java中pdf文件的管理(pdf文件转png文件,base64传输文件以及删除)
这几天根据需求做了一个小demo,从中学习了一些java中pdf文件的管理和文件转base64,主要包括以下几个方面:
1.前端上传影像文件,把影像文件保存到指定的路径下,然后如果是pdf文件,把pdf文件转换为对应的png文件保存到pdf文件保存地址同级的指定文件夹中,同时保留原pdf文件,如下图:
pdf文件和其对应的png文件所在的文件夹
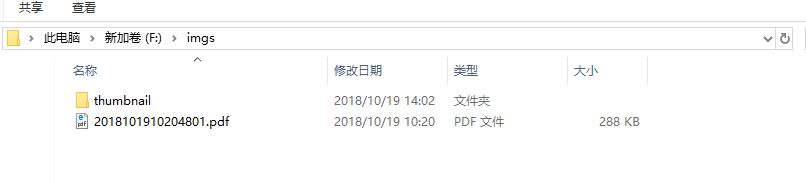
pdf文件对应的png文件(pdf文件和与之对应的png文件名相同,例如:123.pdf对应123.png)
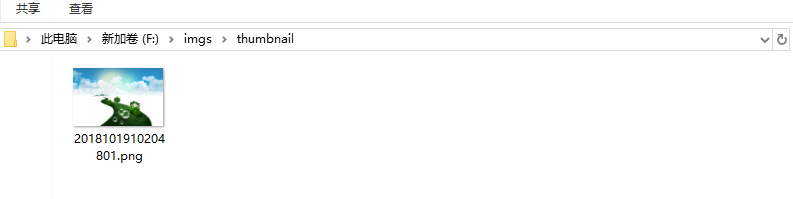
2.影像文件转为base64编码传给前台
3.影像文件的删除,如果是pdf文件,同时把pdf文件和其对应的png文件删除。
引入jar包:
pdf文件转png使用pdfbox.jar,maven地址:使用的是2.0.12版本
<dependency> <groupId>org.apache.pdfbox</groupId> <artifactId>pdfbox</artifactId> <version>2.0.12</version> </dependency>
影像文件转为base64编码使用java自带的BASE64Encoder类
代码部分:
前端上传文件的保存:
@RequestMapping("upLoadImgs") @ResponseBody public String upLoadAgreementsImg(HttpServletResponse response, HttpServletRequest request) throws Exception { //获取前台传输的文件数据 MultipartHttpServletRequest mreq = (MultipartHttpServletRequest) request; JSONObject rt = new JSONObject(); //默认保存成功,失败时再修改对应状态 rt.put("status",0); rt.put("message","文件保存成功"); //上传的合同影像列表 Iterator<String> fileNames = mreq.getFileNames(); MultipartFile file = null; String fileName = null; //文件保存地址,从配置文件中获取 :F:\imgs String folderPath = ResourceBundle.getBundle("systemconfig").getString("imagesDownloadPath"); //自定义影像文件的名字(以当前系统时间+编码区分) String imgName = DateFormatUtils.format(new Date(), "yyyyMMddHHmmss"); //用来区分同次上传多个文件的命名计数器01,02,03 int count = 1; //循环存入前端传来的影像文件 while (fileNames.hasNext()) { String countStr = "0"; fileName = (String) fileNames.next(); file = mreq.getFile(fileName); if (file == null) { logger.info("影像件上传;文件已损坏,请稍后再试"); rt.put("status",1); rt.put("message", "文件已损坏,请稍后再试"); } //获取前台传参文件名 fileName = file.getOriginalFilename(); //创建上传文件目录 File folder = new File(folderPath); if (!folder.exists()) { folder.mkdirs(); } //获取文件的后缀名 String extension = fileName.substring(fileName.lastIndexOf(".")); //保存的文件名 String saveName = imgName + countStr + Integer.toString(count++); String savePath = folderPath + File.separator + saveName + extension; try { file.transferTo(new File(savePath)); } catch (IOException e) { e.printStackTrace(); rt.put("status",1); rt.put("message", "文件保存失败"); } //如果上传的是pdf文件,转换为png文件 if(extension.equals(".pdf")){ //转换为jpg文件 try { pdf2Png(savePath,folderPath,300,1); }catch (IOException e) { e.printStackTrace(); rt.put("status",1); rt.put("message", "pdf缩略图保存失败"); } } logger.info("file path:" + savePath); } return rt.toJSONString(); }
pdf文件转为png文件,并将转换后的png文件保存到pdf同级指定文件夹中:
这里需要注意的是,使用PDDocument 对象转换pdf文件后,使用该对象的close()方法关闭,不然会导致对应的pdf文件时出现因文件被占用而导致无法删除。
