UICollectionView
//
// CollectionController.h
// MGuardian
//
// Created by krmao on 16/5/13.
// Copyright © 2016年 krmao. All rights reserved.
//
#import <UIKit/UIKit.h>
@interface CollectionController : UIViewController
@end
//********************************************************************************
//*自定义类 UICollectionViewCell START
//********************************************************************************
//静态变量声明定义
static NSString * __nonnull collectionCellID=@"mmcell";//可复用的reuseIdentifier
@interface MCollectionItemCellView : UICollectionViewCell
@property (weak, nonatomic) IBOutlet UILabel *mLabelView;
+ (MCollectionItemCellView * __nonnull)getCellView: (UICollectionView * __nonnull) uiCollectionView index:(NSIndexPath * __nonnull)indexPath;
@end
//********************************************************************************
//*自定义类 UICollectionViewCell 实现
//********************************************************************************
@implementation MCollectionItemCellView
- (id __nonnull)initWithFrame:(CGRect)frame{
self=[super initWithFrame:frame ];
return self;
}
//静态工厂方法
+ (MCollectionItemCellView * __nonnull)getCellView: (UICollectionView * __nonnull) uiCollectionView index:(NSIndexPath * __nonnull)indexPath;{
MCollectionItemCellView * cellView =(MCollectionItemCellView *) [uiCollectionView dequeueReusableCellWithReuseIdentifier:collectionCellID forIndexPath:indexPath];
//设置cell选中状态
//cellView.selectionStyle=UITableViewCellSelectionStyleDefault;
cellView.selectedBackgroundView=[[UIView alloc]initWithFrame:cellView.frame];
cellView.selectedBackgroundView.backgroundColor=[UIColor greenColor];
[cellView sizeToFit];
return cellView;
}
@end
//********************************************************************************
//*自定义类 UICollectionViewCell END
//********************************************************************************
//
// CollectionController.m
// MGuardian
//
// Created by krmao on 16/5/13.
// Copyright © 2016年 krmao. All rights reserved.
//
#import "CollectionController.h"
@interface CollectionController ()<UICollectionViewDataSource,UICollectionViewDelegate>
@property (weak, nonatomic) IBOutlet UICollectionView *mCollectionView;
@property NSMutableArray *dataList;
@end
@implementation CollectionController
- (void)viewDidLoad {
[super viewDidLoad];
self.mCollectionView.dataSource=self;
self.mCollectionView.delegate=self;
self.dataList=[[NSMutableArray alloc]initWithObjects: @"a",@"b",@"c",@"d",@"e",@"f",@"g",@"h",@"i",@"j",@"k",@"l",@"m",
@"n",@"o",@"p",@"q",@"r",@"s",@"t",@"u",@"v",@"w",@"x",@"y",@"z", nil];
}
- (NSInteger)collectionView:(UICollectionView *)collectionView numberOfItemsInSection:(NSInteger)section{
return self.dataList.count;
}
- (UICollectionViewCell *)collectionView:(UICollectionView *)collectionView cellForItemAtIndexPath:(NSIndexPath *)indexPath{
MCollectionItemCellView *celView=[MCollectionItemCellView getCellView:collectionView index:indexPath];
celView.mLabelView.text=[NSString stringWithFormat: @"item:%i-%@",indexPath.row,[self.dataList objectAtIndex:indexPath.row]];
return celView;
}
@end
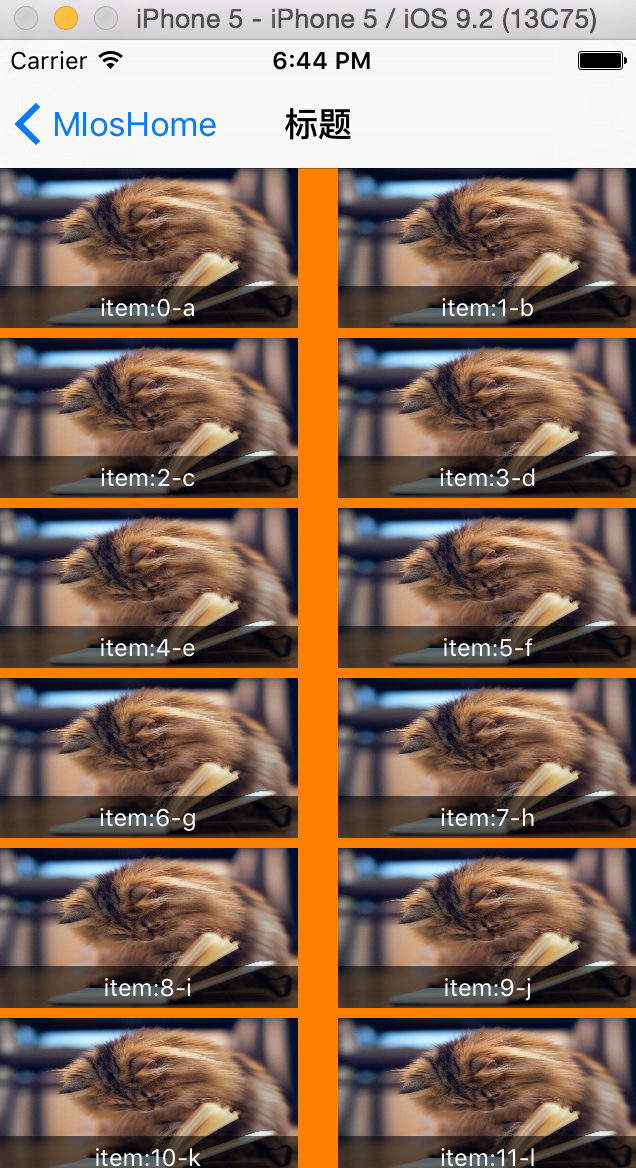
让过去成就未来.