使用Microsoft Graph API整合Office 365
在开始写代码之前我们需要在Azure管理中心中新建应用程序,并且获取下面三个关键的字符串
- 应用程序ID
- 密钥
- 租户ID
这里可以参考文章
- 《如何在Azure的管理中心中注册应用程序并且分配Graph API权限》获取“应用程序ID”和“密钥”
- 《如何获取Azure 租户ID》获取租户ID
*注意:由于该模式使用Azure AD中对应用的授权,所以Azure对应用无条件信任,所以千万不要泄露 应用程序ID、密钥和租户ID
Web应用整合Office 365,使得Web应用可以通过使用Microsoft Graph API获取或管理Office 365上的资源,Microsoft Graph开发堆栈如下:
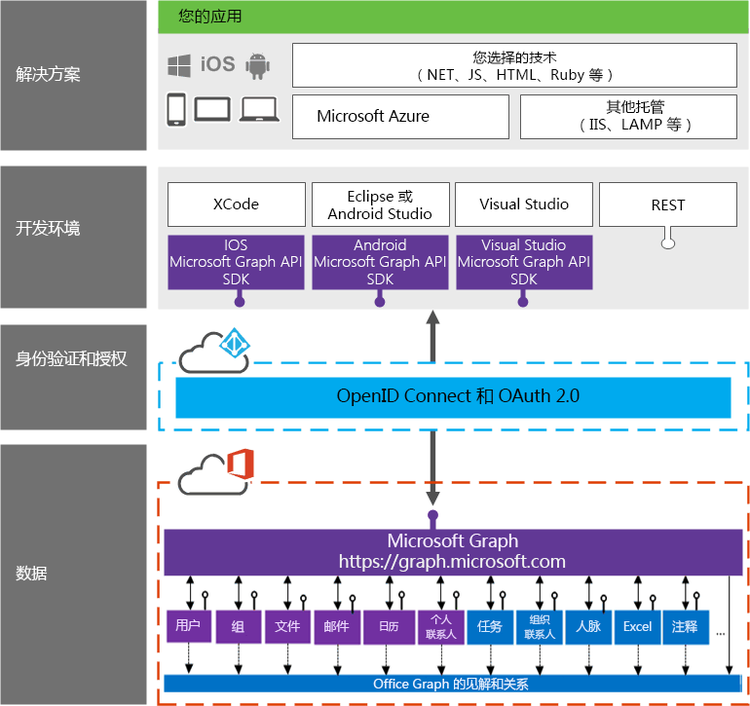
根据上图我们需要拿到Graph API的客户端,这里微软为我们提供了相对应的操作类库可以直接使用
相关类库有以下几个,可以在Visual Studio的Nuget管理器中找到:
- Microsoft.Graph
- Microsoft.Graph.Core
- Active Directory Authentication Library
然后在系统中创建一个 IAuthenticationProvider接口的的实现,我的实现类如下:
public class AzureAuthenticationProvider : IAuthenticationProvider { private string _tenantID; /// <summary> /// 租户ID /// </summary> public string TenantID { get { return _tenantID; } } private string _applicationId; /// <summary> /// 应用ID /// </summary> public string ApplicationId { get { return _applicationId; } } private string _appPassword; /// <summary> /// 应用密码 /// </summary> public string AppPassword { get { return _appPassword; } } public AzureAuthenticationProvider(string tenantID, string applicationId, string appPassword) { _tenantID = tenantID; _applicationId = applicationId; _appPassword = appPassword; } public async Task AuthenticateRequestAsync(System.Net.Http.HttpRequestMessage request) { var authority = string.Format("https://login.chinacloudapi.cn/{0}/",TenantID); AuthenticationContext authenticationContext = new AuthenticationContext(authority); ClientCredential clientCred = new ClientCredential(ApplicationId, AppPassword); try { AuthenticationResult authenticationResult = await authenticationContext.AcquireTokenAsync("https://microsoftgraph.chinacloudapi.cn/", clientCred); request.Headers.Add("Authorization", "Bearer " + authenticationResult.AccessToken); } catch (AdalException ex) { if (ex.ErrorCode == AdalError.FailedToAcquireTokenSilently) { authenticationContext.TokenCache.Clear(); } } } }
然后使用相对应版本的 Graph API地址创建 GraphServiceClient
国内版的地址为:https://microsoftgraph.chinacloudapi.cn/v1.0
国际版的地址为:https://graph.microsoft.com/v1.0
var graphClient = new GraphServiceClient("https://microsoftgraph.chinacloudapi.cn/v1.0",new AzureAuthenticationProvider(TenantID, ApplicationId, AppPassword))
至此就得到了GraphClient对象了,我们可以利用此对象获取配置中允许访问的内容(如邮件、通讯录、个人信息等),例如下面代码可以获取指定用户的未读邮件数量。
var user = "user1@modtsp.partner.onmschina.cn"; var result = graphClient.Users[user].MailFolders.Inbox.Request().GetAsync().Result; var unreadCount = result.UnreadItemCount ?? 0;
相关资源