/** * 把pdf文件转换为jpg文件同步保存到服务器上 * @param savePath 保存的pdf文件路径 * @param folderPath 文件所在的文件夹 * @param dpi 转换后的文件清晰度, * @param flag 转换的pdf文件页数,为0时转换全部页数,也可以指定转换页数 * @throws Exception */ private void pdf2Png (String savePath,String folderPath,int dpi,int flag) throws Exception { //获取pdf文件 File file = new File(savePath); PDDocument pdDocument; //创建生成的文件保存路径 String newFolderPath = folderPath +File.separator+ "thumbnail"; File folder = new File(newFolderPath); if (!folder.exists()) { folder.mkdirs(); } //转换新的文件名,123.pdf转换为123.png int dot = file.getName().lastIndexOf("."); String imgName = file.getName().substring(0, dot); pdDocument = PDDocument.load(file); PDFRenderer renderer = new PDFRenderer(pdDocument); int pages = pdDocument.getNumberOfPages(); //确定要打印的页数 if (flag > 0) { if (flag < pages) { pages = flag; } } //生成新的文件 StringBuffer imgFilePath = null; for (int i = 0; i < pages; i++) { String imgSavePath = newFolderPath + File.separator + imgName; imgFilePath = new StringBuffer(); imgFilePath.append(imgSavePath); // 因为只转换1页,所以生成的图片只有一张,不用分开命名,如果转换多页pdf文件到多个png图片,须加上命名区分 // imgFilePath.append("-"); // imgFilePath.append(String.valueOf(i + 1)); imgFilePath.append(".png"); File dstFile = new File(imgFilePath.toString()); BufferedImage image = renderer.renderImageWithDPI(i, dpi); //引入一个输出流,方便关闭,不然会因为文件被占用而导致无法删除 OutputStream ops = new FileOutputStream(dstFile); ImageIO.write(image, "png", dstFile); pdDocument.close(); ops.close(); } }
根据文件路径查询该路径下的所有影像文件,组装成json返回给前台,注意的是pdf文件和其对应的png文件组装在同一个JsonObject中,方便前台显示:
/** * * @param imgPath 要查找的文件路径 * @return 返回对应的查询状态值和文件对应的base64编码 * @throws Exception */ public String queryAgreementsImgByProvOid(String imgPath) throws Exception { JSONObject rt = new JSONObject(); //默认状态为查不到数据,查到数据后修改为0 rt.put("status",1); String filePath = ""; //服务器保存地址为null直接返回查询失败 if(!StringUtil.isEmpty(imgPath)) { File file = new File(filePath); //判断该路径是否存在 if (!file.exists()) { rt.put("message", "没有相应的影像件"); } else { File[] files = file.listFiles(); //多个文件放在json数组里 JSONArray imgArray = new JSONArray(); for (int i = 0; i < files.length; i++) { if (files[i].isFile()) { JSONObject imgJson = new JSONObject(); String imgName = files[i].getName(); String imgBASE64Encoder = Base64Util.img2BASE64Encoder(files[i]); //如果是pdf文件,把对应的png、文件也转为base64编码传给前台 if (imgName.endsWith(".pdf")) { //找到对应的png文件的文件地址 StringBuffer imgPngFileName = new StringBuffer(); imgPngFileName.append(filePath + File.separator + "thumbnail"); int dot = imgName.lastIndexOf("."); imgPngFileName.append(File.separator + imgName.substring(0, dot) + ".png"); File