Django微讲解(十一)
Django微讲解(十一)
csrf装饰器
# 1.针对FBV
(1).csrf_protect开启csrf校验
代码演示:
from django.views.decorators.csrf import csrf_exempt,csrf_protect
@csrf_protect
def login(request):
return render(request,'login.html')
(2).csrf_exempt忽略csrf校验
代码演示:
from django.views.decorators.csrf import csrf_exempt,csrf_protect
@csrf_exempt
def login(request):
return render(request,'login.html')
# 2.针对CBV
(1).csrf_protect开启csrf校验,针对CBV基于添加装饰器的三种方式都可以生效。
代码演示:
from django import views
from django.utils.decorators import method_decorator
# @method_decorator(csrf_protect,name='post') # 可以生效
class MyViews(views.View):
# @method_decorator(csrf_protect) # 可以生效
def post(self,request):
return HttpResponse('不要大笑,以防乐极生悲')
@method_decorator(csrf_protect) # 可以生效
def dispatch(self, request, *args, **kwargs):
return super(MyViews,self).dispatch(request, *args, **kwargs)
(2).csrf_exempt忽略csrf校验,针对CBV基于添加装饰器的三种方式只有一种可以生效。
代码演示:
from django import views
from django.utils.decorators import method_decorator
# @method_decorator(csrf_exempt,name='post') # 不会生效
class MyViews(views.View):
# @method_decorator(csrf_exempt) # 不会生效
def post(self,request):
return HttpResponse('不要大笑,以防乐极生悲')
@method_decorator(csrf_exempt) # 可以生效
def dispatch(self, request, *args, **kwargs):
return super(MyViews,self).dispatch(request, *args, **kwargs)
基于中间件思想编写项目
# 1.import importlib模块
可以通过字符串的形式导入模块,常规的导入方式是可以直接指定到变量名的,但是importlib模块不行,最小导入单位就
是模块文件。
代码演示:
import importlib
module_path = 'ccc.aaa'
res = importlib.import_module(module_path)
print(res.name)
# 2.发送提示信息简单代码演示
import settings
import importlib
def send_all(msg):
# 循环获取配置文件中字符串信息
for str_path in settings.NOTIFY_FUNC_LIST:
# 切割路径信息
module_path,class_str_name = str_path.rsplit('.',maxsplit=1)
# 根据module_path导入文件
module = importlib.import_module(module_path)
# 利用反射获取模块文件中对应的类名
class_name = getattr(module,class_str_name)
# 实例化
obj = class_name()
# 调用发送信息的功能
obj.send(msg)
auth认证模块
auth认证模块是Django提供给我们快速完成用户相关功能的模块,比如用户的创建、认证等等,关于auth认证模块Django
也配套提供了一张用户表,这张表是执行数据库迁移命令的时候自动生成的auth_user表,Django自带的admin后台管理用户登录参考
的就是这张auth_user表,我们可以创建一个管理员用户,就可以登录到后台,点击上方的:Tools-->run manage.py-->
createsuperuser,就可以创建管理员用户。
# 1.验证用户和密码是否正确
auth.authenticate()
# 2.保存用户登录状态
auth.login()
代码演示:
from django.contrib import auth
def lg(request):
if request.method == 'POST':
username = request.POST.get('username')
password = request.POST.get('password')
