JSON解析遇到的字段名转换问题
一、问题背景
在使用JSON
对Object
进行解析后得到的是一个K-V
键值对,但是原始的Object
字段名可能为驼峰命名或下划线或帕斯卡等各种各样的方式,那么在进行JSON
解析之后得到的\(key\)名字就会不同。如何解决这个问题?现考虑代码中使用了fastjson
或jackson
的解析方法进行转换。
二、测试
1、实体测试类:
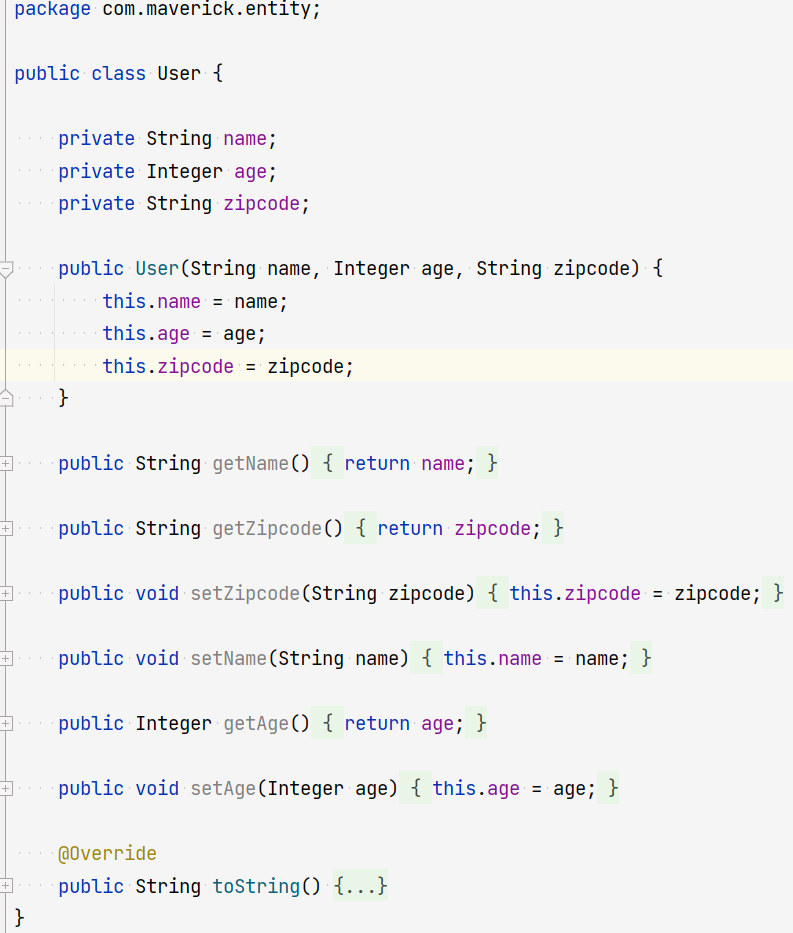
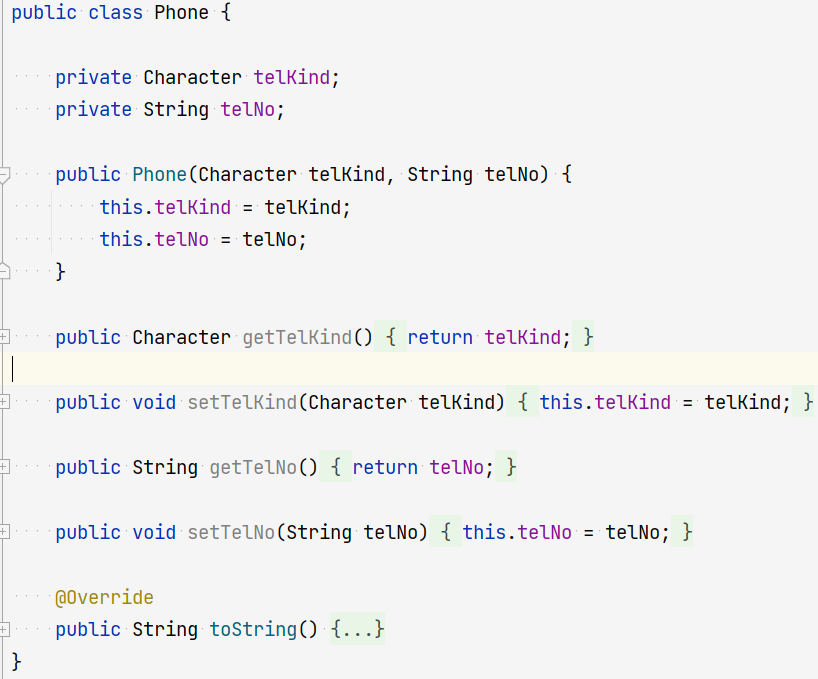
再搞个复杂点的DTO类:
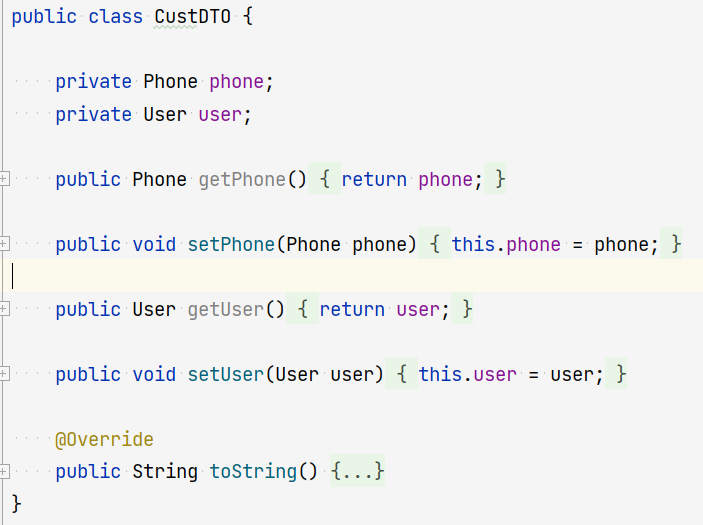
2、封装fastjson
和jackson
两个工具类:
package com.maverick.util;
import com.alibaba.fastjson.JSON;
import com.alibaba.fastjson.PropertyNamingStrategy;
import com.alibaba.fastjson.serializer.SerializeConfig;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.ObjectMapper;
public class JsonUtils {
private final static SerializeConfig FAST_JSON_CONFIG = new SerializeConfig();
static {
FAST_JSON_CONFIG.propertyNamingStrategy = PropertyNamingStrategy.SnakeCase;
}
private final static ObjectMapper OBJECT_MAPPER = new ObjectMapper();
static {
OBJECT_MAPPER.setPropertyNamingStrategy(com.fasterxml.jackson.databind.PropertyNamingStrategy.SNAKE_CASE);
}
private JsonUtils() {
}
/**
* 使用fastjson
*
* @param object
* @return
*/
public static String getFromFastJson(Object object) {
try {
return JSON.toJSONString(object, FAST_JSON_CONFIG);
} catch (Exception e) {
throw new RuntimeException(e.toString());
}
}
/**
* 使用jackson
*
* @param object
* @return
*/
public static String getFromJackson(Object object) {
try {
return OBJECT_MAPPER.writeValueAsString(object);
