SpringBoot - 整合Mybatis-Plus
前言
Mybatis-Plus
是Mybatis
的增强,Mybatis-Plus
在Mybatis
的基础上借鉴了很多JPA
的做法,记录下SpringBoot
下的整合方法。
环境
SpringBoot2.53 + Mybatis-Plus3.3.0
具体实现
项目结构
项目配置
pom.xml
<!-- web -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- mysql -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<scope>runtime</scope>
</dependency>
<!-- mybatis-plus -->
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.3.0</version>
</dependency>
<!-- lombok -->
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<scope>provided</scope>
</dependency>
application.yml
spring:
application:
name: mybatis-learn
# 文件编码 UTF8
mandatory-file-encoding: UTF-8
# 数据源配置,请修改为你项目的实际配置
datasource:
driver-class-name: com.mysql.cj.jdbc.Driver
url: jdbc:mysql://localhost:3306/cms?useSSL=false&serverTimezone=UTC&characterEncoding=UTF8
username: root
password: sunday
# mybatis-plus配置
mybatis-plus:
configuration:
# 开启下划线转驼峰
map-underscore-to-camel-case: true
# mapper路径位置
mapper-locations: classpath:mapper/*.xml
server:
port: 8084
Table
CREATE TABLE `product` (
`id` int(11) unsigned NOT NULL AUTO_INCREMENT,
`title` varchar(100) COLLATE utf8_unicode_ci DEFAULT NULL,
`create_time` datetime DEFAULT CURRENT_TIMESTAMP,
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=3 DEFAULT CHARSET=utf8 COLLATE=utf8_unicode_ci
实现代码
- 启动类
import org.mybatis.spring.annotation.MapperScan;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
@MapperScan(basePackages = "com.coisini.mybatisplus.mapper") // mapper扫描位置
public class MybatisplusApplication {
public static void main(String[] args) {
SpringApplication.run(MybatisplusApplication.class, args);
}
}
ProductController.java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import java.util.List;
@RequestMapping("/product")
@RestController
public class ProductController {
@Autowired
private ProductService productService;
/**
* 查询Products
* @return
*/
@GetMapping("/select")
public List<Product> selectProducts() {
return productService.getProducts();
}
}
ProductService.java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
@Service
public class ProductService {
@Autowired
private ProductMapper productMapper;
/**
* 查询products
* @return
*/
public List<Product> getProducts() {
return productMapper.selectList(null);
}
}
ProductMapper.java
import com.baomidou.mybatisplus.core.mapper.BaseMapper;
import org.springframework.stereotype.Repository;
@Repository
public interface ProductMapper extends BaseMapper<Product> {
// 继承 BaseMapper,与JPA类似
}
Product.java
import com.baomidou.mybatisplus.annotation.TableName;
import lombok.Getter;
import lombok.Setter;
import java.util.Date;
@Getter
@Setter
@TableName("product")
public class Product {
private Integer id;
private String title;
private Date createTime;
}
测试
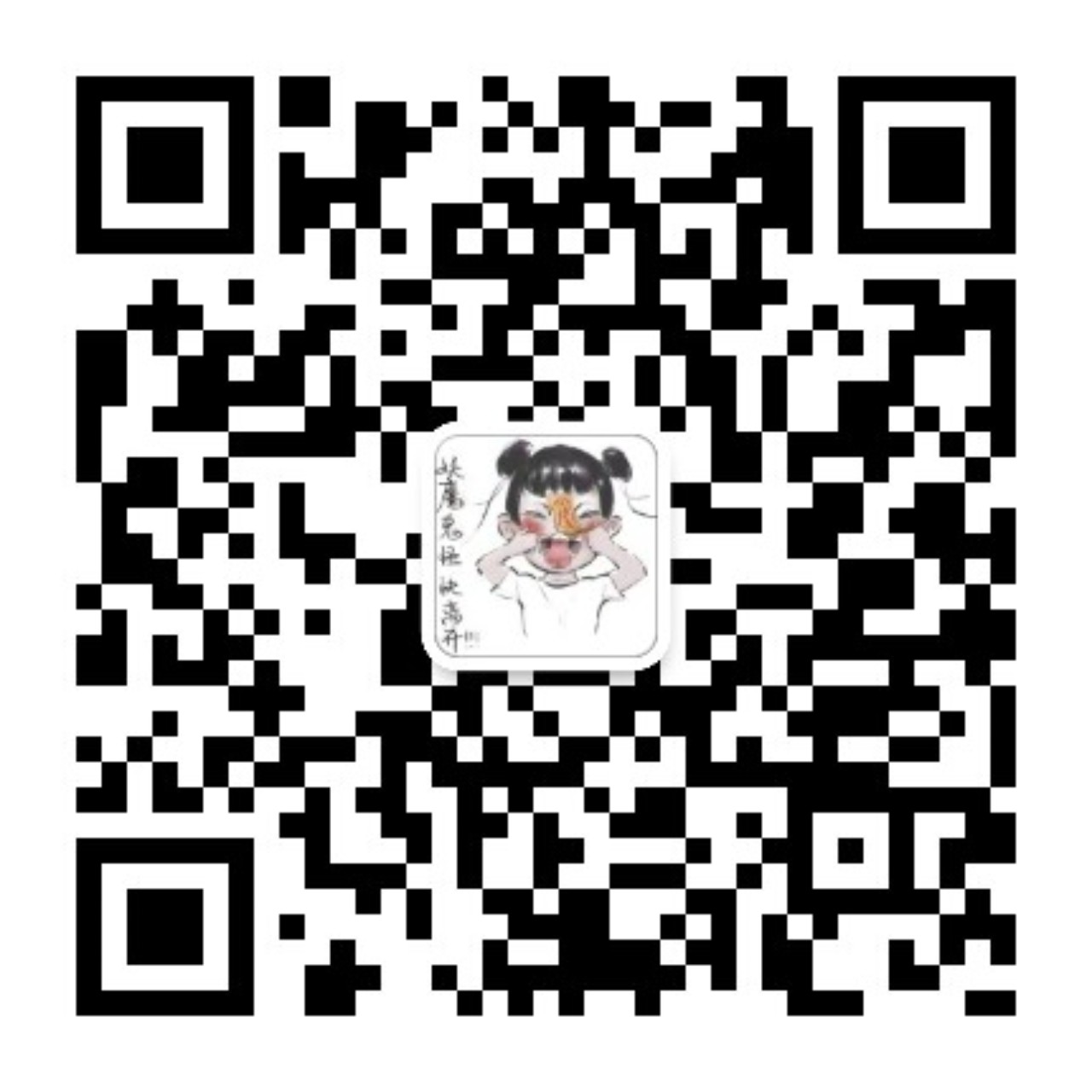