检查Python对象
编程环境中的对象很象现实世界中的对象。实际的对象有一定的形状、大小、重量和其它特征。实际的对象还能够对其环境进行响应、与其它对象交互或执行任务。计算机中的对象试图模拟我们身边现实世界中的对象,包括象文档、日程表和业务过程这样的抽象对象。
类似于实际的对象,几个计算机对象可能共享共同的特征,同时保持它们自己相对较小的变异特征。想一想您在书店中看到的书籍。书籍的每个物理副本都可能有污迹、几张破损的书页或唯一的标识号。尽管每本书都是唯一的对象,但都拥有相同标题的每本书都只是原始模板的实例,并保留了原始模板的大多数特征。
对于面向对象的类和类实例也是如此。例如,可以看到每个 Python 字符串都被赋予了一些属性, dir()
函数揭示了这些属性。在前一个示例中,我们定义了自己的 Person
类,它担任创建个别 Person 实例的模板,每个实例都有自己的 name 和 age 值,同时共享自我介绍的能力。这就是面向对象。
于是在计算机术语中,对象是拥有标识和值的事物,属于特定类型、具有特定特征和以特定方式执行操作。并且,对象从一个或多个父类继承了它们的许多属性。除了关键字和特殊符号(象运算符,如 +
、 -
、 *
、 **
、 /
、 %
、 <
、 >
等)外,Python 中的所有东西都是对象。Python 具有一组丰富的对象类型:字符串、整数、浮点、列表、元组、字典、函数、类、类实例、模块、文件等。
当您有一个任意的对象(也许是一个作为参数传递给函数的对象)时,可能希望知道一些关于该对象的情况。在本节中,我们将向您展示如何让 Python 对象回答如下问题:
- 对象的名称是什么?
- 这是哪种类型的对象?
- 对象知道些什么?
- 对象能做些什么?
- 对象的父对象是谁?
并非所有对象都有名称,但那些有名称的对象都将名称存储在其 __name__
属性中。注:名称是从对象而不是引用该对象的变量中派生的。下面这个示例着重说明了这种区别:
$ python Python 2.2.2 (#1, Oct 28 2002, 17:22:19) [GCC 3.2 (Mandrake Linux 9.0 3.2-1mdk)] on linux2 Type "help", "copyright", "credits" or "license" for more information. >>> dir() # The dir() function ['__builtins__', '__doc__', '__name__'] >>> directory = dir # Create a new variable >>> directory() # Works just like the original object ['__builtins__', '__doc__', '__name__', 'directory'] >>> dir.__name__ # What's your name? 'dir' >>> directory.__name__ # My name is the same 'dir' >>> __name__ # And now for something completely different '__main__'
模块拥有名称,Python 解释器本身被认为是顶级模块或主模块。当以交互的方式运行 Python 时,局部 __name__
变量被赋予值 '__main__'
。同样地,当从命令行执行 Python 模块,而不是将其导入另一个模块时,其 __name__
属性被赋予值 '__main__'
,而不是该模块的实际名称。这样,模块可以查看其自身的 __name__
值来自行确定它们自己正被如何使用,是作为另一个程序的支持,还是作为从命令行执行的主应用程序。因此,下面这条惯用的语句在 Python 模块中是很常见的:
清单 2. 用于执行或导入的测试
if __name__ == '__main__': # Do something appropriate here, like calling a # main() function defined elsewhere in this module. main() else: # Do nothing. This module has been imported by another # module that wants to make use of the functions, # classes and other useful bits it has defined.
type()
函数有助于我们确定对象是字符串还是整数,或是其它类型的对象。它通过返回类型对象来做到这一点,可以将这个类型对象与 types
模块中定义的类型相比较:
清单 3. 我是您的类型吗?
