基本要求
1、拓扑文件代码(/home/k/032002225/lab3/):
from mininet.net import Mininet
from mininet.node import Controller, RemoteController, OVSController
from mininet.node import CPULimitedHost, Host, Node
from mininet.node import OVSKernelSwitch, UserSwitch
from mininet.node import IVSSwitch
from mininet.cli import CLI
from mininet.log import setLogLevel, info
from mininet.link import TCLink, Intf
from subprocess import call
def myNetwork():
net = Mininet( topo=None,
build=False,
ipBase='10.0.0.0/8')
info( '*** Adding controller\n' )
c0=net.addController(name='c0',
controller=Controller,
protocol='tcp',
port=6633)
info( '*** Add switches\n')
s1 = net.addSwitch('s1', cls=OVSKernelSwitch)
s2 = net.addSwitch('s2', cls=OVSKernelSwitch)
info( '*** Add hosts\n')
h1 = net.addHost('h1', cls=Host, ip='192.168.0.101/24', defaultRoute=None)
h2 = net.addHost('h2', cls=Host, ip='192.168.0.102/24', defaultRoute=None)
h3 = net.addHost('h3', cls=Host, ip='192.168.0.103/24', defaultRoute=None)
h4 = net.addHost('h4', cls=Host, ip='192.168.0.104/24', defaultRoute=None)
info( '*** Add links\n')
net.addLink(h1, s1)
net.addLink(h2, s1)
net.addLink(s1, s2)
net.addLink(s2, h3)
net.addLink(s2, h4)
info( '*** Starting network\n')
net.build()
info( '*** Starting controllers\n')
for controller in net.controllers:
controller.start()
info( '*** Starting switches\n')
net.get('s1').start([c0])
net.get('s2').start([c0])
info( '*** Post configure switches and hosts\n')
CLI(net)
net.stop()
if __name__ == '__main__':
setLogLevel( 'info' )
myNetwork()
2、hello
控制器6633端口(我最高能支持OpenFlow 1.0) ---> 交换机49344端口
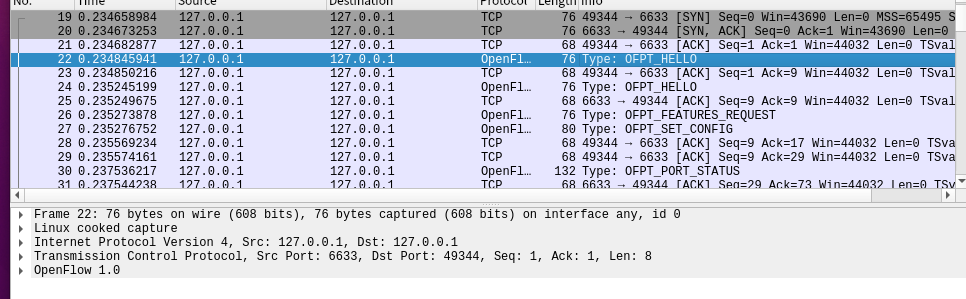
交换机49344端口(我最高能支持OpenFlow 1.5) ---> 控制器6633端口
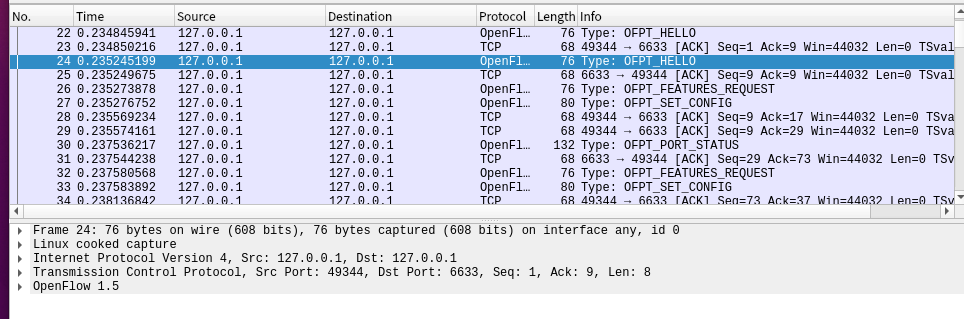
于是双方建立连接,并使用OpenFlow 1.0
3、Features Request
控制器6633端口(我需要你的特征信息) ---> 交换机49344端口
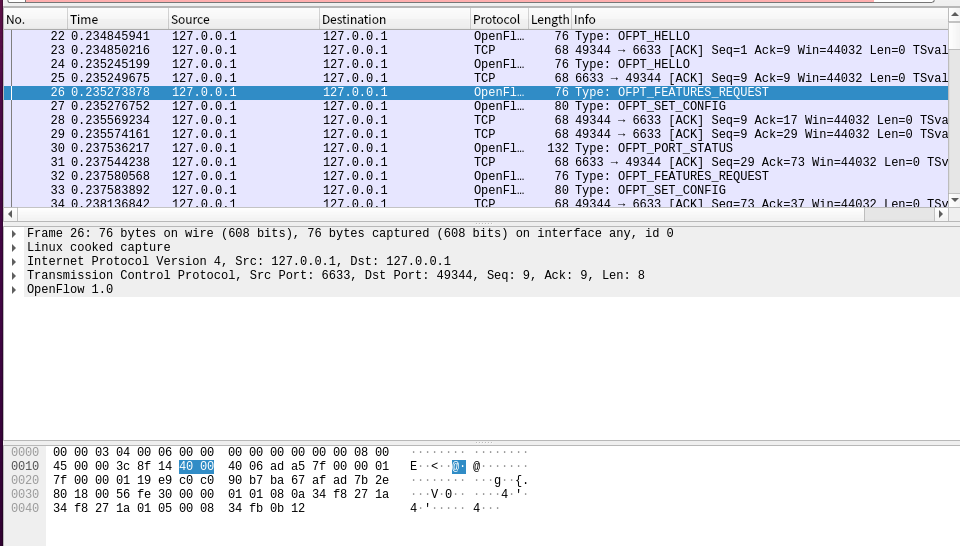
