在Windows上运行单节点的Cassandra
Cassandra可以安裝在很多系统上, 我是安装在windows server 2008 R2上,安装相当简单,只要把下载下来的压缩包解压缩放到一个目录下就可以了,这里主要是记录下使用体验:
Cassandra官网: http://cassandra.apache.org/,下载页面 http://cassandra.apache.org/download/
Cassandra用java开发的,要求安装JVM 1.6以上,推荐 Version 6 Update 23 到Java官网下载 http://www.java.com/zh_CN/download/manual.jsp#win,要注意的是,Cassandra在windows上安装要设置两个系统参数:
- JAVA_HOME : 一般是 C:\Program Files\Java\jre6
- CASSANDRA_HOME : 看你解压缩到那个位置就写那个,我的是D:\apache-cassandra-0.7.0-rc4\
到Cassandra的bin下面运行cassandra.bat就会启动了,大概是这个样子:
在windows上Cassandra 不知道怎么设置成按Windows 服务方式运行,所以就另外开一个命令行来操作。
要确认Cassandra有没有再跑,可以用nodetool.bat这个工具进行确认。
因为只有一个节点,所以啥东西都不用配,直接用默认的 keyspace就可以玩了,Cassandra 提供了一个叫做 Cassandra CLI 的工具可以直接输入命令,运行cassadnra-cli.bat 就可以了了。我们就拿这个来试一下,Cassandra CLI 常用的命令有 set get show count,先拿set和get来做示例,还有quit/exit是离开 Cassandra CLI,也可以用 help 去查可用的命令,记得运行 cassandra-cli.bat 时要加个参数 --host 指定 cassandra node 的位置,不然就玩不转了。
然后,我们可以参考README.txt文件中提供的范例进行测试。Cassandra 0.7.0 rc3 已经没有了默认的Keyspace (EX:Keyspace1) ,使用之前需要创建。set的语法大概长的像下面这样:
01.set Keyspace1.Standard1['geffzhang']['blog'] = 'http://www.dotnetting.cn'
02. \ \ \ \ \
03. \ \ \_ key \ \_ value
04. \ \ \_ column
05. \_ keyspace \_ column family
就按照上面的语法来加几个数据进去,然后用get把数据拉出来看看
Cassandra在设计的时候,就是支持Thrift的,这意味着我们可以使用多种语言开发。对于Cassandra的开发本身而言,这是使用Thrift的好处:支持多语言。坏处也是显而易见的:Thrift API功能过于简单,不具备在生产环境使用的条件。
Cassandra 建议用户在它们的程序内用高阶API与Cassandrar进行通信,以C#来说,像是FluentCassandra 或 Aquiles。但是你也可以用官方出的最低阶API - Thrift 来与Cassandra沟通。
Thrift这个是Cassandra自带的最简单的一类API,这个文件在apache-cassandra-0.5.1.中包含了。可以直接使用。我们也可以自己安装一个Thrift,然后通过cassandra.thrift文件自动生成。如果你要使用Cassandra,那么我们必须要了解Thrift API,毕竟所有的其他更加高级的API都是基于这个来包装的。
Step 1 : Download Thrift
To Thrift Download Page get the latest stable release , for me , that is thrift-0.5.0.tar.gz (use for making thrift.dll) and Thrift compiler for Windows (thrift-0.5.0.exe) (for making Apache.Cassandra.dll).
Step 2 : Gnerate Thrift DLL
Unzip thrift-0.5.0.tar.gz (or new version) , find Thrift.csproj(or Thrift.sln) in \thrift\lib\csharp\src\
In Visual Studio , right click on [Thrift] -> [Build] , then you will get Thrift.dll in \bin\Debug\ (if no error)
Step 3 : Generate Apache.Cassandra DLL
Copy Thrift compiler for Windows (thrift-0.5.0.exe) to Cassandra Folder\interface\ , execute following command
thrift-0.5.0.exe --gen csharp cassandra.thrift
In principle, it should be generate a new folder called "gen-csharp", but dunno why , I had an error when I running it with version 0.6.8 , so I change cassandra version to 0.7.0 rc3 , then it passed.
After get C# source code , using Visual Studio create a new Class Libary project , then add all files in gen-csharp\Apache\Cassandra\ folder. Now build the project , maybe you will got 19~26 error, but don't worry, just some word-casing problem , do some upper case job can fixed it.
(EX: .count -> .Count )
Step 4 : Connecting Cassandra with C#
Add Thrift.dll and Apache.Cassandra.dll to your refence , you can use windows forms/console application/web application/or other , it doesn't matter .
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using Apache.Cassandra;
using Thrift.Transport;
using Thrift.Protocol;
namespace Cassandra_Console
{
class Program
{
static void Main(string[] args)
{
TTransport frameTransport = new TFramedTransport(new TSocket("localhost", 9160));
TProtocol protocol = new TBinaryProtocol(transport);
TProtocol frameProtocol = new TBinaryProtocol(frameTransport);
Cassandra.Client client = new Cassandra.Client(frameProtocol, frameProtocol);
frameTransport.Open();
Console.WriteLine("Opening connection");
//for 0.7.0 , need select keyspace at first
client.set_keyspace("Keyspace1");
Console.WriteLine("keyspace set to Keyspace1");
System.Text.Encoding utf8Encoding = System.Text.Encoding.UTF8;
long timeStamp = DateTime.Now.Millisecond;
ColumnPath nameColumnPath = new ColumnPath()
{
Column_family = "Standard2",
Column = utf8Encoding.GetBytes("name")
};
ColumnParent nameColumnParent = new ColumnParent()
{
Column_family = "Standard2"
};
Column nameColume = new Column()
{
Name = utf8Encoding.GetBytes("name"),
Value = utf8Encoding.GetBytes("Geff zhang"),
Timestamp=timeStamp
};
Console.WriteLine("Inserting name columns");
//insert data
client.insert(utf8Encoding.GetBytes("Geff"),
nameColumnParent,
nameColume,
ConsistencyLevel.ONE);
//using the key to get column 'name'
ColumnOrSuperColumn returnedColumn = client.get(utf8Encoding.GetBytes("Geff"), nameColumnPath, ConsistencyLevel.ONE);
Console.WriteLine("Column Data in Keyspace1/Standard1: name: {0}, value: {1}",
utf8Encoding.GetString(returnedColumn.Column.Name),
utf8Encoding.GetString(returnedColumn.Column.Value));
Console.WriteLine("Closing connection");
frameTransport.Close();
Console.ReadLine();
}
}
}
欢迎大家扫描下面二维码成为我的客户,扶你上云
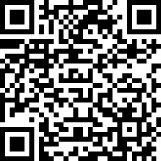