>>> import types >>> print types.__doc__ Define names for all type symbols known in the standard interpreter. Types that are part of optional modules (e.g. array) are not listed. >>> dir(types) ['BufferType', 'BuiltinFunctionType', 'BuiltinMethodType', 'ClassType', 'CodeType', 'ComplexType', 'DictProxyType', 'DictType', 'DictionaryType', 'EllipsisType', 'FileType', 'FloatType', 'FrameType', 'FunctionType', 'GeneratorType', 'InstanceType', 'IntType', 'LambdaType', 'ListType', 'LongType', 'MethodType', 'ModuleType', 'NoneType', 'ObjectType', 'SliceType', 'StringType', 'StringTypes', 'TracebackType', 'TupleType', 'TypeType', 'UnboundMethodType', 'UnicodeType', 'XRangeType', '__builtins__', '__doc__', '__file__', '__name__'] >>> s = 'a sample string' >>> type(s) <type 'str'> >>> if type(s) is types.StringType: print "s is a string" ... s is a string >>> type(42) <type 'int'> >>> type([]) <type 'list'> >>> type({}) <type 'dict'> >>> type(dir) <type 'builtin_function_or_method'>
先前我们说过,每个对象都有标识、类型和值。值得注意的是,可能有多个变量引用同一对象,同样地,变量可以引用看起来相似(有相同的类型和值),但拥有截然不同标识的多个对象。当更改对象时(如将某一项添加到列表),这种关于对象标识的概念尤其重要,如在下面的示例中, blist
和 clist
变量引用同一个列表对象。正如您在示例中所见, id()
函数给任何给定对象返回唯一的标识符:
清单 4. 目的地……
>>> print id.__doc__ id(object) -> integer Return the identity of an object. This is guaranteed to be unique among simultaneously existing objects. (Hint: it's the object's memory address.) >>> alist = [1, 2, 3] >>> blist = [1, 2, 3] >>> clist = blist >>> clist [1, 2, 3] >>> blist [1, 2, 3] >>> alist [1, 2, 3] >>> id(alist) 145381412 >>> id(blist) 140406428 >>> id(clist) 140406428 >>> alist is blist # Returns 1 if True, 0 if False 0 >>> blist is clist # Ditto 1 >>> clist.append(4) # Add an item to the end of the list >>> clist [1, 2, 3, 4] >>> blist # Same, because they both point to the same object [1, 2, 3, 4] >>> alist # This one only looked the same initially [1, 2, 3]
我们已经看到对象拥有属性,并且 dir()
函数会返回这些属性的列表。但是,有时我们只想测试一个或多个属性是否存在。如果对象具有我们正在考虑的属性,那么通常希望只检索该属性。这个任务可以由 hasattr()
和 getattr()
函数来完成,如本例所示:
>>> print hasattr.__doc__ hasattr(object, name) -> Boolean Return whether the object has an attribute with the given name. (This is done by calling getattr(object, name) and catching exceptions.) >>> print getattr.__doc__ getattr(object, name[, default]) -> value Get a named attribute from an object; getattr(x, 'y') is equivalent to x.y. When a default argument is given, it is returned when the attribute doesn't exist; without it, an exception is raised in that case. >>> hasattr(id, '__doc__') 1 >>> print getattr(id, '__doc__') id(object) -> integer Return the identity of an object. This is guaranteed to be unique among simultaneously existing objects. (Hint: it's the object's memory address.)
可以调用表示潜在行为(函数和方法)的对象。可以用 callable()
函数测试对象的可调用性:
>>> print callable.__doc__ callable(object) -> Boolean Return whether the object is callable (i.e., some kind of function). Note that classes are callable, as are instances with a __call__() method. >>> callable('a string') 0 >>> callable(dir) 1
在 type()
函数提供对象的类型时,还可以使用 isinstance()
函数测试对象,以确定它是否是某个特定类型或定制类的实例:
>>> print isinstance.__doc__ isinstance(object, class-or-type-or-tuple) -> Boolean Return whether an object is an instance of a class or of a subclass thereof. With a type as second argument, return whether that is the object's type. The form using a tuple, isinstance(x, (A, B, ...)), is a shortcut for isinstance(x, A) or isinstance(x, B) or ... (etc.). >>> isinstance(42, str) 0 >>> isinstance('a string', int) 0 >>> isinstance(42, int) 1 >>> isinstance('a string', str) 1
我们先前提到过,定制类的实例从该类继承了属性。在类这一级别,可以根据一个类来定义另一个类,同样地,这个新类会按照层次化的方式继承属性。Python 甚至支持多重继承,多重继承意味着可以用多个父类来定义一个类,这个新类继承了多个父类。 issubclass()
函数使我们可以查看一个类是不是继承了另一个类:
>>> print issubclass.__doc__ issubclass(C, B) -> Boolean Return whether class C is a subclass (i.e., a derived class) of class B. >>> class SuperHero(Person): # SuperHero inherits from Person... ... def intro(self): # but with a new SuperHero intro ... """Return an introduction.""" ... return "Hello, I'm SuperHero %s and I'm %s." % (self.name, self.age) ... >>> issubclass(SuperHero, Person) 1 >>> issubclass(Person, SuperHero) 0 >>>
欢迎大家扫描下面二维码成为我的客户,扶你上云
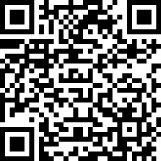