控制器6633端口(请按照我给你的flag和max bytes of packet进行配置) --->交换机49344端口
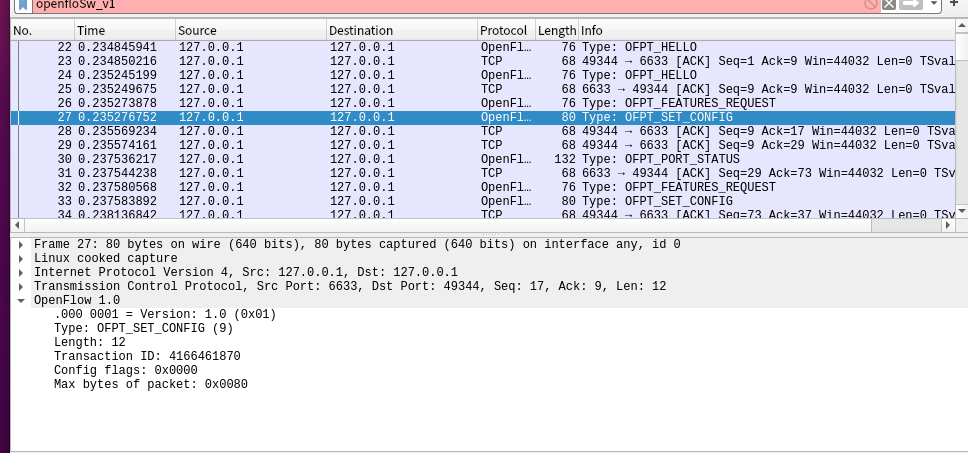
⚫flag:指示交换机如何处理IP分片数据包
⚫max bytes of packet:当交换机无法处理到达的数据包时,向控制器发送如何处理的最大字节数,本实验中控制器发送的值是0x0080,即128字节。
4、Port_Status
当交换机端口发生变化时,告知控制器相应的端口状态。
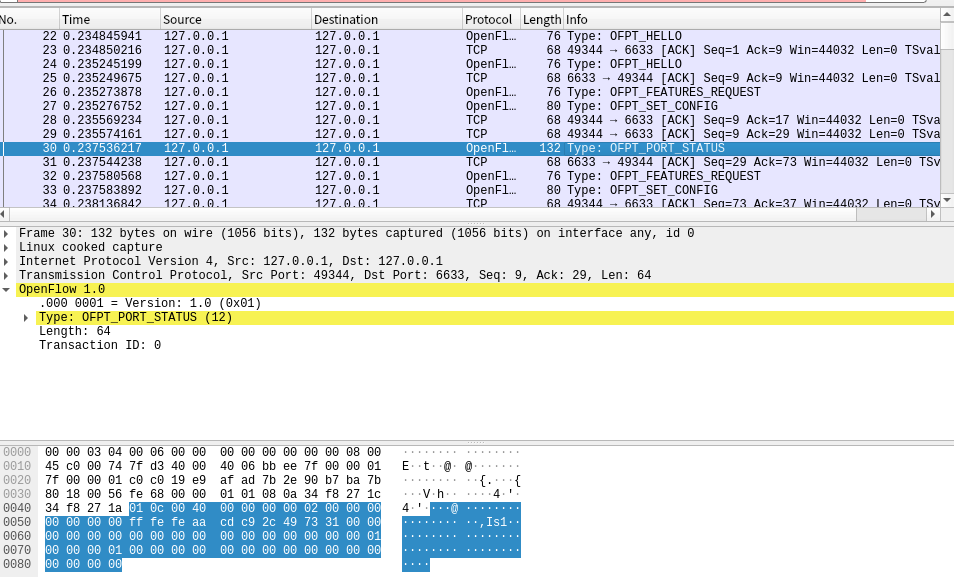
5、Features Reply
交换机49344端口(这是我的特征信息,请查收) ---> 控制器6633端口
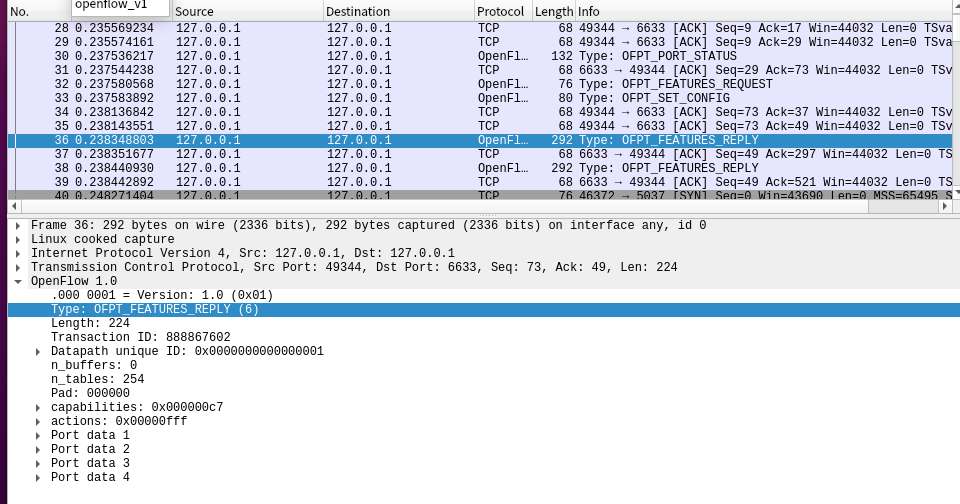
6、Packet_in
交换机49344端口(有数据包进来,请指示)--- 控制器6633端口
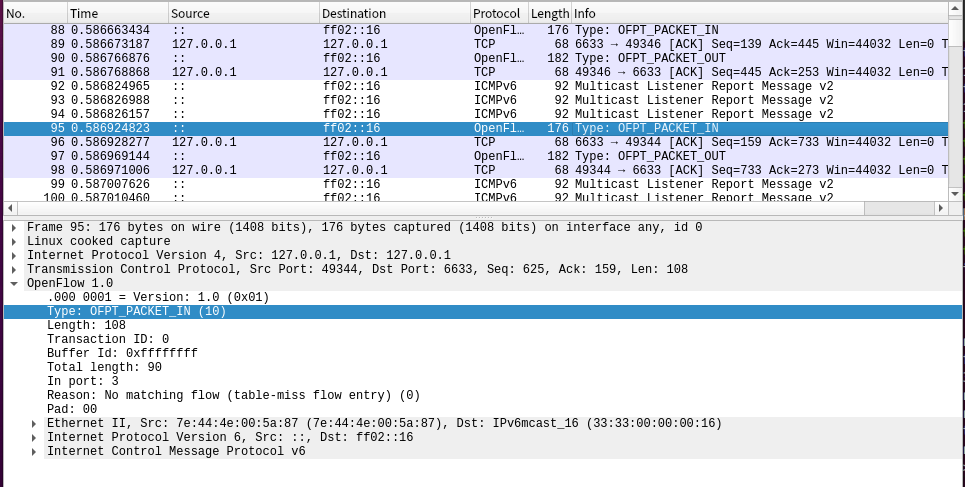
7、Flow_mod
分析抓取的flow_mod数据包,控制器通过6633端口向交换机49346端口、交换机49344端口 下发流表项,指导数据的转发处理
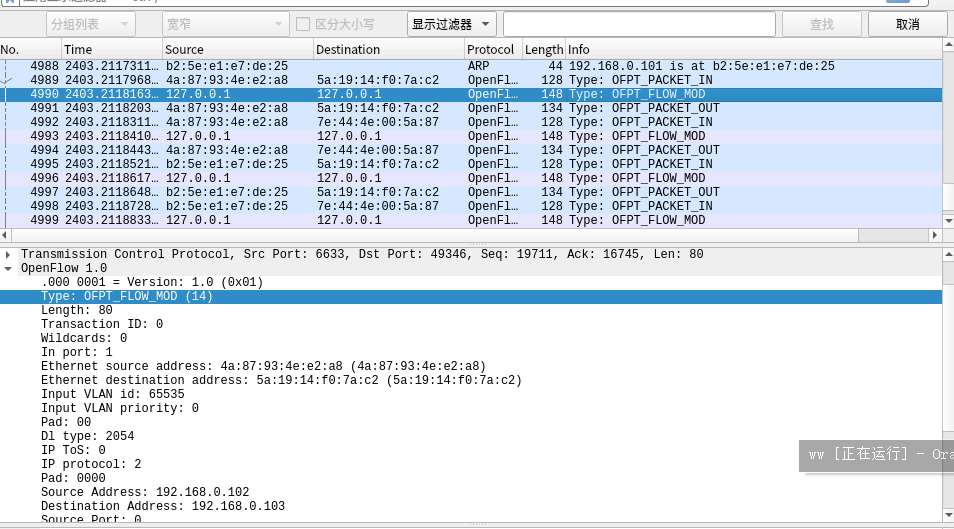
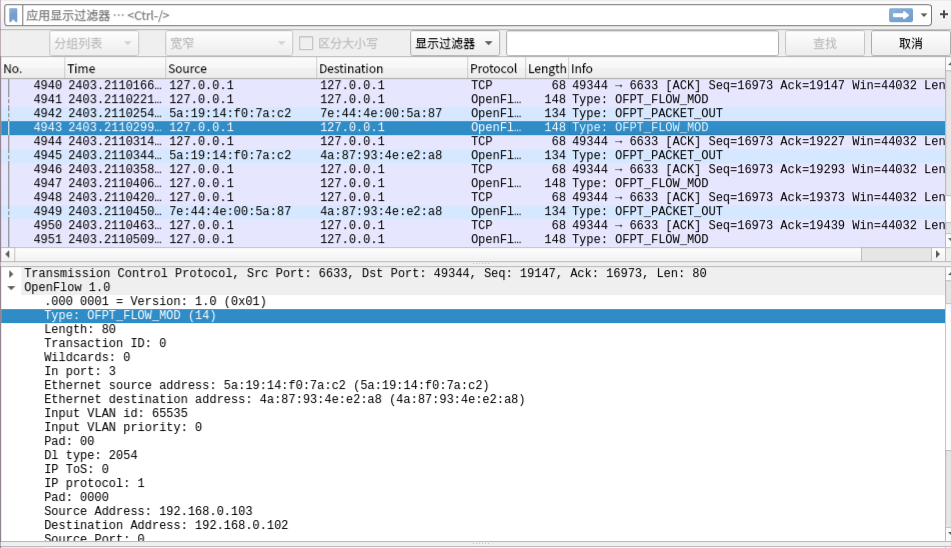
8、Packet_out
控制器6633端口(请按照我给你的action进行处理) ---> 交换机49346端口
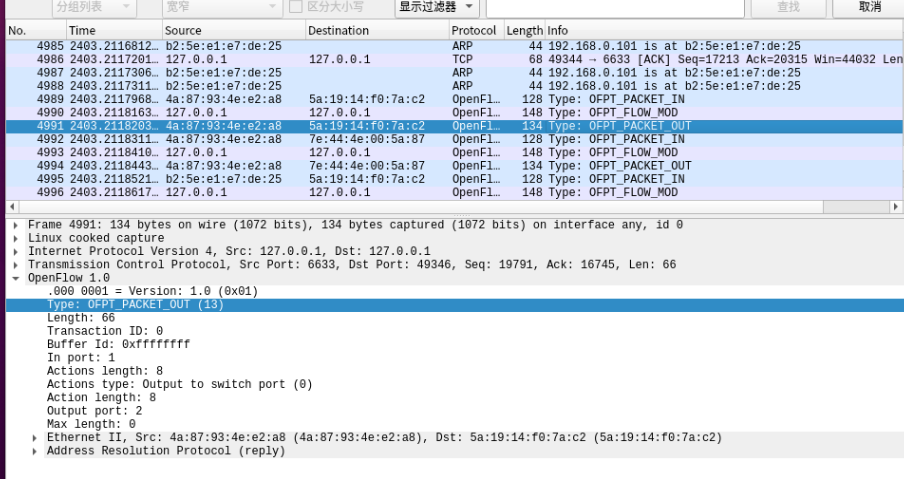
告诉输出到交换机的2端口
9、交互图
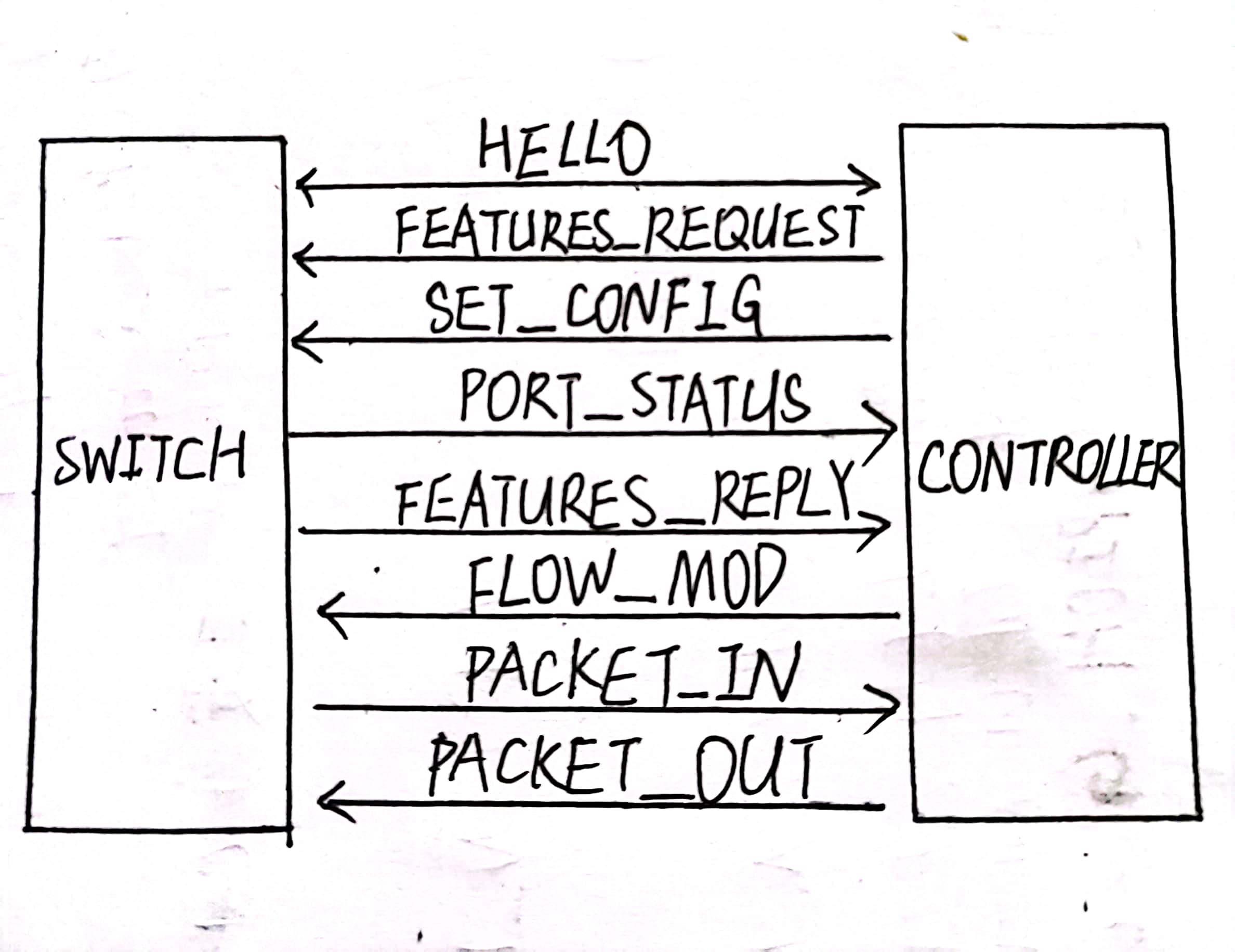
10、交换机与控制器建立通信时是使用TCP协议还是UDP协议?
交换机与控制器建立通信时是使用TCP协议。

进阶要求
1、hello
/* Header on all OpenFlow packets. */
struct ofp_header {
uint8_t version; /* OFP_VERSION. */
