chdir
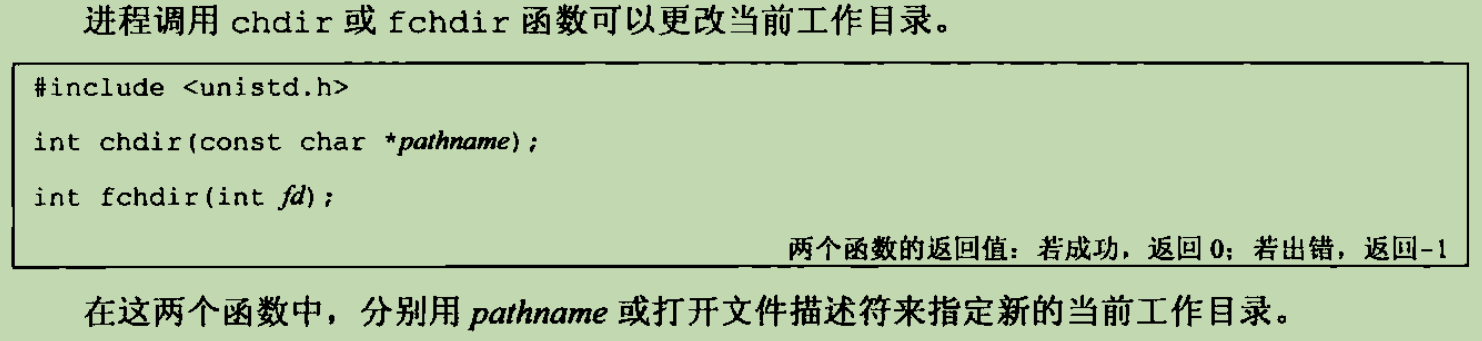
getcwd

#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#define MAX 512
int main(int argc, char * argv[])
{
// 方法一
char path[MAX];
path[0] = '\0';
getcwd(path, sizeof(path));
puts(path);
// 改变路径
if (chdir("/home") < 0)
{
perror("Error chdir.\n");
exit(EXIT_FAILURE);
}
// 方法二
char * buf = NULL;
buf = getcwd(NULL, 0);
puts(buf);
free(buf);
exit(EXIT_SUCCESS);
}
第二版
/*
API chdir \ getcwd
*/
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#define MAX 20
int main(int argc, char *argv[])
{
// getcwd,获取当前路径
char *path = NULL;
path = getcwd(NULL, 0);
getcwd(NULL, 0);
puts("Original path is :");
puts(path);
// 切换路径地址
if (chdir("../") < 0)
{
perror("Error chdir:");
exit(EXIT_FAILURE);
}
path = getcwd(NULL, 0);
puts("Now path is:");
puts(path);
free(path);
exit(EXIT_SUCCESS);
}
第三版
/*
API chdir \ getcwd
切换目录地址
*/
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
int main(int argc, char *argv[])
{
// getcwd,获取当前路径
char *path = NULL;
path = getcwd(NULL, 0);
getcwd(NULL, 0);
puts("Original path is :");
puts(path);
// 读取参数,目标路径
char * o_path = NULL;
o_path = argv[1];
puts("I want to munu:");
puts(o_path);
// 切换路径地址
if (chdir(o_path) < 0)
{
perror("Error chdir:");
exit(EXIT_FAILURE);
}
path = getcwd(NULL, 0);
puts("Now path is:");
puts(path);
free(path);
exit(EXIT_SUCCESS);
}
第四版
/*
API chdir \ getcwd
切换目录地址
*/
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
int main(int argc, char *argv[])
{
char o_path[20];
// getcwd,获取当前路径
char *path = NULL;
path = getcwd(NULL, 0);
getcwd(NULL, 0);
puts("Original path is :");
puts(path);
for(;;)
{
puts("I want to munu:");
scanf("%s", o_path);
puts(o_path);
if (strcmp(o_path, "q") == 0)
{
puts("quit");
break;
}
// 切换路径地址
if (chdir(o_path) < 0)
{
perror("Error chdir:");
exit(EXIT_FAILURE);
}
path = getcwd(NULL, 0);
puts("Now path is:");
puts(path);
}
free(path);
exit(EXIT_SUCCESS);
}
makefile
cshell:code.o
gcc -o cshell code.o
code.o:code.c
gcc -c code.c
.PHONY: clean
clean:
rm -f *.o
rm -f cshell