前言
- 本文只做记录,不做具体源码解析。
- 博主也是初学,系列文章只做入门,不建议当做范文。
代码实现
项目结构
GlobalException.java
是全局异常处理类
MyJwtException.java
是自定义的异常处理类
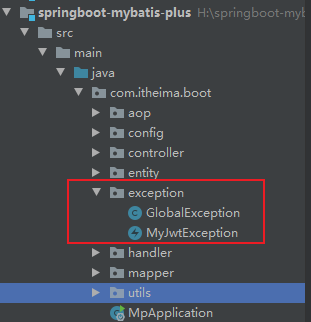
GlobalException.java
实现全局异常捕获并返回json。
package com.itheima.boot.exception;
import com.itheima.boot.utils.Resp;
import lombok.extern.slf4j.Slf4j;
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.context.request.RequestContextHolder;
import org.springframework.web.context.request.ServletRequestAttributes;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.io.PrintWriter;
import java.io.StringWriter;
@ControllerAdvice
@ResponseBody
@Slf4j
public class GlobalException {
/**
* 全局异常捕获
*/
@ExceptionHandler(Exception.class)
public Resp exceptionHandler(Exception e) {
WriteException(e);
return Resp.error().msg(e.getMessage());
}
@ExceptionHandler(MyJwtException.class)
public Resp JwtExceptionHandler(Exception e) {
// 获取response重写状态码
ServletRequestAttributes requestAttributes = (ServletRequestAttributes) RequestContextHolder.getRequestAttributes();
assert requestAttributes != null;
HttpServletResponse response = requestAttributes.getResponse();
assert response != null;
response.setStatus(403);
// 写异常日志
WriteException(e);
return Resp.error().code(40300).msg("token校验失败!");
}
/**
* 把异常写进日志
*/
private void WriteException(Exception e) {
StringWriter sw = null;
PrintWriter pw = null;
try {
sw = new StringWriter();
pw = new PrintWriter(sw);
e.printStackTrace(pw);
log.error(sw.toString());
} finally {
if (sw != null) {
try {
sw.close();
} catch (IOException e1) {
e1.printStackTrace();
}
}
if (pw != null) {
pw.close();
}
}
}
}
MyJwtException.java
是自定义的异常类,继承RuntimeException实现。
package com.itheima.boot.exception;
public class MyJwtException extends RuntimeException {
public MyJwtException(String message) {
super(message);
}
}
业务中需要的位置抛出MyJwtException
try {
int a = 10/0;
}catch(Exception e) {
throw new MyJwtException ("token验证失败");
}