uint8_t type; /* One of the OFPT_ constants. */
uint16_t length; /* Length including this ofp_header. */
uint32_t xid; /* Transaction id associated with this packet.
Replies use the same id as was in the request
to facilitate pairing. */
};
OFP_ASSERT(sizeof(struct ofp_header) == 8);
/* OFPT_HELLO. This message has an empty body, but implementations must
* ignore any data included in the body, to allow for future extensions. */
struct ofp_hello {
struct ofp_header header;
};
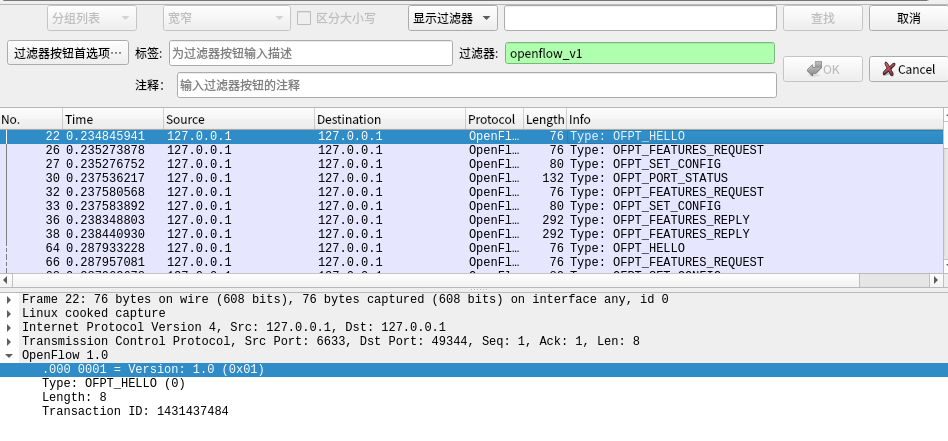
2、Features Request
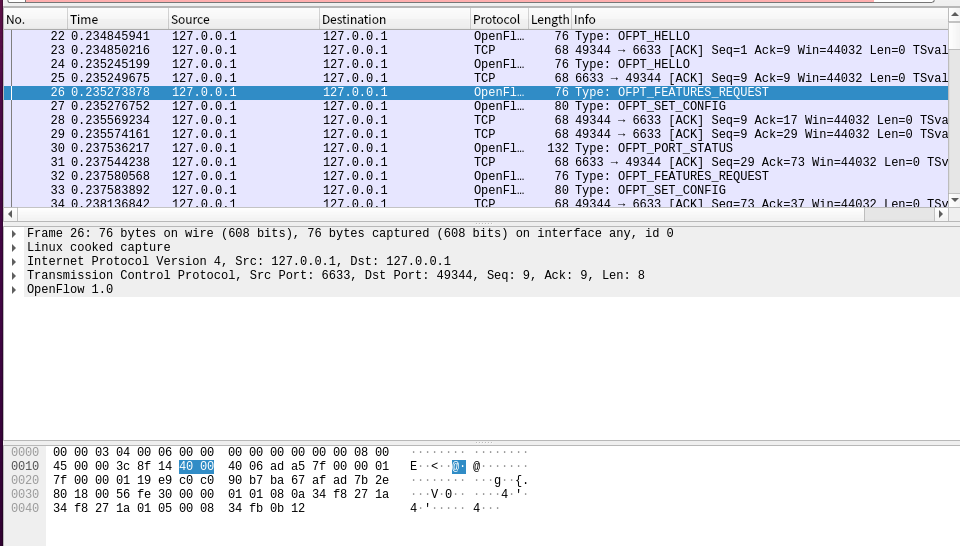
3、Set config
/* Switch configuration. */
struct ofp_switch_config {
struct ofp_header header;
uint16_t flags; /* OFPC_* flags. */
uint16_t miss_send_len; /* Max bytes of new flow that datapath should
send to the controller. */
};
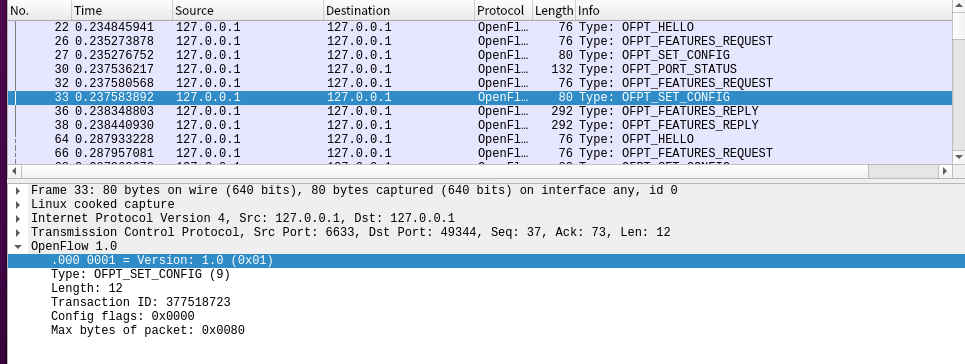
4、Port_Status
/* A physical port has changed in the datapath */
struct ofp_port_status {
