待办事项
效果图
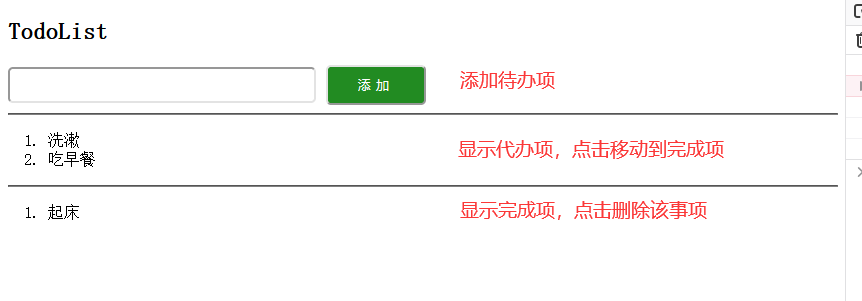
目录结构
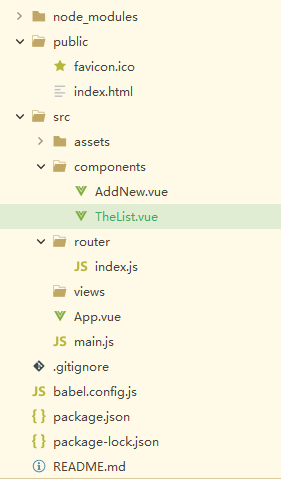
详细代码
AddNew.vue
<template>
<div>
<input v-model="content"/>
<button @click="addList()">添加</button>
</div>
</template>
<script>
export default {
name: "AddNew",
data() {
return {
content: ''
}
},
methods: {
addList() {
if(!this.content) {
alert("请输入内容");
return;
}
//调用App.vue文件中的add方法
this.$emit("addFunc",{content: this.content});
this.content = ''
}
}
}
</script>
<style>
input {
width: 300px;
height: 30px;
border-radius: 5px;
}
button {
width: 100px;
height: 40px;
border-radius: 5px;
background: forestgreen;
color: #fff;
margin-left: 10px;
letter-spacing: 5px;
}
</style>
TheList.vue
<template>
<ol >
<li v-for="(item, index) in list" :key="index" @click="selectDel(index)">{{item.content}}</li>
</ol>
</template>
<script>
export default {
name:"TheList",
//props属性除了可以是一个数组外,还可以是一个对象
/*props: [
'list',
'flag'
],*/
props: {
list: {
type: Array,
required: true,
},
flag: {
type: Boolean,
default: true
}
},
methods: {
selectDel(index) {
if(this.flag) {
this.$emit('toDelFunc',index);
}else {
this.$emit('doDelFunc',index);
}
}
}
}
</script>
<style>
</style>
index.js
import Vue from 'vue'
import VueRouter from 'vue-router'
Vue.use(VueRouter)
const routes = [
]
const router = new VueRouter({
routes
})
export default router
App.vue
<template>
<div>
<h2>TodoList</h2>
<AddNew @addFunc="add"></AddNew>
<hr />
<TheList :list="toList" @toDelFunc="toDelList"></TheList>
<hr />
<TheList :list="delList" @doDelFunc="doDelList" :flag="false"></TheList>
</div>
</template>
<script>
//引入组件对象
import AddNew from './components/AddNew.vue';
import TheList from './components/TheList.vue';
export default {
data() {
return {
toList: [],
delList: []
}
},
methods: {
add(val) {
console.log(val)
this.toList.push(val);
},
toDelList(index) {
this.delList.push(this.toList.splice(index, 1)[0]);
},
doDelList(index) {
this.delList.splice(index, 1);
}
},
components: {
AddNew,
TheList
}
}
</script>
<style>
</style>
main.js
import Vue from 'vue'
import App from './App.vue'
import router from './router'
Vue.config.productionTip = false
new Vue({
router,
render: h => h(App)
}).$mount('#app')