实验一 类与对象
#ifndef Complex_HPP #define Complex_HPP #include<iostream> class Complex{ private:double real,imag; public:Complex(){} Complex(double x1):real(x1),imag(0){} Complex(double x1,double x2):real(x1),imag(x2 ){} Complex(Complex &c1):real(c1.real ),imag(c1.imag ) {} ~Complex() {}; double get_real() const { return real ;} double get_imag() const { return imag ;} void show() const { if(imag>=0) std::cout<<real<<"+"<<imag<<"i"; else std::cout<<real<<imag<<"i";} void add(const Complex &c2) { real+=c2.real;imag+=c2.imag;} friend Complex add(const Complex &c1,const Complex &c2) ; friend bool is_equal(const Complex &c1,const Complex &c2); friend double abs(const Complex &c); }; bool is_equal (const Complex &c1,const Complex &c2) { if(c1.real==c2.real&&c1.imag==c2.imag) return true ; else return false ; } double abs(const Complex &c) { double m; m=sqrt(c.real *c.real +c.imag *c.imag ); return m; } Complex add(const Complex &c1,const Complex &c2) { Complex c3; c3.imag =c1.imag +c2.imag ; c3.real =c1.real +c2.real ; return c3;
}
#endif
#include<iostream>
#include"Complex.hpp"
int main()
{
using namespace std;
Complex c1(3, -4);
const Complex c2(4.5);
Complex c3(c1);
cout << "c1 = ";
c1.show();
cout << endl;
cout << "c2 = ";
c2.show();
cout << endl;
cout << "c2.imag = " << c2.get_imag() << endl;
cout << "c3 = ";
c3.show();
cout << endl;
cout << "abs(c1) = ";
cout << abs(c1) << endl;
cout << boolalpha;
cout << "c1 == c3 : " << is_equal(c1, c3) << endl;
cout << "c1 == c2 : " << is_equal(c1, c2) << endl;
Complex c4;
c4 = add(c1, c2);
cout << "c4 = c1 + c2 = ";
c4.show();
cout << endl;
c1.add(c2);