struct ofp_header header;
uint8_t reason; /* One of OFPPR_*. */
uint8_t pad[7]; /* Align to 64-bits. */
struct ofp_phy_port desc;
};
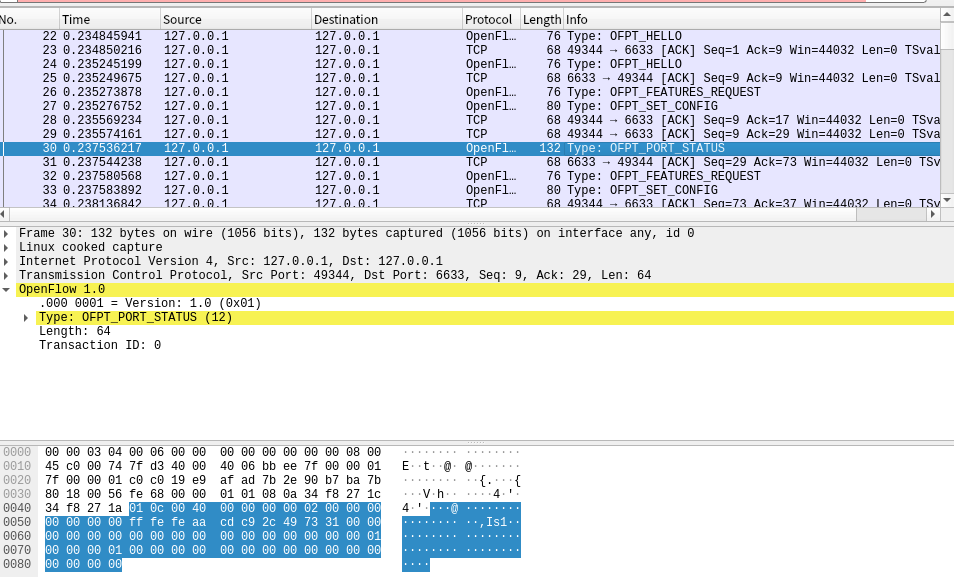
5、Features Reply
/* Description of a physical port */
struct ofp_phy_port {
uint16_t port_no;
uint8_t hw_addr[OFP_ETH_ALEN];
char name[OFP_MAX_PORT_NAME_LEN]; /* Null-terminated */
uint32_t config; /* Bitmap of OFPPC_* flags. */
uint32_t state; /* Bitmap of OFPPS_* flags. */
/* Bitmaps of OFPPF_* that describe features. All bits zeroed if
* unsupported or unavailable. */
uint32_t curr; /* Current features. */
uint32_t advertised; /* Features being advertised by the port. */
uint32_t supported; /* Features supported by the port. */
uint32_t peer; /* Features advertised by peer. */
};
OFP_ASSERT(sizeof(struct ofp_phy_port) == 48);
/* Switch features. */
struct ofp_switch_features {
struct ofp_header header;
