#Given a positive integer n, find the least number of perfect square numbers (for example, 1, 4, 9, 16, ...) which sum to n.
#Example 1:
#Input: n = 12
#Output: 3
#Explanation: 12 = 4 + 4 + 4.
#Example 2:
#Input: n = 13
#Output: 2
#Explanation: 13 = 4 + 9.
#Example 1:
#Input: n = 12
#Output: 3
#Explanation: 12 = 4 + 4 + 4.
#Example 2:
#Input: n = 13
#Output: 2
#Explanation: 13 = 4 + 9.
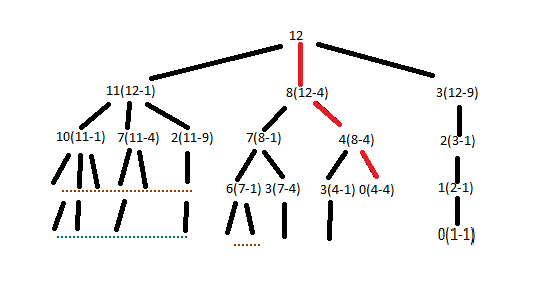
#主要思路: 将n减去所有可能的数字并记录每一个差,并再把每个差减去所有可能的数字,直到差为0。(参考图)
#1.先列出所有可能包含的数字:[1,4,...,m]
#2.写个while 循环:每一次循环count+1,并记录n-m的每一个值,在最后把n-m覆盖原来的n
#3.当n == m,结束,return count
class Solution(object):
def numSquares(self, n):
lst = [u**2 for u in range(1,int(n**0.5)+1) if u**2 <= n] #建立一个包含所有小于n的平方数列
check, count = {n}, 0
while check:
count += 1 #每进行一次循环,+1,
temp = set()
for i in check:
for j in lst:
num = i - j #全部减一次
if num < 0: #如果小于0,说明后面的数再减下去也会小于0,跳过以节省空间
break
if num == 0: #如果等于0, 说明已经找到最短路径,返回count
return count
temp.add(num)
check = temp #替代掉原来的差集
return count
#1.先列出所有可能包含的数字:[1,4,...,m]
#2.写个while 循环:每一次循环count+1,并记录n-m的每一个值,在最后把n-m覆盖原来的n
#3.当n == m,结束,return count
class Solution(object):
def numSquares(self, n):
lst = [u**2 for u in range(1,int(n**0.5)+1) if u**2 <= n] #建立一个包含所有小于n的平方数列
check, count = {n}, 0
while check:
count += 1 #每进行一次循环,+1,
temp = set()
for i in check:
for j in lst:
num = i - j #全部减一次
if num < 0: #如果小于0,说明后面的数再减下去也会小于0,跳过以节省空间
break
if num == 0: #如果等于0, 说明已经找到最短路径,返回count
return count
temp.add(num)
check = temp #替代掉原来的差集
return count