day 81 天 ORM 操作复习总结
# ###############基于对象查询(子查询)##############
一、对多查询
正向查询
from django.shortcuts import render,HttpResponse from app01 import models # Create your views here. def query(request): # ###############基于对象查询(子查询)############## # 一对多查询 book-->publish #正向查询: # 查询python这本书的出版社的email邮箱. python_obj = models.Book.objects.filter(title ='python').first() print(python_obj.publish.email) return HttpResponse(' OK')
# ###############基于对象查询(子查询)##############
# 正向查询按字段查询
# 一对多查询 book----------------------->publish
# <----------------------
#反向查询 book_set.all()
反向查询
反向查询#查询五道口出版社的书籍名称 pub_obj =models.Publish.objects.filter(name ='五道口出版社').last()
for obj in pub_obj.book_set.all():
print(obj.title)
return HttpResponse(' OK')
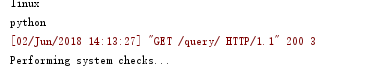
二、多对多 查询
# 按字段查询 正向查询(author.all())
# 多对多 book----------------------> author
# <----------------------
# book_set.all()
正向多对多查询
#查询python作者的年龄
python_obj =models.Book.objects.filter(title ='python').first() print(python_obj) for author in python_obj.authors.all(): print(author.name,author.age) return HttpResponse('ok')
输出结果
反向多对多
#查询 alex出版过的书籍 alex =models.Author.objects.filter(name ='哪吒').first() for book in alex.book_set.all(): print(book.title) return HttpResponse('ok')
三 、一对一的关系
author 于authordetail表的关系 。
一对一正向查询
反向查询
查询家在山东的作者名:
###############基于queryset和__查询(join的查询)####################
四 、
正向查询 : 按字段
反向查询:按表名
案例1 .
#查询python这本书籍的出版社的邮箱
python_obj = models.Book.objects.filter(title = 'python' ).first() print(python_obj.publish.email) return HttpResponse( ' OK' ) |
案例2.
案例3
两种方法
输出结果:
VIEWS文件 :
from django.shortcuts import render,HttpResponse from app01 import models # Create your views here. def query(request): # ###############基于对象查询(子查询)############## # 正向查询按字段查询 # 一对多查询 book----------------------->publish # <---------------------- #反向查询 book_set.all() # #正向查询: # # # 查询python这本书的出版社的email邮箱. # # python_obj = models.Book.objects.filter(title ='python').first() # print(python_obj.publish.email) #f反向查询 按表明小写_set.all() #查询五道口出版社的书籍名称 # pub_obj =models.Publish.objects.filter(name ='五道口出版社').last() # for obj in pub_obj.book_set.all(): # print(obj.title) # 按字段查询 正向查询(author.all()) # 多对多 book----------------------> author # <---------------------- # book_set.all() #查询python作者的年龄 # python_obj =models.Book.objects.filter(title ='python').first() # print(python_obj) # for author in python_obj.authors.all(): # print(author.name,author.age) #查询 alex出版过的书籍 # alex =models.Author.objects.filter(name ='哪吒').first() # for book in alex.book_set.all(): # print(book.title) # # 按字段查询 正向查询(authordetail.all()) # 多对多 author----------------------> authordetail # <---------------------- # 按表名 author #查询 哪吒的电话号码 nezhao_obj = models.Author.objects.filter(name ='哪吒').first() print(nezhao_obj.authorDetail.telephone) # 反向查询 # 查询家在山东的作者名字 add_obj = models.AuthorDetail.objects.filter(addr ="山东").first() print(add_obj.author.name) #############################基于queryset和__查询(join的查询)######################### #正向查询:按字段 #反向查询 :表名小写 #查询python这本书籍的出版社的邮箱 ret =models.Book.objects.filter(title ='python').values('publish__email') print(ret ) #查询清华出版社的书籍名称 #方法1 qinghua_obj =models.Publish.objects.filter(name ='清华出版社').values('book__title') print(qinghua_obj) #方法2 qinghua_obj= models.Book.objects.filter(publish__name='清华出版社').values('title') print(qinghua_obj) # 查询哪吒的手机号 ret = models.Author.objects.filter(name ='哪吒').values('authorDetail__telephone') print(ret) return HttpResponse('ok') #查询手机号 以 186开头的作者的出版过的书籍名称以及对应的出版社
2018.10.29日整理
from django.db import models # Create your models here. class Author(models.Model): nid = models.AutoField(primary_key=True) name=models.CharField( max_length=32) age=models.IntegerField() # 与AuthorDetail建立一对一的关系 authorDetail=models.OneToOneField(to="AuthorDetail",on_delete=models.CASCADE) def __str__(self): return self.name class AuthorDetail(models.Model): nid = models.AutoField(primary_key=True) birthday=models.DateField() telephone=models.BigIntegerField() addr=models.CharField( max_length=64) def __str__(self): return self.addr class Publish(models.Model): nid = models.AutoField(primary_key=True) name=models.CharField( max_length=32) city=models.CharField( max_length=32) email=models.EmailField() def __str__(self): return self.name class Book(models.Model): nid = models.AutoField(primary_key=True) title = models.CharField( max_length=32) publishDate=models.DateField() price=models.DecimalField(max_digits=5,decimal_places=2) # 与Publish建立一对多的关系,外键字段建立在多的一方 publish=models.ForeignKey(to="Publish",to_field="nid",on_delete=models.CASCADE) # 与Author表建立多对多的关系,ManyToManyField可以建在两个模型中的任意一个,自动创建第三张表 authors=models.ManyToManyField(to='Author',) def __str__(self): return self.title
orm查询
from django.shortcuts import render,HttpResponse # Create your views here. from .models import * def index(request): #查询沙河出版社所有书籍的名称和价格(一对多) ret = Publish.objects.filter(name="沙河出版社").values("book__title","book__price") #正向查询按字段,反向查询按表名. print(ret) #打印结果:<QuerySet [{'book__title': 'python入门', 'book__price': Decimal('100.00')}]> """ select book.title,book.price from Publish inner join book on Publish.pk =Book.pulish_id where publish.name ="沙河出版社" """
ret=Book.objects.filter(publish__name="沙河出版社").values("title","price") print(ret) #打印结果:<QuerySet [{'title': 'python入门', 'price': Decimal('100.00')}]> return HttpResponse("ok ")