uint64_t datapath_id; /* Datapath unique ID. The lower 48-bits are for
a MAC address, while the upper 16-bits are
implementer-defined. */
uint32_t n_buffers; /* Max packets buffered at once. */
uint8_t n_tables; /* Number of tables supported by datapath. */
uint8_t pad[3]; /* Align to 64-bits. */
/* Features. */
uint32_t capabilities; /* Bitmap of support "ofp_capabilities". */
uint32_t actions; /* Bitmap of supported "ofp_action_type"s. */
/* Port info.*/
struct ofp_phy_port ports[0]; /* Port definitions. The number of ports
is inferred from the length field in
the header. */
};
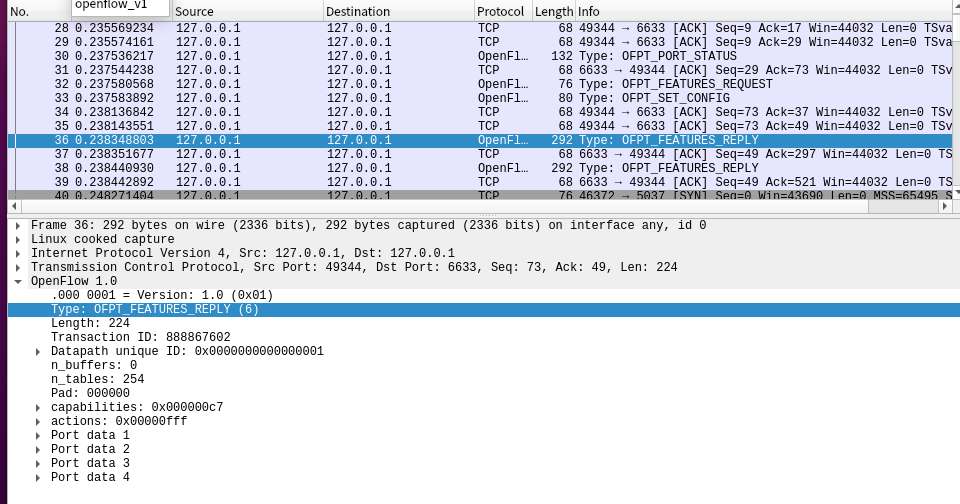
6、Packet_in
/* Why is this packet being sent to the controller? */
enum ofp_packet_in_reason {
OFPR_NO_MATCH, /* No matching flow. */
OFPR_ACTION /* Action explicitly output to controller. */
};
struct ofp_packet_in {
struct ofp_header header;
uint32_t buffer_id; /* ID assigned by datapath. */
uint16_t total_len; /* Full length of frame. */