cout << "c1 += c2, " << "c1 = ";
c1.show();
cout << endl;
}
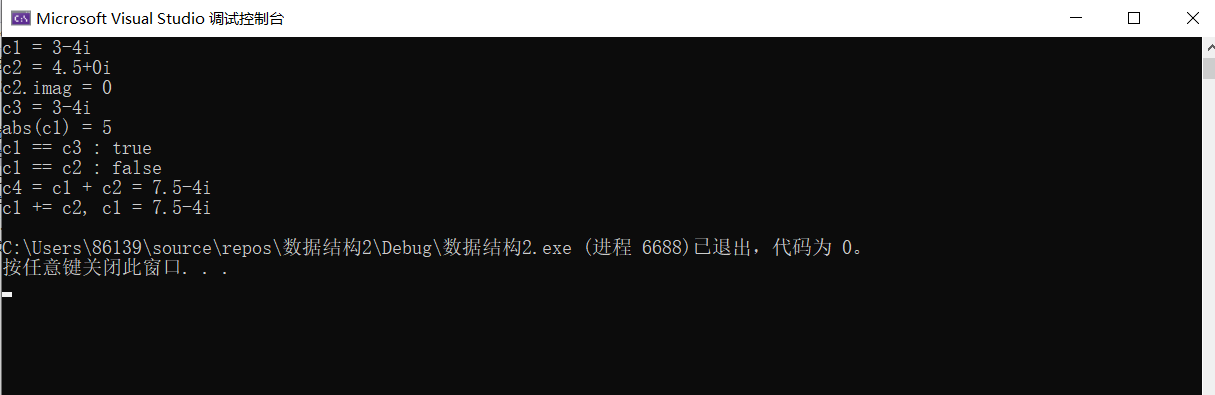
#ifndef User_HPP
#define User_HPP
#include<iostream>
#include<cmath>
#include<string>
using namespace std;
class User {
private:string name, passwd, email; static int count;
public:User() {}
User(string name1, string passwd1 = "111111", string email1 = "") :name(name1), passwd(passwd1), email(email1) { count++; }
~User() {}
void set_email() { cout << "Enter email address:"; cin >> email; cout << "email is set sucessful..." << endl; }
void change_passwd() {
cout << "Enter old password:";
int i = 3;
while (i--)
{
cin >> passwd;
if (passwd == "111111") { break; cout << "Enter new passwd:"; cin >> passwd; cout << "new passwd is set sucessfully..." << endl; }
else {
if (i == 0) cout << "password input errro.Please try after a while." << endl;
else cout << "password input errro.Please re-enter again: ";
}
}
}
void print_info() {
int mid = size(passwd); string m = "";
if (passwd == "111111") m = "******";
else while (mid--) m = m + "*";
cout << "name: " << name << endl
<< "passwd: " << m << endl
<< "email: " << email << endl;
}
static void print_n() { cout << "therer are " << count << " uers." << endl; }
};
int User::count = 0;
#endif
#include "User.hpp"
#include <iostream>
int main()
{
using namespace std;
cout << "testing 1......" << endl;
User user1("Jonny", "92197", "xyz@hotmail.com");
user1.print_info();
cout << endl
<< "testing 2......" << endl
<< endl;
User user2("Leonard");
user2.change_passwd();
user2.set_email();
user2.print_info();
User::print_n();
}
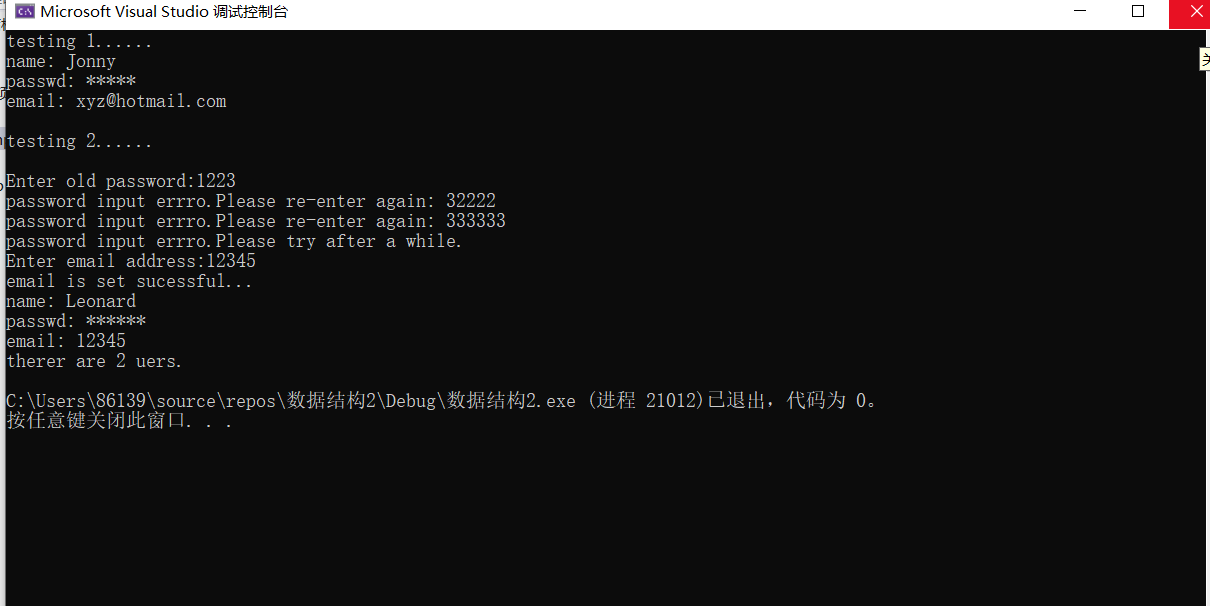