} catch (JsonProcessingException e) {
throw new RuntimeException(e.toString());
}
}
}
3、测试程序:
public class Demo {
public static void main(String[] args) {
User user = new User("张三", 20, "101101");
Phone phone = new Phone('1', "1234455767");
List<CustDTO> list = new ArrayList<>();
CustDTO custDTO = new CustDTO();
custDTO.setUser(new User("李四", 30, "101101"));
custDTO.setPhone(new Phone('0', "057105710571"));
list.add(custDTO);
Map<String, Object> map = new HashMap<>();
map.put("user", user);
map.put("phone", phone);
map.put("custdto", custDTO);
// 实际项目中不要使用这种方式, 尽量使用单例模式
// SerializeConfig config = new SerializeConfig();
// config.propertyNamingStrategy = PropertyNamingStrategy.SnakeCase;
String json_1 = JsonUtils.getFromFastJson(map);
System.out.println(json_1);
String json_2 = JsonUtils.getFromJackson(map);
System.out.println(json_2);
System.out.println(json_1.equals(json_2));
}
}
测试结果如下,可以看到解析后驼峰字段都转为了下划线的方式(如tel_kind
、tel_no
)
fastjson: {"phone":{"tel_kind":"1","tel_no":"1234455767"},"custdto":{"phone":{"tel_kind":"0","tel_no":"057105710571"},"user":{"age":30,"name":"李四","zipcode":"101101"}},"user":{"age":20,"name":"张三","zipcode":"101101"}}
jackson: {"phone":{"tel_kind":"1","tel_no":"1234455767"},"custdto":{"phone":{"tel_kind":"0","tel_no":"057105710571"},"user":{"name":"李四","age":30,"zipcode":"101101"}},"user":{"name":"张三","age":20,"zipcode":"101101"}}