LeetCode-94 Binary Tree Inorder Traversal Solution (with Java)
1. Description: 
Notes:
2. Examples: 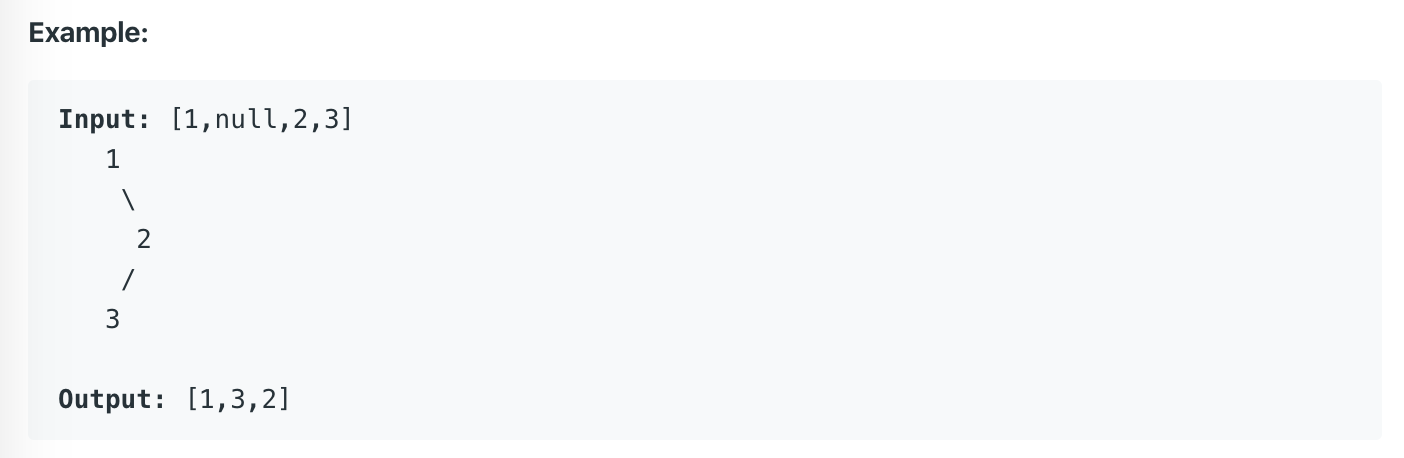
3.Solutions:
1 /** 2 * Created by sheepcore on 2019-05-09 3 * Definition for a binary tree node. 4 * public class TreeNode { 5 * int val; 6 * TreeNode left; 7 * TreeNode right; 8 * TreeNode(int x) { val = x; } 9 * } 10 */ 11 class Solution { 12 public List<Integer> inorderTraversal(TreeNode root) { 13 Stack<TreeNode> stack = new Stack<TreeNode>(); 14 List<Integer> res = new ArrayList<>(); 15 while (root != null || !stack.isEmpty()){ 16 while (root != null) { 17 stack.push(root); 18 root = root.left; 19 } 20 root = stack.pop(); 21 res.add(root.val); 22 root = root.right; 23 } 24 return res; 25 } 26 }