uint16_t in_port; /* Port on which frame was received. */
uint8_t reason; /* Reason packet is being sent (one of OFPR_*) */
uint8_t pad;
uint8_t data[0]; /* Ethernet frame, halfway through 32-bit word,
so the IP header is 32-bit aligned. The
amount of data is inferred from the length
field in the header. Because of padding,
offsetof(struct ofp_packet_in, data) ==
sizeof(struct ofp_packet_in) - 2. */
};
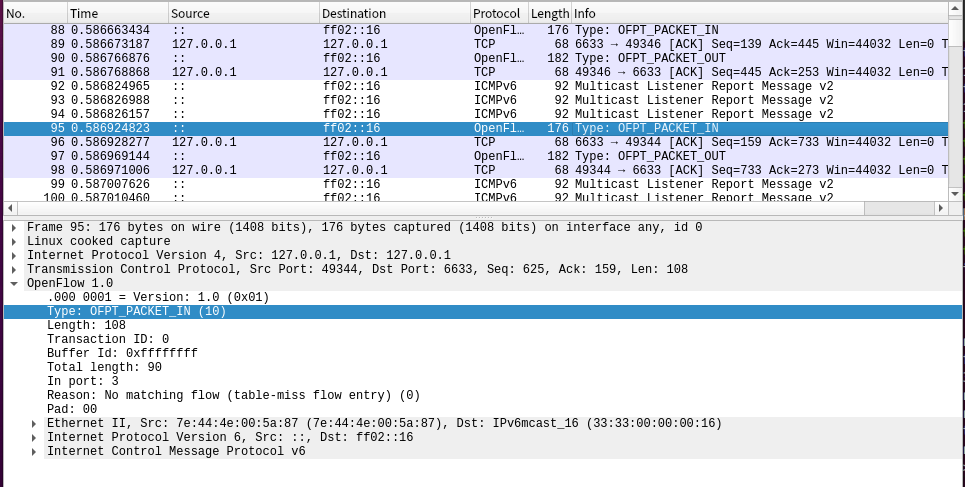
7、Flow_mod
struct ofp_flow_mod {
struct ofp_header header;
struct ofp_match match; /* Fields to match */
uint64_t cookie; /* Opaque controller-issued identifier. */
/* Flow actions. */
uint16_t command; /* One of OFPFC_*. */
uint16_t idle_timeout; /* Idle time before discarding (seconds). */
uint16_t hard_timeout; /* Max time before discarding (seconds). */
uint16_t priority; /* Priority level of flow entry. */
uint32_t buffer_id; /* Buffered packet to apply to (or -1).