pngFile = new File(imgPngFileName.toString()); //如果不存在,返回给前台对应的状态码 if(!pngFile.exists()){ imgJson.put("thumbnail", ""); }else { String pngBASE64Encoder = Base64Util.img2BASE64Encoder(pngFile); imgJson.put("thumbnail", pngBASE64Encoder); } } //组装进json对象里,方便返回给前台 imgJson.put("fileName", imgName); imgJson.put("fileValue", imgBASE64Encoder); imgArray.add(imgJson); } } if (imgArray.size() != 0) { rt.put("status", 0); rt.put("imgs", imgArray); } else { rt.put("message", "没有相应的影像件"); } } }else { rt.put("message", "没有相应的影像件"); } return rt.toJSONString(); }
其中Base64Util.img2BASE64Encoder(pngFile)的代码如下:
/** * 图片转为base64编码,其他文件也是通用的 * @param file 要转换的文件 * @return * @throws Exception */ public static String img2BASE64Encoder(File file) throws Exception{ InputStream in = null; byte[] data = null; try { // 读取图片字节数组 in = new FileInputStream(file); data = new byte[in.available()]; in.read(data); in.close(); } catch (IOException e) { e.printStackTrace(); } BASE64Encoder encoder = new BASE64Encoder(); // 返回Base64编码过的字节数组字符串 return encoder.encode(data); }
根据路径删除该路径下的选中的影像文件,pdf文件同时删除与之对应的png文件:
/** * * @param delFilePath 要删除的文件路径 * @param fileNames 要删除的文件列表 * @return 根据删除状态返回给前台对应的状态值 * @throws Exception */ public String delImgsByPath(String delFilePath, List<String> fileNames) throws Exception { JSONObject rt = new JSONObject(); rt.put("status",0); rt.put("message", "文件删除成功"); if(StringUtil.isEmpty(delFilePath) || fileNames.size()==0){ rt.put("status", 1); rt.put("message", "删除数据出错,请联系相关人员"); } //根据文件路径开始删除 if(!StringUtil.isEmpty(delFilePath)){ String fulllFileName = ""; for (int j = 0; j < fileNames.size(); j++) { String name = fileNames.get(j); //要删除的文件完整路径名 fulllFileName = delFilePath + File.separator + name; File delFile = new File(fulllFileName); if (delFile.exists()) { if (!delFile.delete()) { rt.put("status", 1); rt.put("message", "文件删除失败,请稍后重试"); } } if (name.endsWith(".pdf")) { StringBuffer imgPngFileName = new StringBuffer(); imgPngFileName.append(delFilePath + File.separator + "thumbnail"); int dot = name.lastIndexOf("."); imgPngFileName.append(File.separator + name.substring(0, dot) + ".png"); File pngFile = new File(imgPngFileName.toString()); if(pngFile.exists()){ if (!pngFile.delete()) { rt.put("status", 1); rt.put("message", "文件删除失败,请稍后重试"); } } } } } return rt.toJSONString(); }
以上就是对影像文件进行增,删,查的功能的代码,其中pdf文件由于文件类型特殊,前段无法显示pdf文件的缩略图进行选中删除操作,所以把pdf文件转换为png文件后再保存到指定的文件夹中,查询的时候一起组装为一个json对象里传给前台,方便前台的显示,删除时同时把pdf文件对应的png文件删除,防止占用内存空间。关于pdf文件在前段无法生成缩略图这个问题的解决方案,还在持续的学习中,如果有好的建议,欢迎留言大家一起学习。