is_user_obj = auth.authenticate(request,username=username,password=password) # 先对密码进行
加密,然后进行比对
# print(is_user_obj) # 校验正确返回的是用户对象,错误返回None
if is_user_obj:
# 记录用户登录状态
auth.login(request,is_user_obj) # 自动操作session
return render(request,'lg.html')
# 3.获取当前用户对象
request.user
# 4.判断当前用户是否登录
request.user.is_authenticated()
代码演示:
def get_user(request):
print(request.user) # 已登录返回的是用户对象,没有登录返回AnonymousUser
print(request.user.is_authenticated()) # 已登录返回Ture,没有登录返回False
return HttpResponse('查看用户是否已登录')
# 5.校验登录装饰器
from django.contrib.auth.decorators import login_required
@login_required(login_url='/lg/') # 局部配置
@login_required # 全局配置
LOGIN_URL = '/lg/' # 全局配置需要在配置文件中添加配置
代码演示:
from django.contrib.auth.decorators import login_required
# @login_required(login_url='/func/') # 用户没有登录默认跳转到accounts/login/,也可以加括号自定义跳转页面
# @login_required(login_url='/func/') # 局部配置
@login_required # 全局配置
def index(request):
return HttpResponse('登录之后才能查看的index页面')
# 6.修改密码
request.user.check_password()
request.user.set_password()
request.user.save()
代码演示:
@login_required
def set_pwd(request):
if request.method == 'POST':
old_password = request.POST.get('old_password')
new_password = request.POST.get('new_password')
is_right = request.user.check_password(old_password) # 自动加密在比对
if is_right:
# 修改密码
request.user.set_password(new_password) # 临时修改密码
# 保存数据
request.user.save() # 将修改的密码同步到数据库中
return render(request,'set_pwd.html',locals())
# 7.注销登录
auth.logout(request)
代码演示:
@login_required
def login_out(request):
auth.logout(request)
return HttpResponse('注销成功')
# 8.注册用户
from django.contrib.auth.models import User
User.objects.create_superuser() # 创建管理员
User.objects.create_user() # 创建普通用户
代码演示:
from django.contrib.auth.models import User
def register(request):
# User.objects.create_user(username='jason',password='123') # 创建普通用户
User.objects.create_superuser(username='tom',password='123',email='123@321.com') # 创建管理
员,这三个字段必须填写
return HttpResponse('注册')
auth扩展表字段
扩展auth的表字段有两种方式,第一种是编写一对一表关系,这个稍作了解就行,主要是第二种用类继承来扩展auth的
表字段,需要注意的是类继承之后需要重新执行数据库迁移命令,并且库里面是第一次操作才可以,而且auth模块的所有方法都可
以直接在自定义模型类上面使用,会自动切换参照表。
# 类继承扩展auth表字段
from django.contrib.auth.models import AbstractUser
class MyUser(AbstractUser):
# 编写AbstractUser类中没有的字段,不能冲突
phone = models.BigIntegerField()
AUTH_USER_MODEL = 'app01.Users' # 在配置文件中添加配置,告诉auth模块,不在使用auth_user表,使用自定义的表
这里是IT小白陆禄绯,欢迎各位大佬的指点!!!
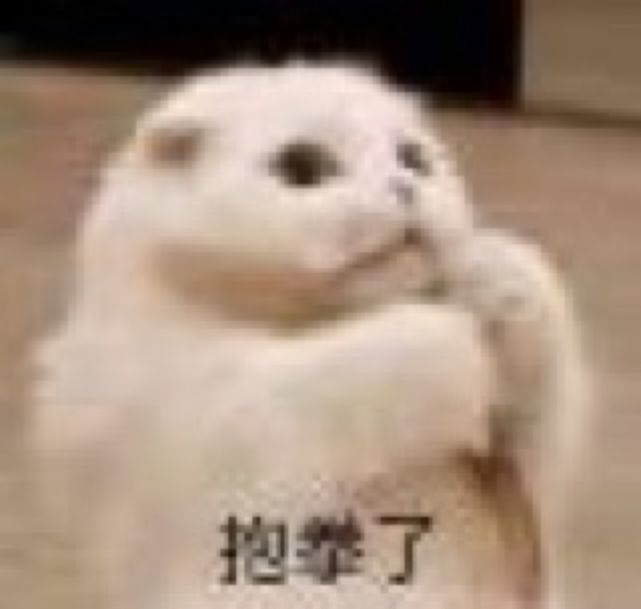