实验4 8086标志寄存器及中断
实验任务1
1 assume cs:code, ds:data 2 3 data segment 4 x dw 1020h, 2240h, 9522h, 5060h, 3359h, 6652h, 2530h, 7031h 5 y dw 3210h, 5510h, 6066h, 5121h, 8801h, 6210h, 7119h, 3912h 6 data ends 7 code segment 8 start: 9 mov ax, data 10 mov ds, ax 11 mov si, offset x 12 mov di, offset y 13 call add128 14 15 mov ah, 4ch 16 int 21h 17 18 add128: 19 push ax 20 push cx 21 push si 22 push di 23 24 sub ax, ax 25 26 mov cx, 8 27 s: mov ax, [si] 28 adc ax, [di] 29 mov [si], ax 30 31 inc si 32 inc si 33 inc di 34 inc di 35 loop s 36 37 pop di 38 pop si 39 pop cx 40 pop ax 41 ret 42 code ends 43 end start
① line31~line34的4条inc指令,能否替换成如下代码?你的结论的依据/理由是什么?
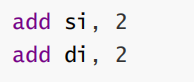
不能替换成如下代码。
ADC在求和运算时会加入了CF标志位。ADD指令在运算时可能会产生进位,即改变CF的值,会影响ADC
的结果,而INC指令将影响SF,AF,ZF,PF,OF标志位,但是不影响CF标志位。
② 在debug中调试,观察数据段中做128位加之前,和,加之后,数据段的值的变化。
实验任务2
1 assume cs:code, ds:data 2 data segment 3 str db 80 dup(?) 4 data ends 5 6 code segment 7 start: 8 mov ax, data 9 mov ds, ax 10 mov si, 0 11 s1: 12 mov ah, 1 13 int 21h 14 mov [si], al 15 cmp al, '#' 16 je next 17 inc si 18 jmp s1 19 next: 20 mov ah, 2 21 mov dl, 0ah 22 int 21h 23 24 mov cx, si 25 mov si, 0 26 s2: mov ah, 2 27 mov dl, [si] 28 int 21h 29 inc si 30 loop s2 31 32 mov ah, 4ch 33 int 21h 34 code ends 35 end start
① 汇编指令代码line11-18,实现的功能是?
循环键入字符,当键入“#”时跳出循环。
cmp指令实现两个操作数相减,运算结果会影响标志位CF, ZF, SF, OF, PF,当操作数相同时ZF零标志位置1,
此时je指令跳转至next标记处。
② 汇编指令代码line20-22,实现的功能是?
输出“回车”。
③ 汇编指令代码line24-30,实现的功能是?
循环输出键入的字符。
实验任务3
1 assume cs:code, ds:data 2 3 data segment 4 x dw 91, 792, 8536, 65521, 2021 5 len equ $ - x 6 data ends 7 8 code segment 9 start: 10 mov ax, data 11 mov ds, ax 12 13 mov cx, len/2 14 mov si, offset x 15 print: 16 mov ax, [si] 17 inc si 18 mov dx, [si] 19 inc si 20 call printNumber 21 call printSpace 22 loop print 23 24 mov ah, 4ch 25 int 21h 26 27 printNumber: 28 push cx 29 mov cx, 0 30 31 save: 32 mov dx, 0 33 mov bx, 10 34 div bx 35 36 push dx 37 inc cx 38 39 cmp ax, 0 40 jne save 41 42 print_save: 43 44 pop bx 45 mov dl, bl 46 mov ah, 2 47 or dl, 30h 48 int 21h 49 50 loop print_save 51 52 pop cx 53 ret 54 55 56 printSpace: 57 push ax 58 push dx 59 60 mov ah, 2 61 mov dl, ' ' 62 int 21h 63 64 pop dx 65 pop ax 66 ret 67 68 code ends 69 end start

实验任务4
1 assume cs:code, ds:data 2 data segment 3 str db "assembly language, it's not difficult but tedious" 4 len equ $ - str 5 data ends 6 7 code segment 8 start: 9 mov ax, data 10 mov ds, ax 11 mov si, offset str 12 mov cx, len 13 14 s: call strupr 15 16 mov ah, 4ch 17 int 21h 18 19 strupr: 20 s1: mov al, [si] 21 cmp al, 'a' 22 jb s2 23 cmp al, 'z' 24 ja s2 25 sub byte ptr [si], 20h 26 s2: inc si 27 loop s1 28 ret 29 30 code ends 31 end start
实验任务5
1 assume cs:code, ds:data 2 3 data segment 4 str1 db "yes", '$' 5 str2 db "no", '$' 6 data ends 7 8 code segment 9 start: 10 mov ax, data 11 mov ds, ax 12 13 mov ah, 1 14 int 21h 15 16 mov ah, 2 17 mov bh, 0 18 mov dh, 24 19 mov dl, 70 20 int 10h 21 22 cmp al, '7' 23 je s1 24 mov ah, 9 25 mov dx, offset str2 26 int 21h 27 28 jmp over 29 30 s1: mov ah, 9 31 mov dx, offset str1 32 int 21h 33 over: 34 mov ah, 4ch 35 int 21h 36 code ends 37 end start
程序的功能是判断输入的字符是否为7,若为7则在指定行输出yes,否则输出no。
实验任务6
task6_1.asm
1 assume cs:code 2 3 code segment 4 start: 5 ; 42 interrupt routine install code 6 mov ax, cs 7 mov ds, ax 8 mov si, offset int42 ; set ds:si 9 10 mov ax, 0 11 mov es, ax 12 mov di, 200h ; set es:di 13 14 mov cx, offset int42_end - offset int42 15 cld 16 rep movsb 17 18 ; set IVT(Interrupt Vector Table) 19 mov ax, 0 20 mov es, ax 21 mov word ptr es:[42*4], 200h 22 mov word ptr es:[42*4+2], 0 23 24 mov ah, 4ch 25 int 21h 26 27 int42: 28 jmp short int42_start 29 str db "welcome to 2049!" 30 len equ $ - str 31 32 ; display string "welcome to 2049!" 33 int42_start: 34 mov ax, cs 35 mov ds, ax 36 mov si, 202h 37 38 mov ax, 0b800h 39 mov es, ax 40 mov di, 24*160 + 32*2 41 42 mov cx, len 43 s: mov al, [si] 44 mov es:[di], al 45 mov byte ptr es:[di+1], 2 46 inc si 47 add di, 2 48 loop s 49 50 iret 51 int42_end: 52 nop 53 code ends 54 end start
task6_2.asm
1 assume cs:code 2 3 code segment 4 start: 5 int 42 6 7 mov ah, 4ch 8 int 21h 9 code ends 10 end start
中断:CPU在接收到外部发送的或内部产生的一种特殊信息时,会立即处理特殊信息。
软中断:CPU内部产生特殊信息(如除法错误、单步执行、执行into指令和执行int指令)时,立即处理该情况。