt.textAlignment = Qt::AlignCenter;
header_opt.state = option.state;
//header_opt.state |= QStyle::State_HasFocus;//QStyle::State_Enabled | QStyle::State_Horizontal | QStyle::State_None | QStyle::State_Raised;
if (HHeaderView::instance->isItemPress(row, col))
{
header_opt.state |= QStyle::State_Sunken; //按钮按下效果
}
// if ((QApplication::mouseButtons() && (Qt::LeftButton || Qt::RightButton)))
// header_opt.state |= QStyle::State_Sunken;
painter->save();
QApplication::style()->drawControl(QStyle::CE_Header, &header_opt, painter);
painter->restore();
}
五、测试代码
把需要合并的列和行进行合并,即可达到多级表头的效果,如下是设置表格model合并接口展示。
horizontalHeaderModel->setSpan(0, 0, 1, 4);
horizontalHeaderModel->setSpan(0, 4, 1, 3);
horizontalHeaderModel->setSpan(0, 7, 1, 3);
horizontalHeaderModel->setSpan(0, 10, 2, 1);
horizontalHeaderModel->setSpan(0, 11, 2, 1); //不支持跨越多行多列的情况
}
model设置完毕后只要把Model设置给QHeaderView类即可。
六、相关文章
值得一看的优秀文章:
![]() |
![]() |
很重要--转载声明
-
本站文章无特别说明,皆为原创,版权所有,转载时请用链接的方式,给出原文出处。同时写上原作者:朝十晚八 or Twowords
-
如要转载,请原文转载,如在转载时修改本文,请事先告知,谢绝在转载时通过修改本文达到有利于转载者的目的。