Android入门(九):CheckBox多选清单和ScrollView滚动条
字符串资源文件strings.xml:
<resources>
<string name="hello">主类main</string>
<string name="app_name">选项</string>
<string name="music">音乐</string>
<string name="sing">唱歌</string>
<string name="dance">跳舞</string>
<string name="travel">旅行</string>
<string name="reading">阅读</string>
<string name="writing">写作</string>
<string name="climbing">爬山</string>
<string name="swim">游泳</string>
<string name="exercise">运动</string>
<string name="fitness">健身</string>
<string name="photo">摄影</string>
<string name="eating">美食</string>
<string name="painting">画画</string>
<string name="promptSelOK">确定</string>
<string name="hobList">你的兴趣爱好:</string>
</resources>
界面布局文件main.xml
<?xml version="1.0" encoding="utf-8"?>
<ScrollView xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<LinearLayout
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center_horizontal">
<CheckBox android:id="@+id/chbMusic"
android:layout_width="200dp"
android:layout_height="wrap_content"
android:textSize="30sp"
android:text="@string/music"/>
<CheckBox android:id="@+id/chbSing"
android:layout_width="200dp"
android:layout_height="wrap_content"
android:textSize="30sp"
android:text="@string/sing"/>
<CheckBox android:id="@+id/chbDance"
android:layout_width="200dp"
android:layout_height="wrap_content"
android:textSize="30sp"
android:text="@string/dance"/>
<CheckBox android:id="@+id/chbTravel"
android:layout_width="200dp"
android:layout_height="wrap_content"
android:textSize="30sp"
android:text="@string/travel"/>
<CheckBox android:id="@+id/chbReading"
android:layout_width="200dp"
android:layout_height="wrap_content"
android:textSize="30sp"
android:text="@string/reading"/>
<CheckBox android:id="@+id/chbWriting"
android:layout_width="200dp"
android:layout_height="wrap_content"
android:textSize="30sp"
android:text="@string/writing"/>
<CheckBox android:id="@+id/chbClimbing"
android:layout_width="200dp"
android:layout_height="wrap_content"
android:textSize="30sp"
android:text="@string/climbing"/>
<CheckBox android:id="@+id/chbSwim"
android:layout_width="200dp"
android:layout_height="wrap_content"
android:textSize="30sp"
android:text="@string/swim"/>
<CheckBox android:id="@+id/chbExercise"
android:layout_width="200dp"
android:layout_height="wrap_content"
android:textSize="30sp"
android:text="@string/exercise"/>
<CheckBox android:id="@+id/chbFitness"
android:layout_width="200dp"
android:layout_height="wrap_content"
android:textSize="30sp"
android:text="@string/fitness"/>
<CheckBox android:id="@+id/chbPhoto"
android:layout_width="200dp"
android:layout_height="wrap_content"
android:textSize="30sp"
android:text="@string/photo"/>
<CheckBox android:id="@+id/chbEating"
android:layout_width="200dp"
android:layout_height="wrap_content"
android:textSize="30sp"
android:text="@string/eating"/>
<CheckBox android:id="@+id/chbPainting"
android:layout_width="200dp"
android:layout_height="wrap_content"
android:textSize="30sp"
android:text="@string/painting"/>
<Button android:id="@+id/btnSelOK"
android:layout_width="200dp"
android:layout_height="wrap_content"
android:textSize="30sp"
android:text="@string/promptSelOK"/>
<TextView android:id="@+id/txtHobList"
android:layout_width="300dp"
android:layout_height="wrap_content"
android:textSize="20sp"
android:text="@string/hobList"/>
</LinearLayout>
</ScrollView>
程序代码:
public class MainActivity extends Activity {
private CheckBox chbMusic, chbSing, chbDance, chbTravel, chbReading, chbWriting, chbClimbing,
chbSwim, chbExercise, chbFitness, chbPhoto, chbEating, chbPainting;
private Button btnSelOK;
private TextView txtHobList;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
setupViewComponent();
}
private void setupViewComponent()
{
chbMusic = (CheckBox)findViewById(R.id.chbMusic);
chbSing = (CheckBox)findViewById(R.id.chbSing);
chbDance = (CheckBox)findViewById(R.id.chbDance);
chbTravel = (CheckBox)findViewById(R.id.chbTravel);
chbReading = (CheckBox)findViewById(R.id.chbReading);
chbWriting = (CheckBox)findViewById(R.id.chbWriting);
chbClimbing = (CheckBox)findViewById(R.id.chbClimbing);
chbSwim = (CheckBox)findViewById(R.id.chbSwim);
chbExercise = (CheckBox)findViewById(R.id.chbExercise);
chbFitness = (CheckBox)findViewById(R.id.chbFitness);
chbPhoto = (CheckBox)findViewById(R.id.chbPhoto);
chbEating = (CheckBox)findViewById(R.id.chbEating);
chbPainting = (CheckBox)findViewById(R.id.chbPainting);
btnSelOK = (Button) findViewById(R.id.btnSelOK);
txtHobList = (TextView) findViewById(R.id.txtHobList);
btnSelOK.setOnClickListener(btnSelOKOnClick);
}
private Button.OnClickListener btnSelOKOnClick = new Button.OnClickListener()
{
public void onClick(View v)
{
String s = getString(R.string.hobList);
if(chbMusic.isChecked())
{
s += chbMusic.getText().toString();
}
if(chbSing.isChecked())
{
s += chbSing.getText().toString();
}
if(chbDance.isChecked())
{
s += chbDance.getText().toString();
}
if(chbTravel.isChecked())
{
s += chbTravel.getText().toString();
}
if(chbReading.isChecked())
{
s += chbReading.getText().toString();
}
if(chbWriting.isChecked())
{
s += chbWriting.getText().toString();
}
if(chbClimbing.isChecked())
{
s += chbClimbing.getText().toString();
}
if(chbSwim.isChecked())
{
s += chbSwim.getText().toString();
}
if(chbExercise.isChecked())
{
s += chbExercise.getText().toString();
}
if(chbFitness.isChecked())
{
s += chbFitness.getText().toString();
}
if(chbPhoto.isChecked())
{
s += chbPhoto.getText().toString();
}
if(chbEating.isChecked())
{
s += chbEating.getText().toString();
}
if(chbPainting.isChecked())
{
s += chbPainting.getText().toString();
}
txtHobList.setText(s);
}
};
}
效果图:
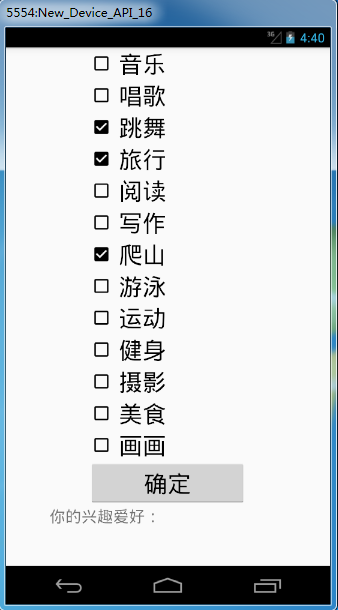