public class MyList {
static class Node {
Object data;
Node next;
public Node(Object data) {
this.data = data;
this.next = null;
}
}
Node head;
public MyList() {
head = null;
}
public void clear() {
head = null;
}
public void bianli()// 遍历
{
Node p = head;
while (p != null) {
System.out.print(p.data + " ");
p = p.next;
}
System.out.println();
}
public boolean isEmpty()// 推断是否为空
{
return head == null;
}
public int size() {
Node p = head;
int sum = 0;
while (p != null) {
sum++;
p = p.next;
}
return sum;
}
public void insert(Object d, int pos) {
if (pos < 0 || pos > size()) {
throw new RuntimeException("下标错误");
}
Node newNode = new Node(d);
if (pos == 0) {
newNode.next = head;
head = newNode;
} else if (pos >= size() - 1) {
get(size() - 1).next = newNode;
} else {
newNode.next = get(pos);
get(pos - 1).next = newNode;
}
}
public Node get(int pos) {
if (pos < 0 || pos > size()) {
throw new RuntimeException("下标错误");
}
if (pos == 0)
return head;
Node p = head;
for (int i = 0; i < pos; i++)
p = p.next;
return p;
}
public static void main(String[] args) {
MyList list = new MyList();
list.insert(10, 0);
list.insert(20, 1);
list.insert(30, 0);
list.insert(40, 1);
System.out.println(list.size());
list.bianli();
System.out.println(list.isEmpty());
System.out.println(list.get(2).data);
list.clear();
System.out.println(list.isEmpty());
}
}
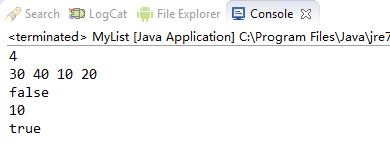