idea 中使用Mybatis Generator逆向工程生成代码
通过MAVEN完成 Mybatis 逆向工程
1. POM文件中添加插件
在 pom 文件的build 标签中 添加 plugin 插件和 数据库连接 jdbc 的依赖。
<build> <plugins> <plugin> <groupId>org.mybatis.generator</groupId> <artifactId>mybatis-generator-maven-plugin</artifactId> <version>1.4.0</version> <dependencies> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <version>8.0.13</version> </dependency> </dependencies> <configuration> <!-- 输出详细信息 --> <verbose>true</verbose> <!-- 覆盖生成文件 --> <overwrite>true</overwrite> <!-- 定义配置文件 --> <configurationFile>${basedir}/src/main/resources/generatorConfig.xml</configurationFile> </configuration> </plugin> </plugins> </build>
若不在pom文件中引入数据库连接依赖,也可在配置文件中通过本地方式启动连接。
2. 在自己定义的位置上添加配置文件 generatorConfig.xml
1 <?xml version="1.0" encoding="UTF-8"?> 2 <!DOCTYPE generatorConfiguration 3 PUBLIC "-//mybatis.org//DTD MyBatis Generator Configuration 1.0//EN" 4 "http://mybatis.org/dtd/mybatis-generator-config_1_0.dtd"> 5 <generatorConfiguration> 6 <!-- 若想单独配置属性,可将其配入properties后 通过此方式导入属性 ${userId} --> 7 <!-- <properties resource="generator.properties"></properties>--> 8 9 <!-- 数据库驱动: 若之前未在build里配置数据库驱动包,可选择本地硬盘上面的数据库驱动包--> 10 <classPathEntry location="D:\Maven\repository\mysql\mysql-connector-java\5.1.38\mysql-connector-java-5.1.38.jar"/> 11 12 <!-- targetRuntime 默认为MyBatis3DynamicSql,该值不会生成xml文件, 可选择Mybatis3 --> 13 <context id="default" targetRuntime="Mybatis3"> 14 15 <!-- optional,旨在创建class时,对注释进行控制 --> 16 <commentGenerator> 17 <!-- 是否去除自动生成的注释 true:是 : false:否 --> 18 <property name="suppressAllComments" value="true" /> 19 </commentGenerator> 20 21 <!-- 配置数据库连接 --> 22 <jdbcConnection 23 driverClass="com.mysql.jdbc.Driver" 24 connectionURL="jdbc:mysql://localhost:3306/test?serverTimezone=Asia/Shanghai" 25 userId="root" 26 password="123456"> 27 </jdbcConnection> 28 29 <!-- 非必需,类型处理器,在数据库类型和java类型之间的转换控制--> 30 <javaTypeResolver > 31 <property name="forceBigDecimals" value="false" /> 32 </javaTypeResolver> 33 34 <!-- Model模型生成器,用来生成含有主键key的类,记录类 以及查询Example类 35 targetPackage 指定生成的model生成所在的包名 36 targetProject 指定在该项目下所在的路径 37 --> 38 <javaModelGenerator targetPackage="com.demo.dao.pojo" targetProject="src/main/java"> 39 <!-- 是否允许子包,即targetPackage.schemaName.tableName --> 40 <property name="enableSubPackages" value="true"/> 41 <!-- 是否对model添加 构造函数 --> 42 <property name="constructorBased" value="false"/> 43 <!-- 是否对类CHAR类型的列的数据进行trim操作 --> 44 <property name="trimStrings" value="false"/> 45 <!-- 建立的Model对象是否 不可改变 即生成的Model对象不会有 setter方法,只有构造方法 --> 46 <property name="immutable" value="true"/> 47 </javaModelGenerator> 48 49 <!-- 生成映射文件的包名和位置--> 50 <sqlMapGenerator targetPackage="mapper" targetProject="src/main/resources"> 51 <property name="enableSubPackages" value="false"/> 52 </sqlMapGenerator> 53 54 <!-- 客户端代码,生成易于使用的针对Model对象和XML配置文件 的代码 55 type="ANNOTATEDMAPPER",生成Java Model 和基于注解的Mapper对象 56 type="MIXEDMAPPER",生成基于注解的Java Model 和相应的Mapper对象 57 type="XMLMAPPER",生成SQLMap XML文件和独立的Mapper接口 58 --> 59 <javaClientGenerator targetPackage="com.demo.dao.mapper" targetProject="src/main/java" type="XMLMAPPER"> 60 <property name="enableSubPackages" value="false"/> 61 </javaClientGenerator> 62 <table tableName="aging_demotion" domainObjectName="AgingDemotion" 63 enableCountByExample="false" enableUpdateByExample="false" 64 enableDeleteByExample="false" enableSelectByExample="false" 65 selectByExampleQueryId="false"> 66 <!-- 插入时自动返回主键ID --> 67 <generatedKey column="aging_demotion_id" sqlStatement="Mysql" identity="true" /> 68 </table> 69 70 <table tableName="aging_listener" domainObjectName="AgingListener" 71 enableCountByExample="false" enableUpdateByExample="false" 72 enableDeleteByExample="false" enableSelectByExample="false" 73 selectByExampleQueryId="false"> 74 </table> 75 76 <table tableName="aging_state" domainObjectName="AgingState" 77 enableCountByExample="false" enableUpdateByExample="false" 78 enableDeleteByExample="false" enableSelectByExample="false" 79 selectByExampleQueryId="false"> 80 </table> 81 </context> 82 </generatorConfiguration>
3.通过maven启动
点击mybatis-generate:generate即可生成对应 java,mapper 和 pojo实体类。(若maven没有显示此插件,可点击左上角刷新)
通过generatedKey 使其插入时返回ID,其值必须为数值型自增主键。
其逆向生成的代码为:
<selectKey keyProperty="agingDemotionId" order="AFTER" resultType="java.lang.Long"> SELECT LAST_INSERT_ID() </selectKey>
也可自己通过这种方式实现返回自增ID。
<insert id="insert" useGeneratedKeys="true" keyProperty="agingDemotionId" parameterType="com.jd.aging.presentation.domain.AgingDemotionEntity">
这种方式只适用于传入对象时,insert方法成功依旧返回的是 1, 不过传入的实体类对象中 主键 ID 的值 不再为 null, 而是获得该插入实体类的主键ID值。
如果有错误或者更优化的解决方案,欢迎大家在评论区留言探讨。
也可以给我的个人公众号私信留言。
作者:殇央 © 转载请注明出处。
-------------------------------------------
如果有错误或者更优化的解决方案,欢迎大家在评论区留言探讨。
也可以给我的个人公众号私信留言。
如果觉得这篇文章对你有小小的帮助的话,记得在右下角点个“推荐”哦,博主在此感谢!
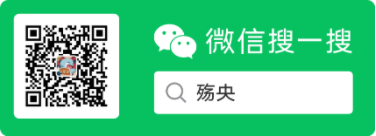