Not meaningful for OFPFC_DELETE*. */
uint16_t out_port; /* For OFPFC_DELETE* commands, require
matching entries to include this as an
output port. A value of OFPP_NONE
indicates no restriction. */
uint16_t flags; /* One of OFPFF_*. */
struct ofp_action_header actions[0]; /* The action length is inferred
from the length field in the
header. */
};
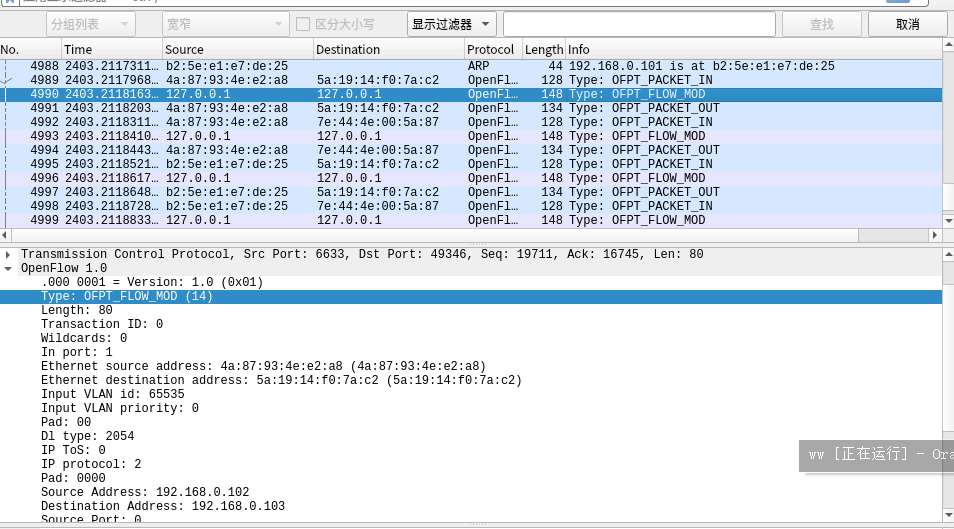
8、Packet_out
struct ofp_packet_out {
struct ofp_header header;
uint32_t buffer_id; /* ID assigned by datapath (-1 if none). */
uint16_t in_port; /* Packet's input port (OFPP_NONE if none). */
uint16_t actions_len; /* Size of action array in bytes. */
struct ofp_action_header actions[0]; /* Actions. */
/* uint8_t data[0]; */ /* Packet data. The length is inferred
from the length field in the header.
(Only meaningful if buffer_id == -1.) */
};
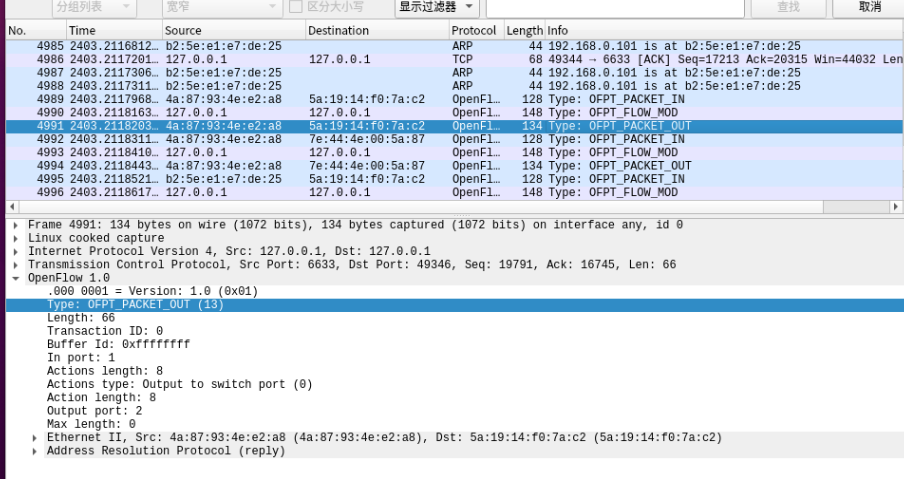
个人总结
这次实验主要就是利用wireshark来对OpenFlow协议数据交互过程进行抓包。相对于前几次实验来说,这次实验难度不大,就是需要比较耐心的去分析结构,还有就是在写报告的时候要用到很多的截图,这些截图是这个实验耗时的主要原因。在实验中的问题是,刚开始始终看不到flow_mod这个包,后来看到这个是一个数据包,就想到是不是没有pingall过的原因,后来pingall了一下,这个数据包就出来了。